How to Use q all in AngularJs
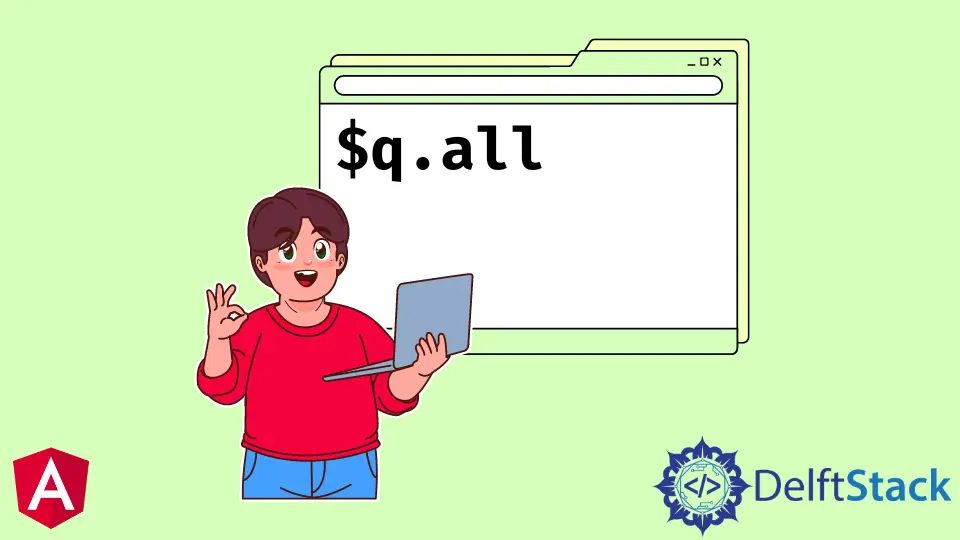
We will introduce promises
in AngularJs and what is $q.all
in AngularJs.
What Are Promises
in AngularJs?
If we make an HTTP request for a series of asynchronous functions, there can be a network failure, and we need to use callback
and errorCallback
. If we have a long function or multiple functions, we may need to use multiple callback
and errorCallback
to make our code messy.
Promises help us execute asynchronous functions in series without messing up our code. Promises are provided by the built-in service $q
.
What Is $q.all
and How to Use It in AngularJs?
As the name of $q.all
suggests, it combines multiple promises into a single promise resolved when all of them are resolved.
Let’s have an example to understand how we can use $q.all
. Let’s create two variables pR45
and pR55
.
# angularjs
angular.module("app",[])
.run(function($q) {
var pR45 = $q.when(45);
var pR55 = $q.when(55);
});
});
Now let’s use $q.all
to log the values of the variables in the console.
# angularjs
angular.module("app",[])
.run(function($q) {
var pR45 = $q.when(45);
var pR55 = $q.when(55);
$q.all([pR45,pR55]).then(function([r45,r55]) {
console.log(r45);
console.log(r55);
});
});
In the code above, $q.all
will take all the variables that we will pass to him, and once all of them are resolved, it will store the results from these variables to new variables r45
and r55
, and then we can use these results in our function as shown in the code above.
<script src="//unpkg.com/angular/angular.js"></script>
<body ng-app="app"></body>
Run the HTML code above and the AngularJS script then you will get the following output.
We can use the $q.all
function to execute asynchronous functions in these easy steps.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn