On Load in Angular
Rana Hasnain Khan
Mar 20, 2022
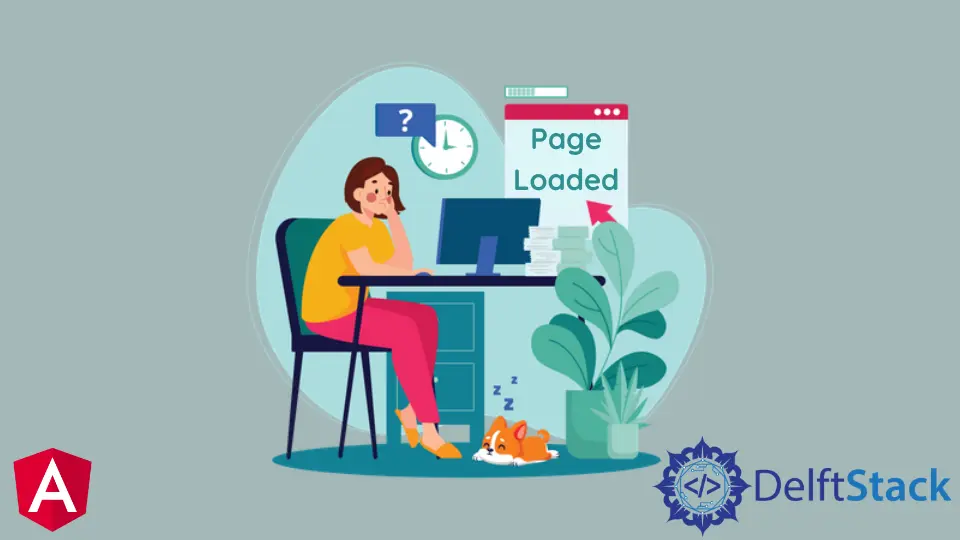
We will introduce a function when a page is loaded in Angular.
On Load in Angular
When we want to execute a function when a page or component is loaded, we call that function on init
.
Let’s start with an example. First, we will create a view.
# angular
<body>
<div ng-app="newapp" ng-controller="newcontroller" data-ng-init="init()">
<h2>Page Loaded</h2>
</div>
</body>
We defined our ng-app
, ng-controller
, initialized component
, and data-ng-init
in the above code.
Now, let’s write a function that will display an output on the console when the page is loaded.
# angular
var app = angular.module("newapp", []);
app.controller("newcontroller", function($scope){
$scope.init = function(){
console.log("Page Loaded")
}
})
Output:
The example we gave shows how easy it is to call a function on page load. We can easily call any function using the above example.
Author: Rana Hasnain Khan
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn