Angular 2 Global Constants
- Understanding Angular 2 Global Constants
- Creating Global Constants in Angular 2
- Benefits of Using Global Constants
- Best Practices for Managing Global Constants
- Conclusion
- FAQ
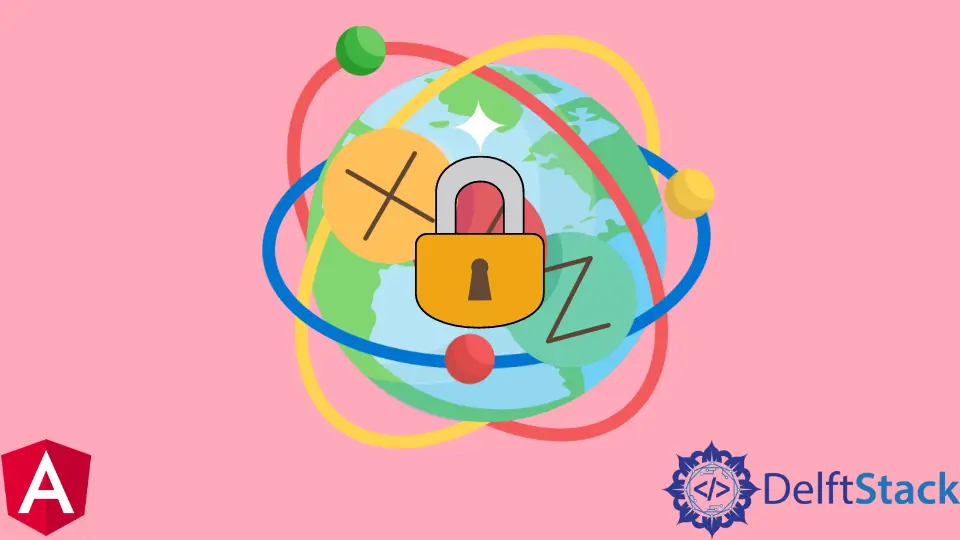
In the world of Angular development, managing data efficiently is crucial for building robust applications. One effective way to handle shared data across various components is through Angular 2 global constants. These constants serve as a centralized storage solution, allowing developers to define values in a global namespace. This approach not only streamlines data management but also enhances code readability and maintainability. Whether you’re working on a large-scale enterprise application or a small project, understanding how to implement global constants can significantly improve your workflow.
In this article, we will explore the concept of Angular 2 global constants, their benefits, and how to effectively utilize them in your applications.
Understanding Angular 2 Global Constants
Angular 2 global constants are essentially variables that hold values accessible throughout the application. By storing frequently used data, such as API endpoints, configuration settings, or application-wide constants, developers can avoid redundancy and ensure consistency across different parts of the application. This approach is particularly beneficial when dealing with large applications where multiple components need to access the same data.
To create a global constant in Angular 2, you typically define it in a dedicated file and then import it wherever needed. This not only keeps your code organized but also allows for easy updates and management of these constants.
Creating Global Constants in Angular 2
To define global constants in Angular 2, you need to follow a few simple steps. First, create a new file where you’ll store your constants. For example, you might create a file named app.constants.ts
. Here’s how you can set it up:
export const API_URL = 'https://api.example.com';
export const APP_TITLE = 'My Angular App';
In this code snippet, we define two constants: API_URL
and APP_TITLE
. The API_URL
holds the base URL for API requests, while APP_TITLE
stores the title of the application.
Next, you can import these constants into any component or service where they are needed. Here’s an example of how to use them in a component:
import { Component } from '@angular/core';
import { API_URL, APP_TITLE } from './app.constants';
@Component({
selector: 'app-root',
template: `<h1>{{ title }}</h1>`,
})
export class AppComponent {
title = APP_TITLE;
fetchData() {
fetch(API_URL)
.then(response => response.json())
.then(data => console.log(data));
}
}
In this component, we import the constants and use them to set the title of the application and fetch data from the API. This not only makes the code cleaner but also ensures that any changes to the constants are reflected throughout the application.
Output:
My Angular App
By defining your constants in a separate file, you can easily manage and update them without having to search through multiple components. This approach promotes better organization and helps maintain a clean codebase.
Benefits of Using Global Constants
Utilizing global constants in your Angular 2 applications comes with several advantages. First and foremost, it enhances code maintainability. When you define a constant in one place, any changes you make will automatically propagate throughout your application, reducing the risk of inconsistencies.
Additionally, global constants improve code readability. Instead of hardcoding values directly into your components, using descriptive constant names makes it easier for other developers (or even yourself) to understand the purpose of each value. This clarity can significantly reduce onboarding time for new team members and facilitate collaboration.
Moreover, global constants can help optimize performance. By reducing the number of times you need to declare the same value, you can minimize memory usage and improve the efficiency of your application. This is especially important in larger applications where performance can be a critical factor.
In summary, the use of global constants in Angular 2 is a best practice that can lead to cleaner, more maintainable, and efficient code.
Best Practices for Managing Global Constants
While using global constants can greatly benefit your Angular application, there are best practices to ensure you’re implementing them effectively. First, always use descriptive names for your constants. Instead of naming a constant URL
, consider a more specific name like USER_API_URL
. This practice improves clarity and reduces confusion.
Secondly, organize your constants logically. Group related constants together in the same file or module. For instance, you might have a file for API-related constants, another for UI configurations, and so on. This organization helps keep your codebase clean and navigable.
Additionally, consider using TypeScript enums for constants that represent a set of related values. This approach not only provides better type safety but also enhances code readability. Here’s an example of using an enum:
export enum UserRoles {
Admin = 'ADMIN',
User = 'USER',
Guest = 'GUEST',
}
In this case, the UserRoles
enum defines a set of user roles, making it easy to manage and reference throughout the application.
Output:
ADMIN, USER, GUEST
Finally, document your constants. Providing comments or documentation can help future developers understand the purpose and usage of each constant. This practice is especially important in larger teams or projects that may evolve over time.
Conclusion
In conclusion, Angular 2 global constants are a powerful tool for managing shared data across your applications. By centralizing values in a global namespace, you can enhance code maintainability, improve readability, and optimize performance. As you implement global constants, remember to follow best practices like using descriptive names, organizing them logically, and documenting their usage. With these strategies in place, you’ll be well on your way to creating cleaner, more efficient Angular applications.
FAQ
-
What are global constants in Angular 2?
Global constants in Angular 2 are values stored in a global namespace, accessible throughout the application, which helps manage shared data efficiently. -
How do I create a global constant in Angular 2?
You create a global constant by defining it in a separate file and exporting it, then importing it in any component or service where it is needed. -
Why should I use global constants?
Using global constants enhances code maintainability, improves readability, and helps optimize performance by reducing redundancy in your code. -
Can I use enums for global constants?
Yes, using TypeScript enums for related constants is a best practice, as it provides better type safety and improves code clarity. -
How can I manage global constants effectively?
To manage global constants effectively, use descriptive names, organize them logically, and document their usage to ensure clarity for future developers.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook