Array Mapping in AngularJS
- Understanding Array Mapping in AngularJS
- Implementing Array Mapping in AngularJS
- Benefits of Using Array Mapping in AngularJS
- Common Use Cases for Array Mapping in AngularJS
- Conclusion
- FAQ
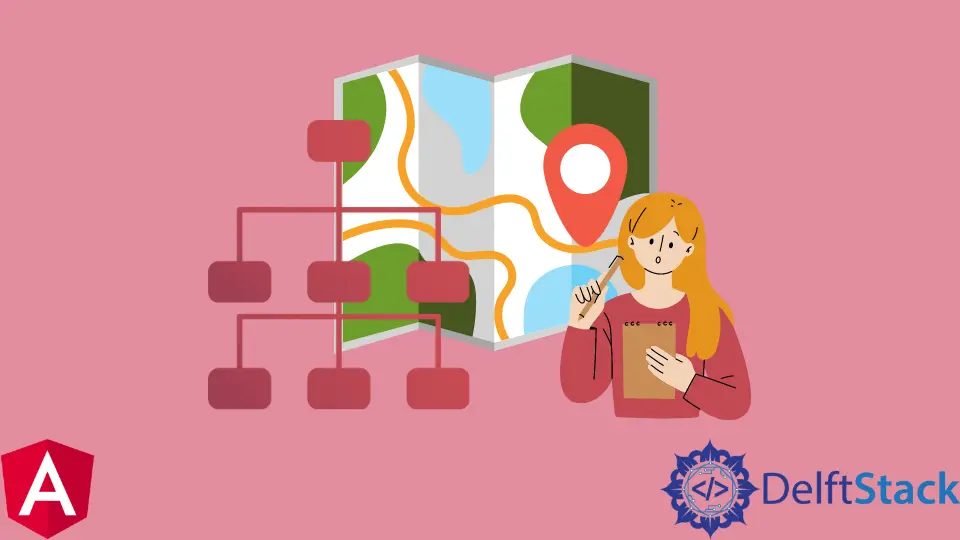
Array mapping in AngularJS is a powerful feature that allows developers to manipulate and transform arrays efficiently. It enables you to create new variables in a concise manner, making your code cleaner and more readable.
In this article, we will explore the concept of array mapping in AngularJS, its significance, and how to effectively implement it in your applications. By the end, you’ll have a solid understanding of how to leverage array mapping to enhance your AngularJS projects.
Understanding Array Mapping in AngularJS
Array mapping is a method that allows you to traverse through an array and apply a function to each element, producing a new array with the results. This is particularly useful when you want to transform data from one format to another or when you need to extract specific properties from objects within an array. In AngularJS, the map
function can be used seamlessly to achieve this.
For instance, consider an array of user objects where you want to extract the names. Using array mapping, you can achieve this in a single line of code, making your application both efficient and easy to understand.
Implementing Array Mapping in AngularJS
To implement array mapping in AngularJS, you can use the following syntax:
let users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' }
];
let userNames = users.map(user => user.name);
Output:
['Alice', 'Bob', 'Charlie']
In this example, we have an array of user objects, each containing an id
and a name
. The map
function iterates over each user object and returns a new array containing only the names. This concise approach not only reduces the amount of code you need to write but also improves the readability of your application.
Benefits of Using Array Mapping in AngularJS
The benefits of using array mapping in AngularJS are numerous. Firstly, it simplifies your code. Instead of writing loops and conditionals to extract data, you can achieve the same result with a straightforward mapping function. This leads to cleaner and more maintainable code.
Secondly, array mapping enhances performance. By using built-in JavaScript methods like map
, you can take advantage of optimizations that the JavaScript engine provides, resulting in faster execution times.
Lastly, array mapping promotes a functional programming style, which is a key aspect of AngularJS. This paradigm encourages immutability and side-effect-free functions, making your code less prone to bugs.
Common Use Cases for Array Mapping in AngularJS
Array mapping can be applied in various scenarios in AngularJS applications. Here are a few common use cases:
- Transforming Data: You might need to convert data formats, such as changing an array of objects into an array of strings or numbers.
- Filtering Data: Although filtering is typically done with the
filter
method, you can combinemap
withfilter
to create a new array based on specific criteria. - Displaying Data: When rendering lists in your AngularJS templates, you can use array mapping to prepare the data for display.
For example, if you have an array of products and you want to create a list of product names for display, you can do so with mapping:
let products = [
{ id: 1, name: 'Laptop', price: 1000 },
{ id: 2, name: 'Phone', price: 500 },
{ id: 3, name: 'Tablet', price: 300 }
];
let productNames = products.map(product => product.name);
Output:
['Laptop', 'Phone', 'Tablet']
In this code snippet, we extract the names of the products to create a new array that can be easily used in the user interface. This approach not only keeps your code clean but also makes it easier to manage and update.
Conclusion
Array mapping in AngularJS is an essential feature that enhances the way developers handle arrays. By allowing for concise data manipulation, it promotes cleaner code and improves performance. Whether you’re transforming data or preparing it for display, understanding how to use array mapping effectively can significantly enhance your AngularJS applications. Embrace this powerful tool and elevate your coding skills to the next level.
FAQ
-
what is array mapping in AngularJS?
Array mapping in AngularJS is a method that allows developers to transform and manipulate arrays efficiently using themap
function. -
how does array mapping improve code readability?
Array mapping simplifies data extraction and transformation, allowing developers to write cleaner and more concise code. -
can array mapping be used for filtering data?
While filtering is typically done with thefilter
method, you can combinemap
withfilter
to create new arrays based on specific criteria.
-
what are the performance benefits of using array mapping?
Array mapping leverages built-in JavaScript optimizations, resulting in faster execution times compared to traditional loops. -
how can I implement array mapping in my AngularJS application?
You can implement array mapping by using themap
function on an array, passing a callback function that defines how each element should be transformed.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook