How to Use Angular console.log Function
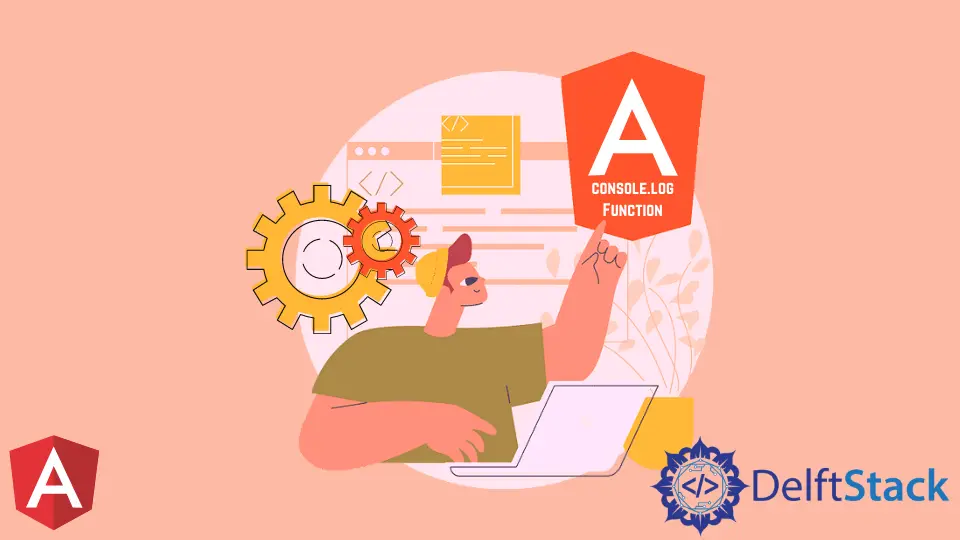
This article will discuss the complete concept of the Angular console.log
.
the console.log
Function in Angular
console.log
is a JavaScript function that logs messages to the developer’s console or the browser console to the web page.
This method helps Angular developers by informing them about the errors, warnings, and what is happening during the execution of their code.
It is used to debug an Angular application, identify performance issues, and troubleshoot problems with third-party libraries or other dependencies.
We can access this function by opening the developer tools on any browser and navigating to the Console tab. We can also find it in Android Studio at View > Tool Windows > Other > Console
.
console.log
messages are always written with a timestamp, a level, and a message inside parenthesis. The message will depend on the type of log.
The level can be one of the following.
Debug
: This is for debugging purposes and should be avoided if possible.Error
: A runtime error has occurred and will stop the execution of your code until you fix it.Info
: This is for informational purposes and will not stop the execution of your code.
Before writing a code using console.log
, we must first create a new Angular project by typing the following commands in the terminal.
ng new my-project
cd my-project
ng serve
Next, we open our browser and go to http://localhost:4200/
, where we’ll see a blank screen.
Now, let’s write some code using console.log
in Angular.
TypeScript code:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent{
console = console;
}
HTML code:
<h2>Example of Angular Console log</h2>
<p>
press F12 and then run the code
</p>
{{ console.error('It will show up an error') }}
{{ console.log('It will show up logging') }}
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook