Index de réinitialisation de Pandas DataFrame
-
Pandas DataFrame
reset_index()
Méthode -
Réinitialisation de l’index d’une DataFrame en utilisant la méthode
pandas.DataFrame.reset_index()
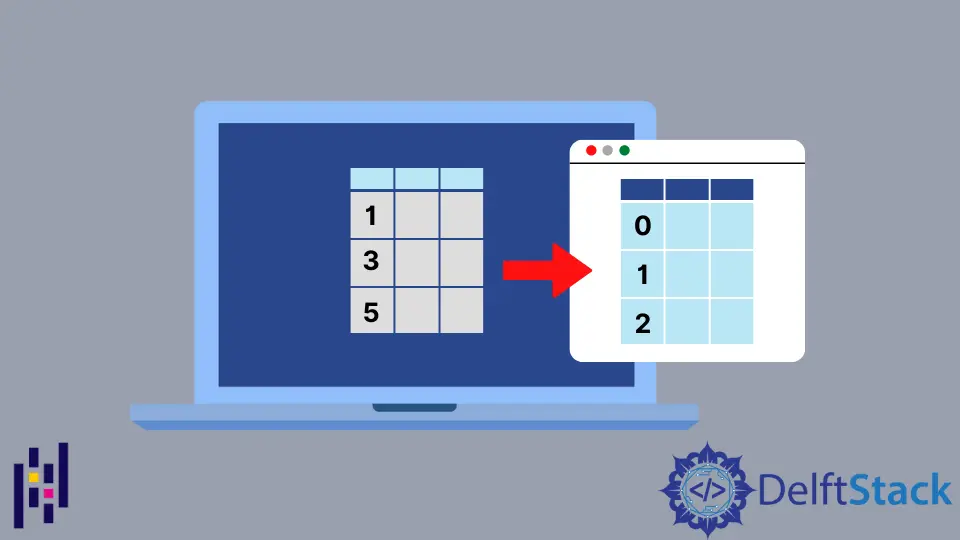
Ce tutoriel explique comment on peut réinitialiser l’index dans Pandas DataFrame en utilisant pandas.DataFrame.reset_index()
. La méthode reset_index()
fixe l’index de la DataFrame à l’index par défaut avec des nombres allant de 0
à (nombre de lignes dans la DataFrame-1)
.
Pandas DataFrame reset_index()
Méthode
Syntaxe
DataFrame.reset_index(level=None, drop=False, inplace=False, col_level=0, col_fill="")
Réinitialisation de l’index d’une DataFrame en utilisant la méthode pandas.DataFrame.reset_index()
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print(student_df)
Production :
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Supposons que nous ayons un DataFrame de cinq lignes et quatre colonnes tel qu’il est affiché dans la sortie. Nous avons également un index défini dans le DataFrame.
Réinitialisation de l’index d’une DataFrame en conservant l’index initial de la DataFrame comme une colonne
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=False)
print(student_df)
Production :
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
index Name Age City Grade
0 501 Alice 17 New York A
1 502 Steven 20 Portland B-
2 503 Neesham 18 Boston B+
3 504 Chris 21 Seattle A-
4 505 Alice 15 Austin A
Il réinitialise l’index de la DataFrame student_df
à l’index par défaut. Le inplace=True
fait le changement dans le DataFrame original lui-même. Si nous utilisons drop=False
, l’index initial est placé comme une colonne dans le DataFrame après avoir utilisé la méthode reset_index()
.
Réinitialisation de l’index d’une DataFrame Suppression de l’index initial de la DataFrame
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=True)
print(student_df)
Production :
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Neesham 18 Boston B+
3 Chris 21 Seattle A-
4 Alice 15 Austin A
Il réinitialise l’index de la DataFrame student_df
à l’index par défaut. Comme nous avons mis drop=True
dans la méthode reset_index()
, l’index initial est supprimé du DataFrame.
Réinitialisation de l’index d’un DataFrame après la suppression de lignes
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
}
)
student_df.drop([2, 3], inplace=True)
print("Initial DataFrame:")
print(student_df)
print("")
student_df.reset_index(inplace=True, drop=True)
print("DataFrame after reset_index:")
print(student_df)
Production :
Initial DataFrame:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
4 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Alice 15 Austin A
Comme nous pouvons le voir dans la sortie, il nous manque des indices après avoir supprimé des lignes. Dans ce cas, nous pouvons utiliser la méthode reset_index()
pour utiliser l’index sans valeurs manquantes.
Si nous voulons que l’index initial soit placé dans la colonne du DataFrame, nous pouvons utiliser drop=False
dans la méthode reset_index()
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn