Cómo comprobar si un archivo existe en Python
-
try...except
para comprobar la existencia del archivo (>Python 2.x) -
os.path.isfile()
para comprobar si el archivo existe (>=Python 2.x) -
pathlib.Path.is_file()
para comprobar si el archivo existe (>=Python 3.4)
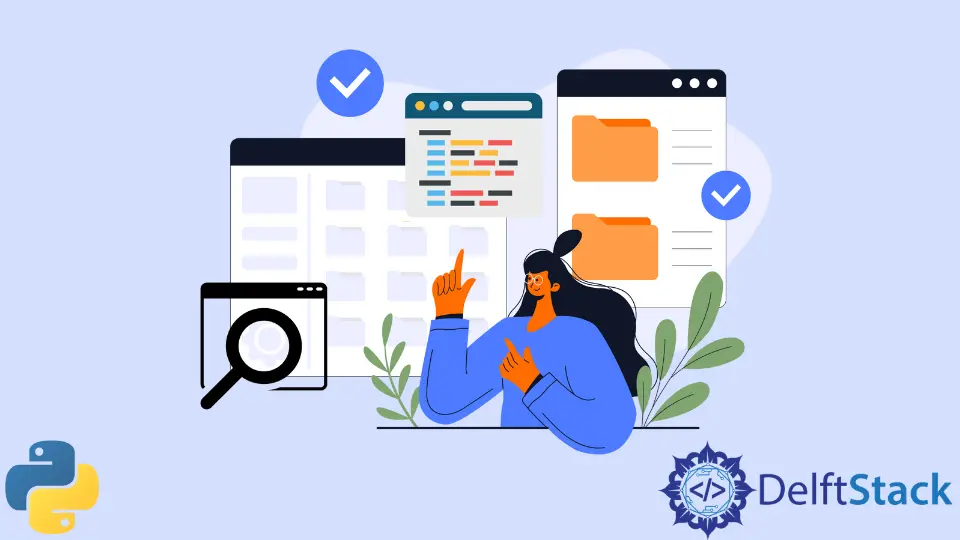
Este tutorial presentará tres soluciones diferentes para comprobar si un archivo existe en Python.
- Bloque
try...except
para comprobar la existencia del archivo (>=Python 2.x) - La función
os.path.isfile
para comprobar la existencia del archivo (>=Python 2.x) pathlib.Path.is_file()
comprobar la existencia del fichero (>=Python 3.4)
try...except
para comprobar la existencia del archivo (>Python 2.x)
Podríamos intentar abrir el archivo y comprobar si existe o no dependiendo de si se lanzará o no el IOError
(en Python 2.x) o FileNotFoundError
(en Python 3.x).
def checkFileExistance(filePath):
try:
with open(filePath, "r") as f:
return True
except FileNotFoundError as e:
return False
except IOError as e:
return False
En los códigos de ejemplo anteriores, lo hacemos compatible con Python 2/3 listando tanto FileNotFoundError
como IOError
en la parte de captura de excepciones.
os.path.isfile()
para comprobar si el archivo existe (>=Python 2.x)
import os
fileName = "temp.txt"
os.path.isfile(fileName)
Comprueba si el archivo fileName
existe.
Algunos desarrolladores prefieren usar os.path.exists()
para comprobar si un fichero existe. Pero no podría distinguir si el objeto es un fichero o un directorio.
import os
fileName = "temp.txt"
print(os.path.exists(fileName))
fileName = r"C:\Test"
print(os.path.exists(fileName))
Por lo tanto, sólo use os.path.isfile
si quiere comprobar si el archivo existe.
pathlib.Path.is_file()
para comprobar si el archivo existe (>=Python 3.4)
Desde Python 3.4, introduce un método orientado a objetos en el módulo pathlib
para comprobar si existe un archivo.
from pathlib import Path
fileName = "temp.txt"
fileObj = Path(fileName)
print(fileObj.is_file())
De forma similar, también tiene métodos is_dir()
y exists()
para comprobar si un directorio o un archivo/directorio existe.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook