Méthode insert des Pandas
-
pandas.DataFrame.insert()
Méthode en Python -
Mettre
allow_duplicates = True
dans la méthodeinsert()
pour ajouter une colonne déjà existante
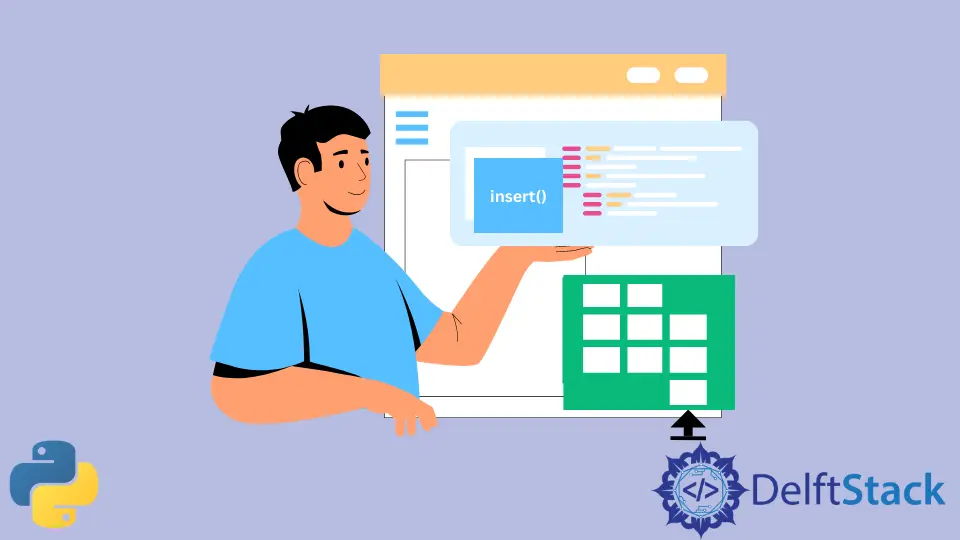
Ce tutoriel explique comment utiliser la méthode insert()
pour un Pandas DataFrame afin d’insérer une colonne dans le DataFrame.
import pandas as pd
countries_df = pd.DataFrame(
{
"Country": ["Nepal", "Switzerland", "Germany", "Canada"],
"Continent": ["Asia", "Europe", "Europe", "North America"],
"Primary Language": ["Nepali", "French", "German", "English"],
}
)
print("Countries DataFrame:")
print(countries_df, "\n")
Production :
Countries DataFrame:
Country Continent Primary Language
0 Nepal Asia Nepali
1 Switzerland Europe French
2 Germany Europe German
3 Canada North America English
Nous allons utiliser le DataFrame countries_df
montré dans l’exemple ci-dessus pour expliquer comment nous pouvons utiliser la méthode insert()
pour un DataFrame de Pandas pour insérer une colonne dans le DataFrame.
pandas.DataFrame.insert()
Méthode en Python
Syntaxe
DataFrame.insert(loc, column, value, allow_duplicates=False)
Il insère la colonne nommée column
dans le DataFrame
avec les valeurs spécifiées par value
à l’emplacement loc
.
Insérer une colonne ayant la même valeur pour toutes les lignes en utilisant la méthode insert()
import pandas as pd
countries_df = pd.DataFrame(
{
"Country": ["Nepal", "Switzerland", "Germany", "Canada"],
"Continent": ["Asia", "Europe", "Europe", "North America"],
"Primary Language": ["Nepali", "French", "German", "English"],
}
)
print("Countries DataFrame:")
print(countries_df, "\n")
countries_df.insert(3, "Capital", "Unknown")
print("Countries DataFrame after inserting Capital column:")
print(countries_df)
Production :
Countries DataFrame:
Country Continent Primary Language
0 Nepal Asia Nepali
1 Switzerland Europe French
2 Germany Europe German
3 Canada North America English
Countries DataFrame after inserting Capital column:
Country Continent Primary Language Capital
0 Nepal Asia Nepali Unknown
1 Switzerland Europe French Unknown
2 Germany Europe German Unknown
3 Canada North America English Unknown
Il insère la colonne Capital
dans le DataFrame countries_df
à la position 3
avec la même valeur de la colonne pour toutes les lignes mises à Unknown
.
La position commence à partir de 0
et donc la position 3
se réfère à la 4ème
colonne du DataFrame.
Insérer une colonne dans un DataFrame en spécifiant la valeur de chaque ligne
Si nous voulons spécifier les valeurs de chaque ligne pour la colonne à insérer en utilisant la méthode insert()
, nous pouvons passer une liste de valeurs comme argument value
dans la méthode insert()
.
import pandas as pd
countries_df = pd.DataFrame(
{
"Country": ["Nepal", "Switzerland", "Germany", "Canada"],
"Continent": ["Asia", "Europe", "Europe", "North America"],
"Primary Language": ["Nepali", "French", "German", "English"],
}
)
print("Countries DataFrame:")
print(countries_df, "\n")
capitals = ["Kathmandu", "Zurich", "Berlin", "Ottawa"]
countries_df.insert(2, "Capital", capitals)
print("Countries DataFrame after inserting Capital column:")
print(countries_df)
Production :
Countries DataFrame:
Country Continent Primary Language
0 Nepal Asia Nepali
1 Switzerland Europe French
2 Germany Europe German
3 Canada North America English
Countries DataFrame after inserting Capital column:
Country Continent Capital Primary Language
0 Nepal Asia Kathmandu Nepali
1 Switzerland Europe Zurich French
2 Germany Europe Berlin German
3 Canada North America Ottawa English
Il insère la colonne Capital
dans le DataFrame countries_df
à la position 2
avec les valeurs spécifiées de chaque ligne pour la colonne Capital
dans le DataFrame.
Mettre allow_duplicates = True
dans la méthode insert()
pour ajouter une colonne déjà existante
import pandas as pd
countries_df = pd.DataFrame(
{
"Country": ["Nepal", "Switzerland", "Germany", "Canada"],
"Continent": ["Asia", "Europe", "Europe", "North America"],
"Primary Language": ["Nepali", "French", "German", "English"],
"Capital": ["Kathmandu", "Zurich", "Berlin", "Ottawa"],
}
)
print("Countries DataFrame:")
print(countries_df, "\n")
capitals = ["Kathmandu", "Zurich", "Berlin", "Ottawa"]
countries_df.insert(4, "Capital", capitals, allow_duplicates=True)
print("Countries DataFrame after inserting Capital column:")
print(countries_df)
Production :
Countries DataFrame:
Country Continent Primary Language Capital
0 Nepal Asia Nepali Kathmandu
1 Switzerland Europe French Zurich
2 Germany Europe German Berlin
3 Canada North America English Ottawa
Countries DataFrame after inserting Capital column:
Country Continent Primary Language Capital Capital
0 Nepal Asia Nepali Kathmandu Kathmandu
1 Switzerland Europe French Zurich Zurich
2 Germany Europe German Berlin Berlin
3 Canada North America English Ottawa Ottawa
Il ajoute la colonne Capital
au DataFrame countries_df
même si la colonne Capital
existe déjà dans le DataFrame countries_df
.
Si nous essayons d’insérer la colonne qui existe déjà dans le DataFrame sans mettre allow_duplicates = True
dans la méthode insert()
, cela va nous lancer une erreur avec le message : ValueError : cannot insert column, already exists.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInArticle connexe - Pandas DataFrame Column
- Comment obtenir les en-têtes de colonne de Pandas DataFrame sous forme de liste
- Comment supprimer une colonne de Pandas DataFrame
- Comment convertir la colonne DataFrame en date-heure dans Pandas
- Comment obtenir la somme de la colonne Pandas
- Comment changer l'ordre des colonnes de Pandas DataFrame
- Comment convertir une colonne de DataFrame en chaîne de caractères dans Pandas