How to Implement Angular 2 Checkbox Two Way Data Binding
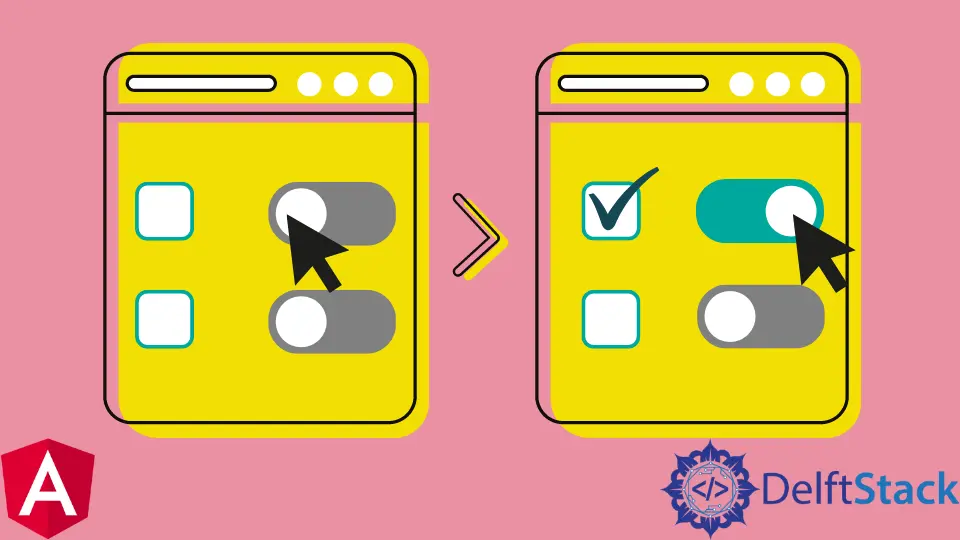
This angular article will look at different methods to carry out a checkbox two-way data binding.
We will use the ngModelOptions
attribute for two-way data binding. This solution best works when there is a name
attribute.
We will also introduce the ngModel
attribute that enables the checkboxFlag
to store the actual checkbox state when it is changed. Then we will use the change
attribute for data binding, such that when the value in the component is changed, the checkbox gets a new value as well.
When ngModel
is used in forms, it mostly does not work, so the ngModelOptions
attribute is best used to ensure the checkbox value changes as the data in the component is changed.
<input
type="checkbox"
[(ngModel)]="itemChange"
[ngModelOptions]="{standalone: true}"/>
In some cases, the code will not work if the input is not inside a form but forms part of the ngFor
loop. Also, the name
attribute needs to be included in the code set up to get the code to work well.
While the example code above handles two-way data binding on the frontend part, this solution carries out changes on the backend, making proper use of ngModel
.
<input
[(ngModel)]="itemOne">
type="checkbox"/>
To get this code to work perfectly, import { FormsModule }
from @angular/forms
into app.module.ts
and add to imports array looking like this:
import { FormsModule } from'@angular/forms';
[...]
@NgModule({
imports: [
[...]
FormsModule
],
[...]
})
The name
attribute has to be unique for the default checked state to work as expected, and it has to be inside the input
tag. But this code will not work if you use the checkbox inside a ngFor
while repeating an array of objects, like so:
[{"checked":true},{"checked":false}]
Then we can as well use the change
attribute to make data binding on checkboxes. With this approach, we create the codes in a more detailed way.
The html component will look like this:
<input #saveCheckboxOne
id="saveCheckboxOne"
type="checkbox"
[checked]="saveCheckbox"
(change)="onSaveCheckboxChange(saveUserNameCheckBox.checked)" />
Then the component.js will be written like below.
public saveCheckbox:boolean;
public onSaveCheckboxChange(value:boolean){
this.saveUsername = value;
}
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn