How to Use ngSwitch in Angular
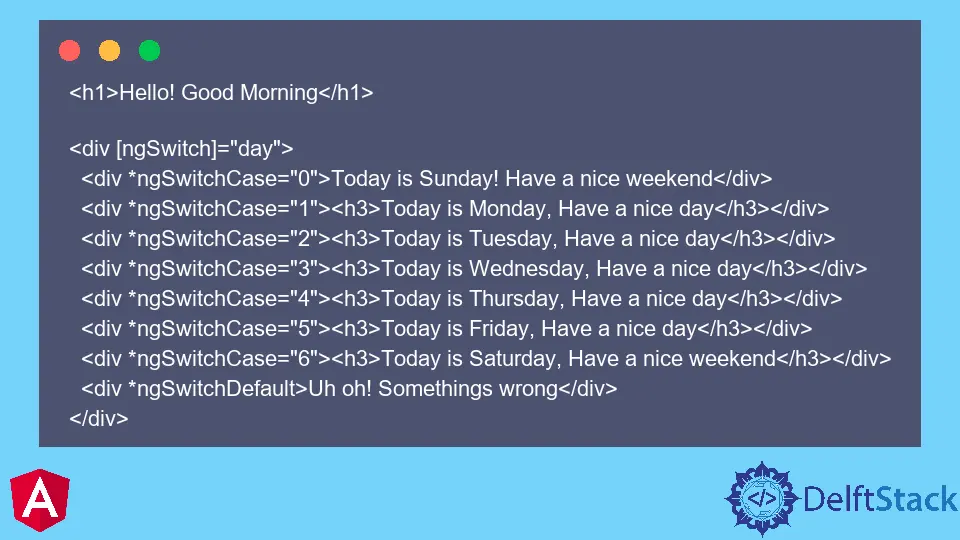
You have heard and used switch
statements in general programming languages at least one time.
A switch
statement is used to execute a block of code when there are many if
statements, and we convert those if
statements into switch
statements to save time and make it work more efficiently.
In this tutorial, we will go through an example in which we will create a scenario so that the switch
statement will be executed based on the day of the week and display a certain block of code for a certain day of the week.
How to Use the ngSwitch
in Angular
Let’s create a new application using the following command.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
We will create a new component using the following command.
# angular
ng g c greeting
In greeting.component.ts
, we will create a day to store the current day of the week.
# angular
day = new Date().getDay();
We will create a switch
statement so that if the day returns 0
, it will be a Sunday, and 1
will be Monday, and so on. So we will bind day
using [ngSwitch]
and use ngSwitchCase
to display different views for each day in greeting.component.html
.
<h1>Hello! Good Morning</h1>
<div [ngSwitch]="day">
<div *ngSwitchCase="0">Today is Sunday! Have a nice weekend</div>
<div *ngSwitchCase="1"><h3>Today is Monday, Have a nice day</h3></div>
<div *ngSwitchCase="2"><h3>Today is Tuesday, Have a nice day</h3></div>
<div *ngSwitchCase="3"><h3>Today is Wednesday, Have a nice day</h3></div>
<div *ngSwitchCase="4"><h3>Today is Thursday, Have a nice day</h3></div>
<div *ngSwitchCase="5"><h3>Today is Friday, Have a nice day</h3></div>
<div *ngSwitchCase="6"><h3>Today is Saturday, Have a nice weekend</h3></div>
<div *ngSwitchDefault>Uh oh! Somethings wrong</div>
</div>
Output:
The above example shows that ngSwitch
has displayed that the day is Saturday. So in this way, using ngSwitch
and ngSwitchCase
, we can display any block of code based on the case scenarios.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn