Bootstrap Tooltip in AngularJS
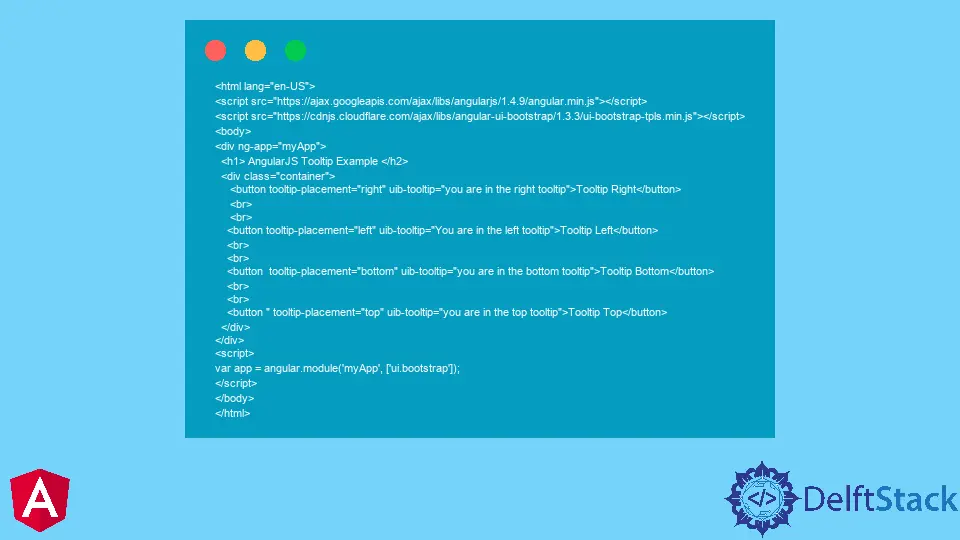
The Bootstrap tooltip is one of the most popular JavaScript libraries that can be used for implementing tooltips in AngularJS applications.
Bootstrap tooltip is an open-source library that provides users with several customizable features like animation effects, positioning, and tooltip placement.
Moreover, tooltips are commonly used to provide help and instructions for users who may not know how to do something or who may be struggling with a particular task. This tutorial will show you how to create Bootstrap tooltips with AngularJS.
Install Bootstrap Tooltip Plugin
The first step is to add the Bootstrap tooltip plugin and AngularJS library to your web page. Install the bootstrap plugin by running the following command in the terminal.
Command:
npm install bootstrap-tooltip --save
Use Bootstrap Tooltip in HTML
Code:
<html lang="en-US">
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.9/angular.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular-ui-bootstrap/1.3.3/ui-bootstrap-tpls.min.js"></script>
<body>
<div ng-app="myApp">
<h1> AngularJS Tooltip Example </h2>
<div class="container">
<button tooltip-placement="right" uib-tooltip="you are in the right tooltip">Tooltip Right</button>
<br>
<br>
<button tooltip-placement="left" uib-tooltip="You are in the left tooltip">Tooltip Left</button>
<br>
<br>
<button tooltip-placement="bottom" uib-tooltip="you are in the bottom tooltip">Tooltip Bottom</button>
<br>
<br>
<button " tooltip-placement="top" uib-tooltip="you are in the top tooltip">Tooltip Top</button>
</div>
</div>
<script>
var app = angular.module('myApp', ['ui.bootstrap']);
</script>
</body>
</html>
Here we added four tooltips. When you hover on these tooltips, a small triangle will show you some additional information about the tooltip.
This is the best method that will guide non-technical people. Bootstrap Tooltip can be used in any project that needs a tooltip with the help of AngularJS.
Click here to check the complete working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook