How to Inject Document in Service in Angular
- Understanding Document Injection in Angular
- Method 1: Using Angular’s Dependency Injection
- Method 2: Manipulating the Document Object
- Method 3: Reading External HTML Content
- Conclusion
- FAQ
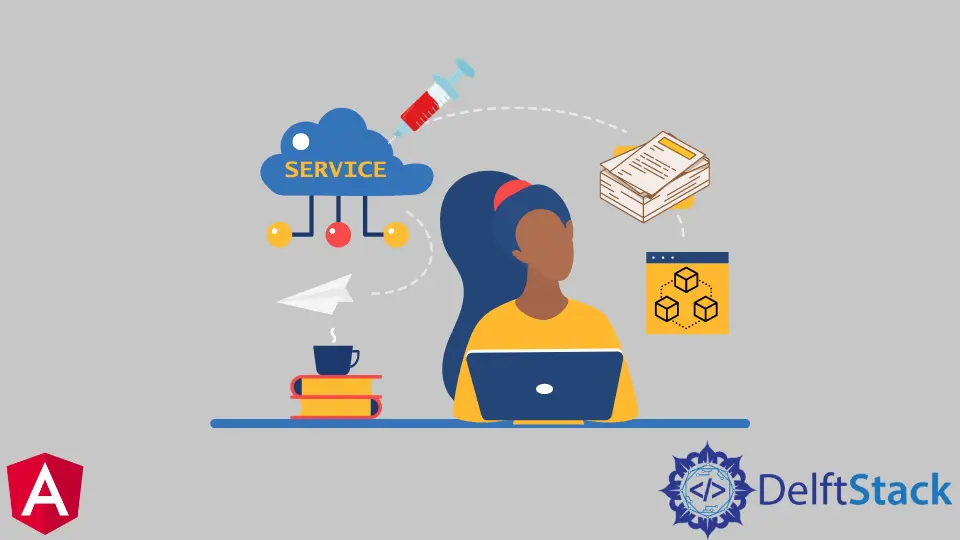
Injecting documents in a service is a powerful technique in Angular that allows developers to read and parse HTML content dynamically. This capability is particularly useful when working with data fetched from external sources or when you need to manipulate the DOM based on user interactions. Understanding how to inject documents into services can enhance your Angular applications’ functionality and improve user experience.
In this article, we’ll explore the methods to achieve this, complete with practical code examples and detailed explanations. Whether you are a beginner or an experienced developer, this guide will help you grasp the concept of document injection in Angular services.
Understanding Document Injection in Angular
Before diving into the implementation, it is essential to understand what document injection means in the context of Angular. Essentially, it involves using Angular’s dependency injection system to access the document object, which represents the HTML document loaded in the browser. This is crucial for manipulating the DOM, reading HTML content, or performing tasks like parsing and rendering data dynamically.
Angular provides a robust service architecture that allows you to create services that can manage data and business logic. By injecting the document into these services, you can create a seamless interaction between your application and the HTML content. This article will guide you through the steps to effectively inject documents into your services.
Method 1: Using Angular’s Dependency Injection
One of the simplest ways to inject a document in an Angular service is through Angular’s built-in dependency injection system. This method allows you to access the document object directly within your service, enabling you to read and manipulate HTML content easily.
Here’s a basic example of how to do this:
import { Injectable } from '@angular/core';
import { DOCUMENT } from '@angular/common';
@Injectable({
providedIn: 'root'
})
export class DocumentService {
constructor(@Inject(DOCUMENT) private document: Document) {}
getDocumentContent(): string {
return this.document.documentElement.innerHTML;
}
}
Output:
<html> ... </html>
In this example, we first import the necessary modules and create a service called DocumentService
. The service constructor uses Angular’s @Inject
decorator to inject the document object. The getDocumentContent
method retrieves the entire HTML content of the document. This method can be called from any component or service in your Angular application.
By using Angular’s dependency injection, you can easily access the document object without having to manipulate the global scope. This approach promotes cleaner code and better maintainability.
Method 2: Manipulating the Document Object
Once you have injected the document into your service, you can perform various manipulations to read or modify the HTML content. This can be particularly useful for dynamically updating the content based on user interactions or external data.
Here’s an example of how to manipulate the document object:
import { Injectable } from '@angular/core';
import { DOCUMENT } from '@angular/common';
@Injectable({
providedIn: 'root'
})
export class DocumentService {
constructor(@Inject(DOCUMENT) private document: Document) {}
changeTitle(newTitle: string): void {
this.document.title = newTitle;
}
appendContent(newContent: string): void {
const body = this.document.body;
const newElement = this.document.createElement('div');
newElement.innerHTML = newContent;
body.appendChild(newElement);
}
}
Output:
Title changed to newTitle and new content appended to the body.
In this example, we extend our DocumentService
by adding two methods: changeTitle
and appendContent
. The changeTitle
method updates the document’s title, while the appendContent
method creates a new div
element and appends it to the body of the document. This allows for dynamic updates to the HTML content based on user actions or data fetched from APIs.
Manipulating the document object in this way can enhance the user experience by providing real-time updates and interactions within your Angular application.
Method 3: Reading External HTML Content
Another powerful feature of injecting documents into services is the ability to read external HTML content. This can be particularly useful when you want to load templates or content from external sources dynamically.
Here’s how you can achieve this:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DocumentService {
constructor(private http: HttpClient) {}
fetchHtmlContent(url: string): Observable<string> {
return this.http.get(url, { responseType: 'text' });
}
}
Output:
HTML content fetched from the external source.
In this example, we utilize Angular’s HttpClient
to fetch HTML content from an external URL. The fetchHtmlContent
method returns an observable that emits the HTML content as a string. This allows components to subscribe to the observable and handle the received content accordingly.
This method is particularly useful for loading templates or content that can change frequently, providing a flexible way to manage HTML content in your Angular application.
Conclusion
Injecting documents in Angular services is a valuable technique that allows developers to read and manipulate HTML content efficiently. By leveraging Angular’s dependency injection system, you can create services that enhance your application’s functionality and improve user experience. Whether you’re changing titles, appending content, or fetching HTML from external sources, the methods outlined in this article will help you master document injection in Angular. By integrating these techniques into your projects, you can create dynamic and responsive applications that meet users’ needs effectively.
FAQ
-
What is document injection in Angular?
Document injection in Angular refers to the practice of using Angular’s dependency injection system to access and manipulate the document object representing the HTML content in the browser. -
How can I read HTML content in an Angular service?
You can read HTML content in an Angular service by injecting the document object and using methods likeinnerHTML
to retrieve the desired content.
-
Can I manipulate the document’s title using Angular services?
Yes, you can manipulate the document’s title by injecting the document object and updating thetitle
property within your service. -
What is the role of HttpClient in fetching external HTML content?
HttpClient is an Angular service that allows you to make HTTP requests to fetch data, including external HTML content, which can be processed and displayed in your application. -
Is it possible to append new content to the document body dynamically?
Yes, by injecting the document object into your service, you can create new elements and append them to the document body dynamically.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook