How to Install Bootstrap in Angular
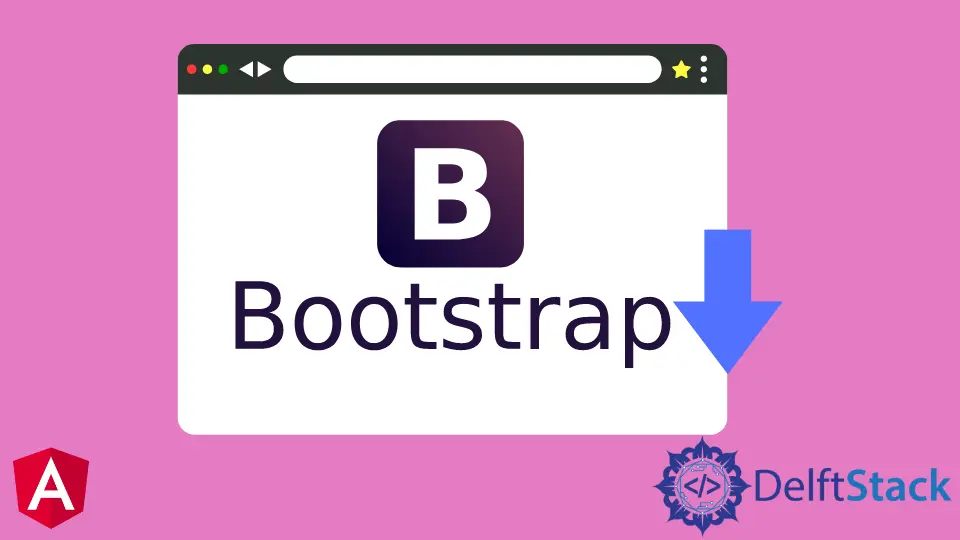
We will introduce how to install and use Bootstrap in Angular.
Install Bootstrap in Angular
Bootstrap
is a CSS framework used to quickly design a responsive and mobile-friendly website. It’s the most popular framework used widely.
We can also install Bootstrap in our Angular application by following these simple and easy steps. To create a new Angular application, we will do the command below in the terminal.
# Terminal
ng new my-app
cd my-app
The command above creates a new Angular project and takes us into the my-app
project directory.
Now we need to install Bootstrap in our Angular project, and to do that, we need to go to the terminal and run the following command.
# cli
npm install bootstrap --save
This command will install the Bootstrap in our Angular project. But if you are using SASS
in your project, install bootstrap-sass
instead.
# cli
npm install bootstrap-sass --save
Once you have installed Bootstrap, it will be saved in node modules, and now we only need to import Bootstrap CSS wherever we want to use Bootstrap using the following import commands.
# angular
@import '~bootstrap/dist/css/bootstrap.css';
Or if you are using SASS
.
# angular
$icon-font-path: '~bootstrap-sass/assets/fonts/bootstrap/';
@import '~bootstrap-sass/assets/stylesheets/bootstrap';
We can easily install Bootstrap in our Angular applications and make it responsive and mobile-friendly by using Bootstrap classes by following these simple steps.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn