Pandas DataFrame Eliminar índice
-
Eliminar el índice de un DataFrame de Pandas utilizando el método
reset_index()
-
Eliminar el índice de un DataFrame de Pandas usando el método
set_index()
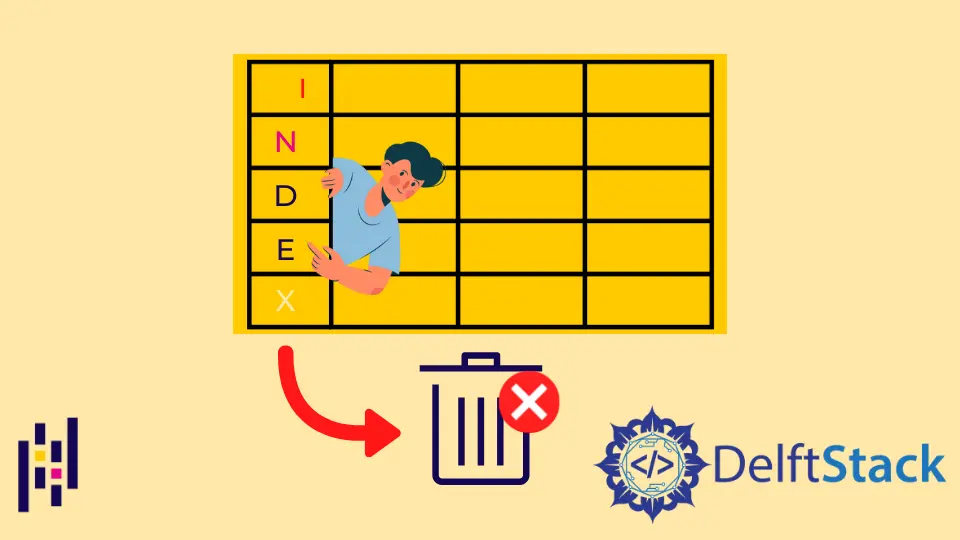
Este tutorial explicará cómo podemos eliminar el índice de Pandas DataFrame.
Utilizaremos el DataFrame que se muestra a continuación para mostrar cómo podemos eliminar el índice.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
print(my_df)
Producción :
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
Eliminar el índice de un DataFrame de Pandas utilizando el método reset_index()
El método pandas.DataFrame.reset_index()
restablecerá el índice del DataFrame al índice por defecto.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.reset_index()
print("Before reseting Index:")
print(my_df, "\n")
print("After reseting Index:")
print(df_reset)
Producción :
Before reseting Index:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After reseting Index:
index Person City Mother Tongue Age
0 A Alice Berlin German 37
1 B Steven Montreal French 20
2 C Neesham Toronto English 38
3 D Chris Rome Italian 23
4 E Alice Munich German 35
Restablecerá el índice del DataFrame my_df
pero el índice aparecerá ahora como la columna index
. Si queremos eliminar la columna index
, podemos establecer drop=True
en el método reset_index()
.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.reset_index(drop=True)
print("Before reseting Index:")
print(my_df, "\n")
print("After reseting Index:")
print(df_reset)
Producción :
Before reseting Index:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After reseting Index:
Person City Mother Tongue Age
0 Alice Berlin German 37
1 Steven Montreal French 20
2 Neesham Toronto English 38
3 Chris Rome Italian 23
4 Alice Munich German 35
Eliminar el índice de un DataFrame de Pandas usando el método set_index()
El método pandas.DataFrame.set_index()
establecerá la columna pasada como argumento como índice del DataFrame anulando el índice inicial.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.set_index("Person")
print("Initial DataFrame:")
print(my_df, "\n")
print("After setting Person column as Index:")
print(df_reset)
El resultado:
Initial DataFrame:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After setting Person column as Index:
City Mother Tongue Age
Person
Alice Berlin German 37
Steven Montreal French 20
Neesham Toronto English 38
Chris Rome Italian 23
Alice Munich German 35
Establece la columna Person
como índice del DataFrame my_df
anulando el índice inicial del DataFrame.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn