Controller as Syntax in AngularJS
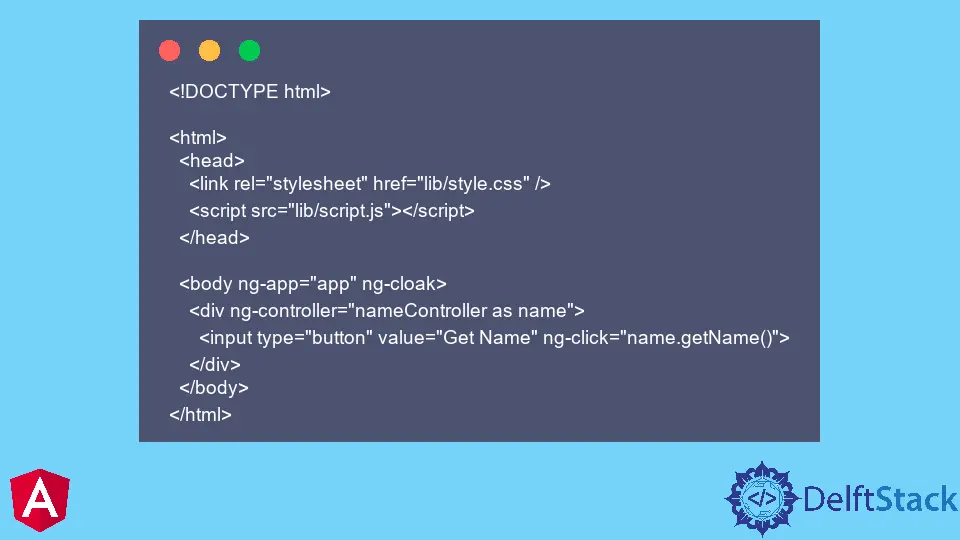
We will introduce controller as
syntax in AngularJS with examples.
Controller as
Syntax in AngularJS
In the 1.1.5
release of AngularJS, Controller as
syntax was introduced. It has a significant impact on the way we think about AngularJS.
This was added as an experimental feature. This is an essential feature and helps us use controller functions faster and easier.
We can use controller as
syntax to call the controller directly access the controller. Let’s go through an example and create a new controller with a simple function to print a name.
Let’s create a new project in AngularJS and go through an example of controller as
syntax. First of all, we will add the AngularJS library using script
tags.
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script>
Now we will define the AngularJS application using ng-app
.
<body ng-app="">
...
</body>
We will create a script.js
file and create a controller. This controller will have a function getName
that will log in to the console, as shown below.
import angular from 'angular';
angular.module('app', []).controller('nameController', function() {
this.getName = function(){
console.log("Activated!")
}
});
We will create the frontend for our application in index.html
. As shown below, we will create a button that will call the function getName
whenever a user clicks on it.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="lib/style.css" />
<script src="lib/script.js"></script>
</head>
<body ng-app="app" ng-cloak>
<div ng-controller="nameController as name">
<input type="button" value="Get Name" ng-click="name.getName()">
</div>
</body>
</html>
Output:
Console output:
The above example shows the controller as
syntax, making it easy for us to call the function and remember the controller’s name as a keyword or a short word that can be typed easily.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn