Borrar lienzo en JavaScript
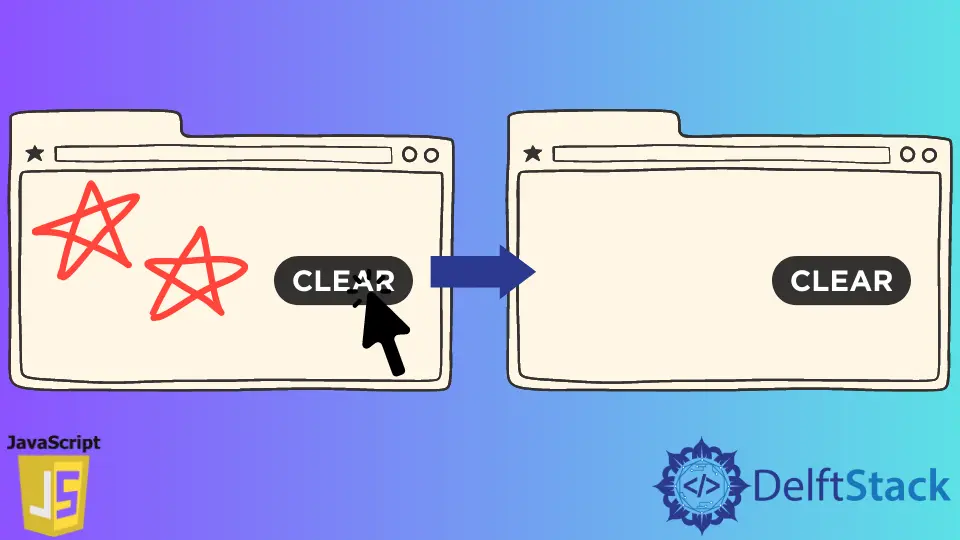
Usamos lienzo para dibujar gráficos. Proporciona múltiples métodos para dibujar, como círculos, cuadros, textos, agregar imágenes, etc. Necesitamos borrarlo y dibujar sobre él cuando usamos el lienzo.
Este artículo aborda cómo borrar un lienzo en JavaScript.
Borrar el lienzo en JavaScript
El elemento canvas
nos ayuda a dibujar gráficos con la ayuda de JavaScript. El lienzo
es solo un contenedor de gráficos y necesita JavaScript para dibujar los gráficos.
Podemos limpiar el lienzo usando el método clearRect()
de JavaScript. El elemento canvas
es compatible con todos los navegadores.
Solo tiene dos atributos: ancho y alto. Podemos personalizar el tamaño del lienzo usando las propiedades de ancho y alto de CSS.
Usamos un objeto de contexto de JavaScript que crea gráficos en el lienzo.
Creando Canvas usando JavaScript en HTML
Crearemos un lienzo con un color y tamaño específicos en el siguiente ejemplo.
Usamos la etiqueta canvas
con el id canvsId
y le dimos un tamaño personalizado al ancho y alto del lienzo. Usamos un script que crea un lienzo de color rojo, como se muestra en la salida.
Código:
<!DOCTYPE HTML>
<html>
<head>
<title>Creating a canvas</title>
</head>
<body>
<canvas id="canvsId" width="680" height="420"></canvas>
<script>
let canvas = document.getElementById('canvsId');
let context = canvas.getContext('2d');
context.beginPath();
context.moveTo(180, 90);
context.bezierCurveTo(120, 80, 110, 170, 250, 170);
context.bezierCurveTo(260, 190, 300, 160, 370, 140);
context.bezierCurveTo(410, 140, 440, 130, 380, 110);
context.bezierCurveTo(440, 50, 350, 40, 350, 50);
context.bezierCurveTo(310, 10, 270, 30, 240, 40);
context.bezierCurveTo(310, 10, 130, 15, 180, 90);
context.closePath();
context.lineWidth = 8;
context.strokeStyle = 'red';
context.stroke();
</script>
</body>
</html>
Producción:
Usaremos el clearRect()
en JavaScript si queremos borrar este lienzo que creamos. Este código se agregará al código de ejemplo anterior.
Código:
const context = canvas.getContext('2d');
context.clearRect(0, 0, canvas.width, canvas.height);
Usamos el código anterior para borrar el lienzo en el siguiente ejemplo. También creamos un botón llamado limpiar
, y le asignamos una función que, al hacer clic, limpiará el lienzo.
El lienzo que creamos se borrará cuando ejecutemos el código y hagamos clic en el botón borrar
.
Código completo:
<!DOCTYPE HTML>
<html>
<head>
<title>Title of the document</title>
<style>
#button {
position: absolute;
top: 5px;
left: 10px;
}
#button input {
padding: 10px;
display: block;
margin-top: 5px;
}
</style>
</head>
<body data-rsssl=1>
<canvas id="myCanvs" width="680" height="420"></canvas>
<div id="button">
<input type="button" id="clear" value="Clear">
</div>
<script>
let canvas = document.getElementById('myCanvs');
let context = canvas.getContext('2d');
context.beginPath();
context.moveTo(180, 90);
context.bezierCurveTo(120, 80, 110, 170, 250, 170);
context.bezierCurveTo(260, 190, 300, 160, 370, 140);
context.bezierCurveTo(410, 140, 440, 130, 380, 110);
context.bezierCurveTo(440, 50, 350, 40, 350, 50);
context.bezierCurveTo(310, 10, 270, 30, 240, 40);
context.bezierCurveTo(310, 10, 130, 15, 180, 90);
context.closePath();
context.lineWidth = 8;
context.strokeStyle = 'red';
context.stroke();
document.getElementById('clear').addEventListener('click', function() {
context.clearRect(0, 0, canvas.width, canvas.height);
}, false);
</script>
</body>
</html>
Producción:
Como puede ver en las imágenes de salida, se borra el lienzo cuando hacemos clic en el botón borrar
.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn