CLI - Build for Production on Angular
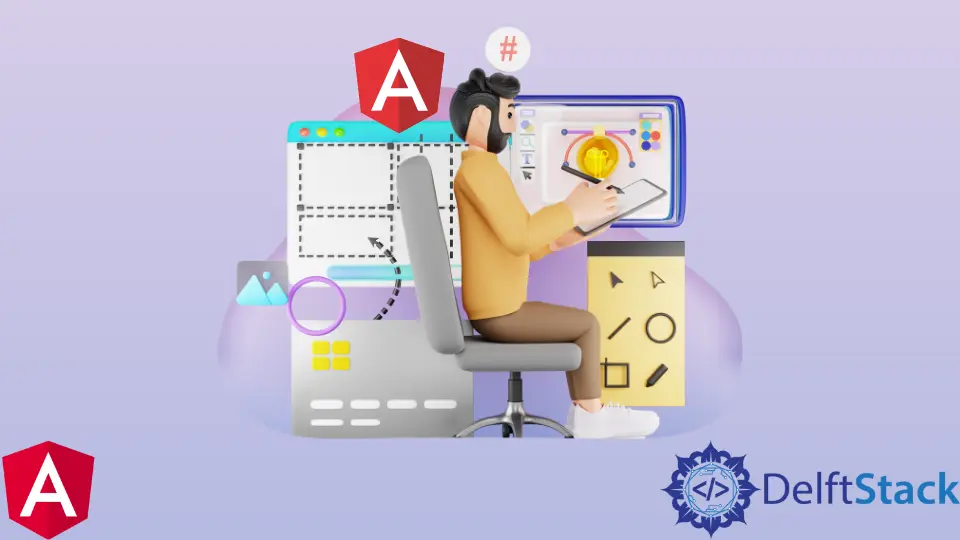
After a web application is made and ready for deployment, we require that Angular compile all the different code snippets into one build. The build is compressed into a smaller size.
During this final step, we are bound to encounter some minor issues. It can be such a frustrating experience, considering the rigors one has gone through to build the app.
It is easy to think this is an isolated problem but even new projects that have not been tampered with go through the same problems. One major problem that has been encountered is where main.js
is not created; then there will be other warnings like:
- Dropping unused function.
- Site effects in initialization.
How to Solve CLI - Build Problems
Before we dive into the different working solutions, let us look at different flag settings for Angular:
angularCopy# Prod these are equivalent
ng build --target=production --environment=prod
ng build --prod --env=prod
ng build --prod
# Dev and so are these
ng build --target=development --environment=dev
ng build --dev --env=dev
ng build --dev
ng build
When --prod
is used in Angular, it sets up some non-flaggable settings:
- Adds service worker if configured in
.angular-cli.json
. - Replaces
process.env.NODE_ENV
in modules with theproduction
value (this is needed for some libraries, like react). - Runs UglifyJS on the code.
When a project is being built, commands such as ng build --env=prod
don’t accomplish a great build production job as they don’t compress the files. Another thing to remember is to confirm that AOT is implemented. AOT is mainly active by default.
The ng build --prod
command should work if Ahead of Time (AOT) is implemented. Use the ng build --prod --aot=false
command if it doesn’t work.
The ng serve --prod
command can also work if the enable production function is enabled in Angular. This command also helps speed up the app load time significantly.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn