How to Check Angular Version
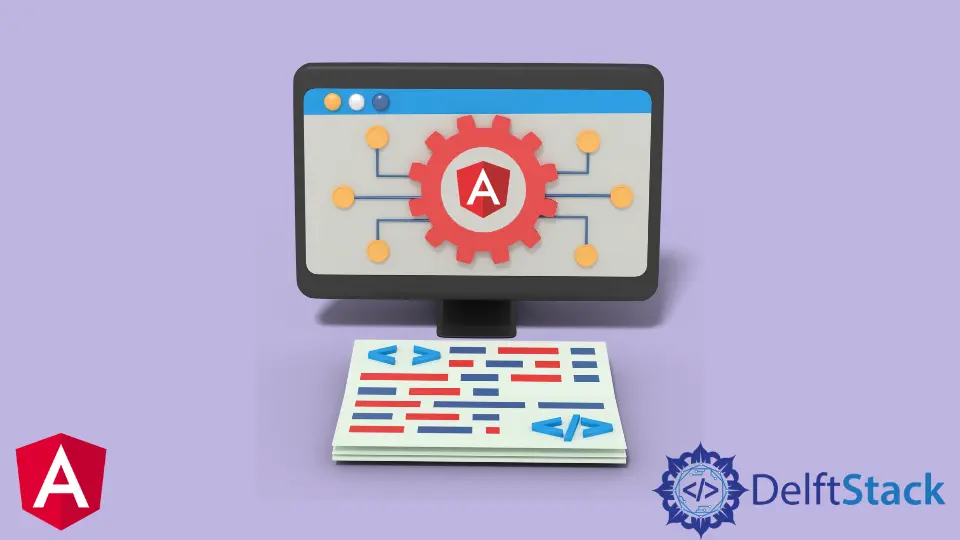
We will introduce different ways to check the angular version
.
Check the Angular Version with CLI
Once you have installed an application in Angular, you may want to know which version of Angular is installed on your application. Or if you recently started to work on your previous app and forget which Angular version you were using for that app, then there are different ways to check the Angular version.
One of them is checking the Angular version using Angular CLI. All you have to do is go to the terminal inside your project and run the following command.
# angular CLI
ng --version
Output:
Check Angular Version Using the package.json
Another way to check your app’s Angular version is by finding it from the package.json
file.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn