How to Use Angular ngAfterViewInit Method
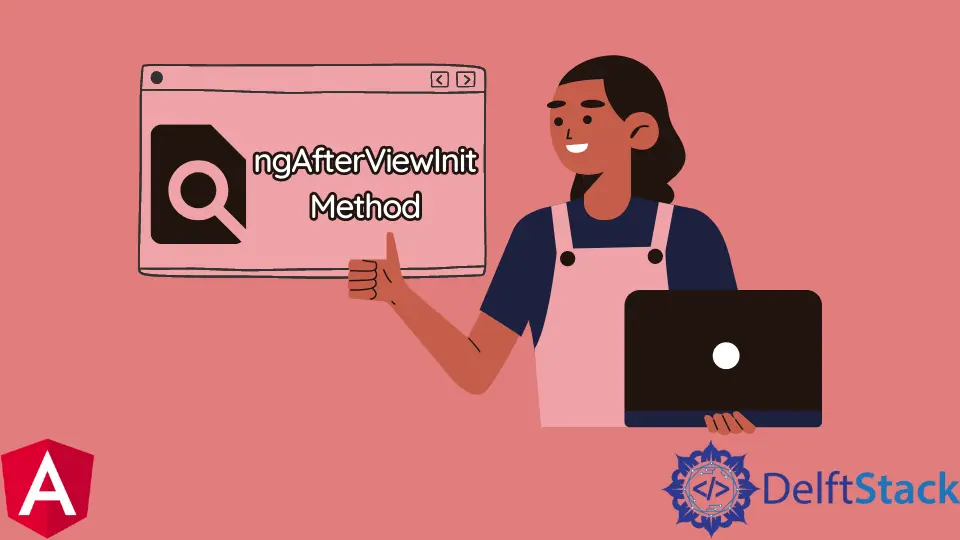
We will introduce the ngAfterViewInit()
method and use it in Angular applications.
the ngAfterViewInit()
Method in Angular
The ngAfterViewInit()
in Angular is a method of AfterViewInit
, and Angular completely initializes a lifecycle hook called after the component’s view.
We can use ngAfterViewInit()
to handle and perform any initialization tasks required in the component, or we want to do after the component’s view has been fully initialized.
We can access the properties annotated using ngAfterViewInit()
with two decorators, @ViewChild()
and @ViewChildren()
decorators.
We will go through an example to understand how ngAfterViewInit()
works and how we can use it in our Angular applications.
Let’s create a new application by using the following command.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
In our app.component.ts
, we will create a method ngAfterViewInit()
to console.log
our message. So our code will look like below.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
ngAfterViewInit() {
console.log("Sent from Ng After View Init");
}
}
Output:
In this way, we can use ngAfterViewInit()
to initialize any important things after the component’s view has been initialized by Angular.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn