How to Use Blur Event in Angular
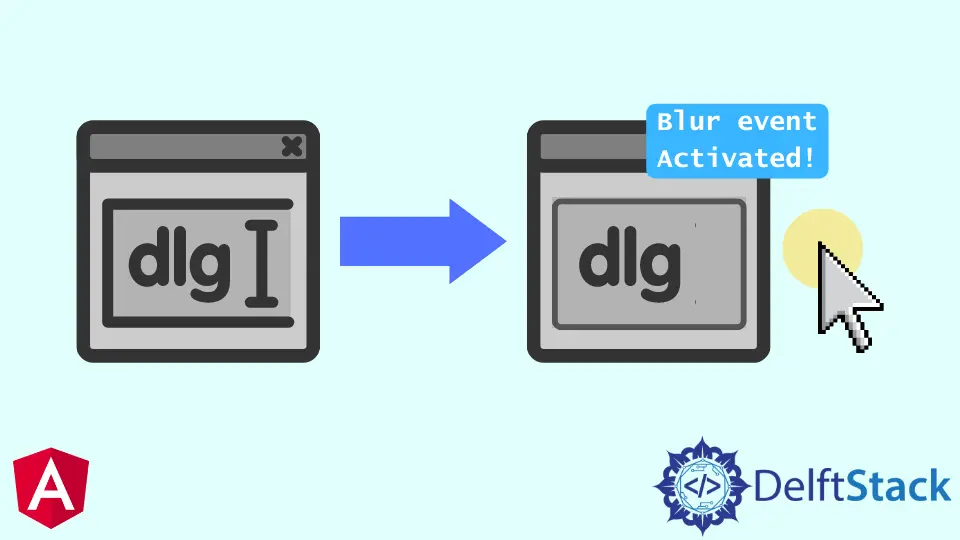
This article will introduce the blur
event in Angular with examples.
Blur in Angular
There are many situations where we may need to use blur
events in Angular. For example, for validating fields, we may want to validate a certain field when the focus from the field is lost.
Angular provides blur
events for this kind of situation.
The blur
event is set off when an element loses its focus. The syntax of the blur
event in Angular is shown below.
<input (blur)='DoSomething()'/>
Let’s create a new application in Angular using the following command.
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
ng serve --open
In app.component.ts
, we will create a function triggered on a blur
event, as shown below.
import { Component } from '@angular/core';
type Fruit = Array<{ id: number; name: string }>;
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
blurEvent(): void {
console.log('Blur event Activated!');
}
}
In app.component.html
, we will create an input
element and set a blur
event, as shown below.
<label>Enter Name:</label>
<input type="text" placeholder="Enter name.." (blur)="blurEvent()">
Now, let’s serve the Angular app by running the following command.
ng serve
Output:
The above example shows that the blur
event is activated whenever the focus is lost from the input.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn