How to Understand Concept of Angular 2 ng-disabled
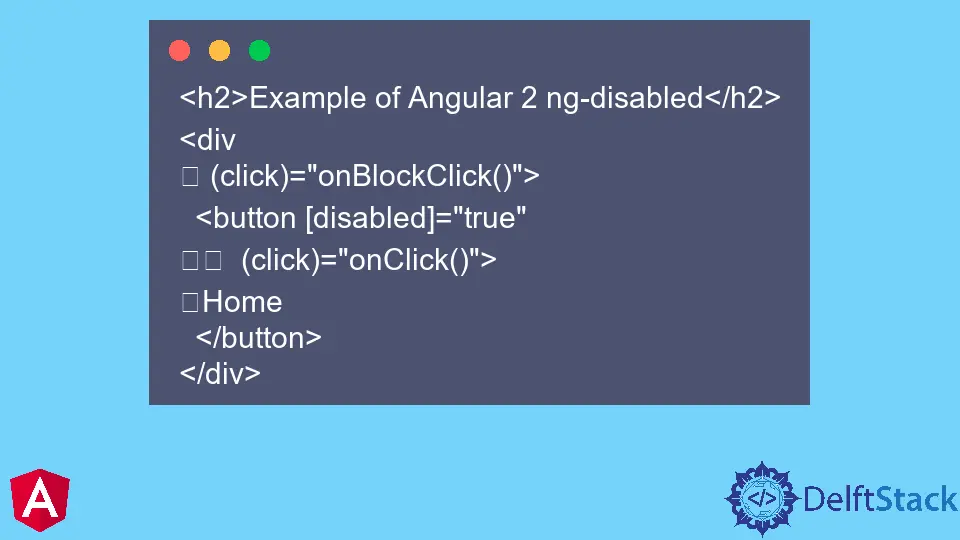
Angular 2 ng-disabled
is a directive that allows developers to disable a form control. It can be used to prevent the user from entering data into a form field, or it can be used to disable an input field that is pre-filled with data.
This attribute can be added to any HTML element, including input, button, select, and text area elements.
The ng-disabled
directive will not change the element’s appearance, but it will prevent user interaction with it. It can be applied in various scenarios, such as disabling an input field when a checkbox is unchecked or disabling a button when the user has not logged in.
Steps to Use Angular 2 ng-disabled
Directive
The simplest way to use ng-disabled
is to set the disabled
property to true
on the Component
’s class. This will disable the component, and it will not be rendered in the DOM.
This article will take you through the steps to use Angular 2 ng-disabled
.
-
Import the module
Import the
ng-disabled
module into yourapp.module.ts
file. -
Add the TypeScript code
import { Component } from '@angular/core'; @Component({ selector: 'my-app', templateUrl: './app.component.html', styleUrls: [ './app.component.css' ] }) export class AppComponent { public onClick(): void { console.log('> click'); } public onBlockClick(): void { console.log('> stopped'); } }
-
Disable components in your application logic
Disable components in your application logic by adding
ng-disabled
as an input parameter and setting it totrue
, like this:<h2>Example of Angular 2 ng-disabled</h2> <div (click)="onBlockClick()"> <button [disabled]="true" (click)="onClick()"> Home </button> </div>
Here we disabled the
Home
button. You can see the button, but you cannot interact with it.That’s how
ng-disabled
works in Angular 2.
Click here to check the live demonstration of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook