Tutorial de PyQt5 - Pulsador
-
Botón PyQt5 -
QPushButton
-
Estilo del conjunto de widgets de etiquetas PyQt5
QLabel
-
Evento de clic en la etiqueta PyQt5
QLabel
Label
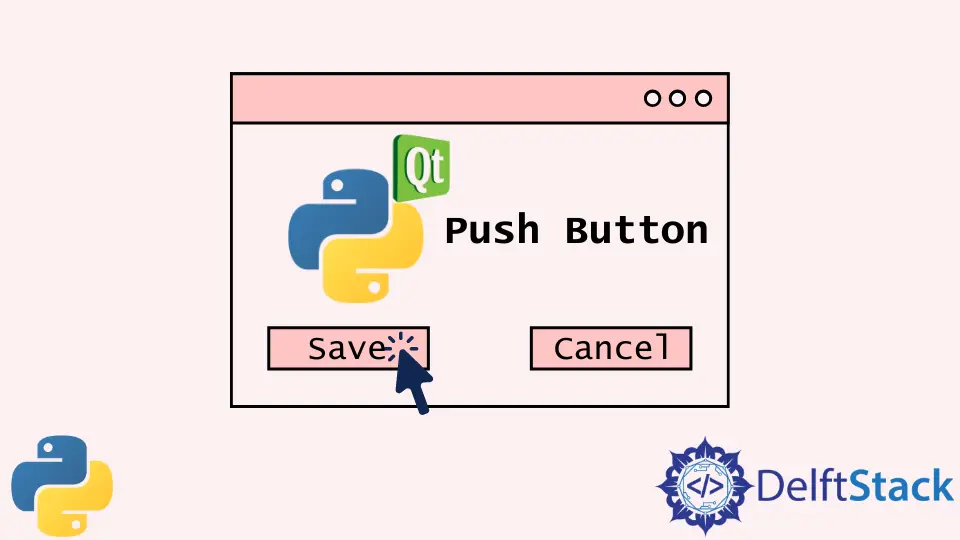
El widget QPushButton
es un botón de comando en PyQt5. El usuario lo pulsa para ordenar al PC que realice alguna acción específica, como Apply
, Cancel
y Save
.
Botón PyQt5 - QPushButton
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
Dónde,
buttonA = QtWidgets.QPushButton(windowExample)
El buttonA
es el QPushButton
de QtWidgets
y debería ser añadido a la ventana windowExample
igual que las etiquetas introducidas en los últimos capítulos.
buttonA.setText("Click!")
Establece que el texto de buttonA
es Click!
.
En realidad no va a hacer nada.
Estilo del conjunto de widgets de etiquetas PyQt5 QLabel
El estilo del widget QLabel
de PyQt5, como el color de fondo, la familia de fuentes y el tamaño de las mismas, se puede establecer con el método setStyleSheet
. Funciona como una hoja de estilo en CSS.
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
Establece buttonA
con los siguientes estilos,
Estilo | Valor |
---|---|
background-color |
red |
font-size |
18px |
font-family |
Times New Roman |
Es conveniente establecer los estilos en PyQt5 porque es similar a CSS.
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
Evento de clic en la etiqueta PyQt5 QLabel
Label
El botón click event está conectado a alguna función específica con el método QLabel.clicked.connect(func)
.
import sys
from PyQt5 import QtWidgets
class Test(QtWidgets.QMainWindow):
def __init__(self):
QtWidgets.QMainWindow.__init__(self)
self.buttonA = QtWidgets.QPushButton("Click!", self)
self.buttonA.clicked.connect(self.clickCallback)
self.buttonA.move(100, 50)
self.labelA = QtWidgets.QLabel(self)
self.labelA.move(110, 100)
self.setGeometry(100, 100, 300, 200)
def clickCallback(self):
self.labelA.setText("Button is clicked")
if __name__ == "__main__":
app = QtWidgets.QApplication(sys.argv)
test = Test()
test.show()
sys.exit(app.exec_())
Cuando se hace clic en el QPushButton
buttonA
, se activa la función clickCallback
para establecer el texto de la etiqueta como Button is clicked
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook