How to Use $broadcast() in AngularJS
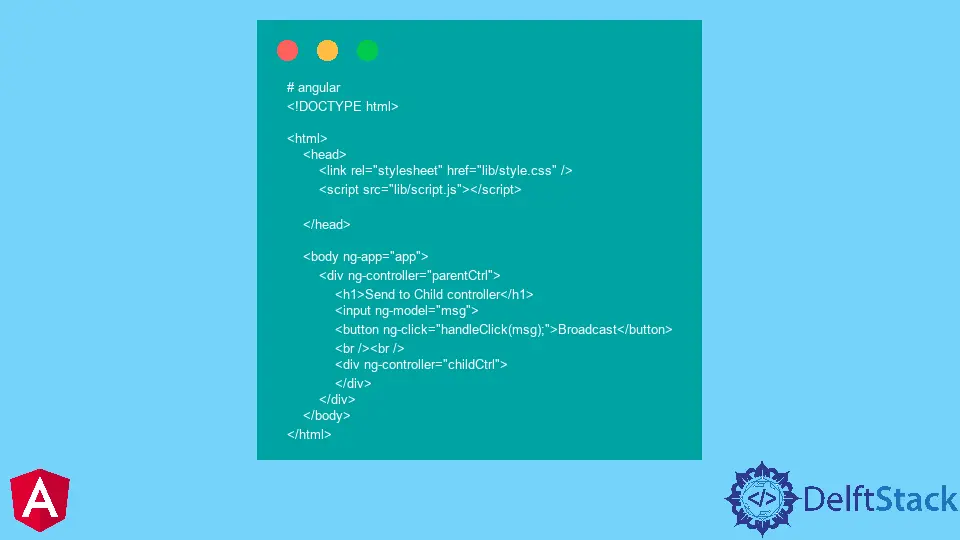
This article will introduce $broadcast()
in AngularJS.
Use $broadcast()
in AngularJS
$broadcast()
is a $rootScope
method to send events from parent controllers to child controllers. It sends an event that the listener of that particular scope can catch.
Once the event is sent from the parent controller to a child controller, the child controller can handle the event using another method, $scope.$on
.
Let’s go through an example in which we will use $broadcast()
. First, we will create a new file, script.js
, in which we will define our controllers.
We will send a message from our parent controller to a child controller using $broadcast()
, and we will receive the event in our child controller and log it into the console using another method, $scope.$on()
, as shown below.
# angular
import angular from 'angular';
var app = angular.module('app', []);
app.controller("parentCtrl", function ($scope) {
$scope.handleClick = function (msg) {
$scope.$broadcast('sendMsg', { message: msg });
};
});
app.controller("childCtrl", function ($scope) {
$scope.$on('sendMsg', function (event, args) {
$scope.message = args.message;
console.log($scope.message);
});
});
Now, let’s create a frontend in index.html
, in which we will create an input that will take a message from the parent controller. When we click on the send
button, it will send it to the child controller.
The child controller will receive the message and log it onto the console, as shown below.
# angular
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="lib/style.css" />
<script src="lib/script.js"></script>
</head>
<body ng-app="app">
<div ng-controller="parentCtrl">
<h1>Send to Child controller</h1>
<input ng-model="msg">
<button ng-click="handleClick(msg);">Broadcast</button>
<br /><br />
<div ng-controller="childCtrl">
</div>
</div>
</body>
</html>
Output:
Console:
From the above example, using $broadcast()
, we sent events or messages from parent controllers to child controllers. And using the $scope.$on()
method, we received the event or message in the child controller and did some functions.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn