Crear LinkedList genérico en Java
-
una breve introducción a
LinkedList
en Java -
una implementación genérica de Singly
LinkedList
en Java
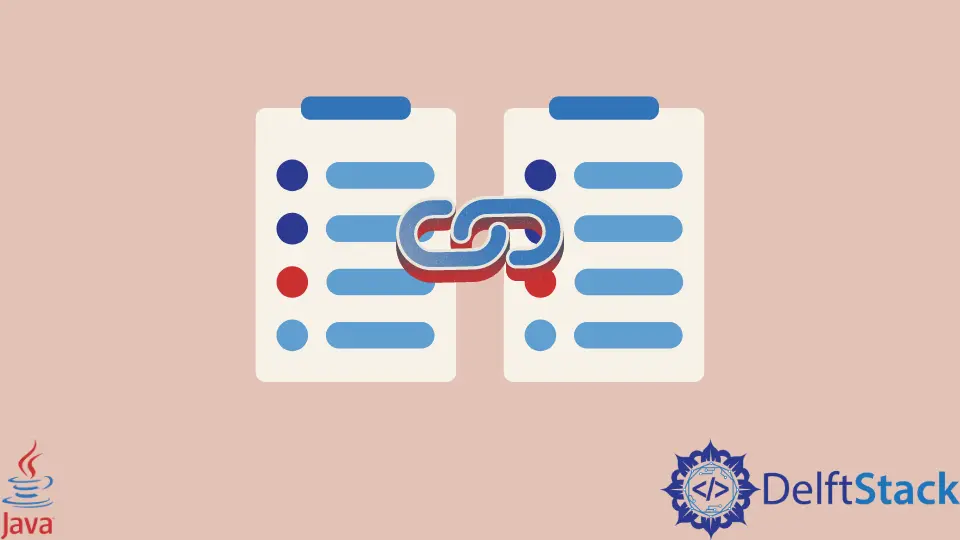
Este artículo nos enseñará cómo crear una Lista enlazada individual
genérica en Java.
una breve introducción a LinkedList
en Java
Las LinkedLists
son estructuras de datos lineales que almacenan datos en nodos en direcciones aleatorias y medios en ubicaciones no contiguas.
Cada nodo tiene dos partes: data
y referencia
(dirección). El campo Datos
/valor
almacena el valor, y el campo referencia
almacena la dirección del siguiente nodo de la lista enlazada.
Algunas de las operaciones comunes de esta estructura de datos que se implementarán como funciones miembro son las siguientes.
addNode()
: este método se usa para agregar un nuevo elemento al final de laLista enlazada
.removeNode(value)
: este método elimina el nodo con el valor especificado.addNode(position,value)
: este método se usa para agregar el valor en una posición específica.clear()
- Este método borra toda laLinkedList
.isEmpty()
: este método se utiliza para comprobar siLinkedList
está vacío o no.longitud()
- Este método nos da la longitud de laLinkedList
.
una implementación genérica de Singly LinkedList
en Java
Una Lista enlazada
normal solo puede almacenar un tipo de valor: entero, cadena, booleano, flotante, etc. Así que tenemos que especificar el tipo en el momento de la creación, pero ¿qué pasa si queremos crear la Lista enlazada
, que puede almacenar datos de cualquier tipo de datos; para ello utilizaremos el concepto de genéricos
en Java.
Creando una clase de nodo genérico:
En el siguiente código, T
denota el tipo de datos almacenados en LinkedList
.
class Node<T> {
T value;
Node<T> nextPtr;
Node(T value) {
this.value = value;
this.nextPtr = null;
}
}
Ahora vamos a crear los métodos genéricos de creación de la clase LinkedList
.
-
Función
añadir()
:void add(T value) { Node<T> temp = new Node<>(value); // creating a new node if (this.head == null) // checking if the list is empty head = temp; else // if the list is not empty, we go till the end and add the node { Node<T> tr = head; while (tr.nextPtr != null) { tr = tr.nextPtr; } tr.nextPtr = temp; } length = length + 1; // increasing the length of list }
-
Función
eliminar()
:void remove(T key) { Node<T> prev = new Node<>(null); prev.nextPtr = head; Node<T> next = head.nextPtr; Node<T> tr = head; boolean isNodepresent = false; // to check if node is present if (head.value == key) { head = head.nextPtr; isNodepresent = true; } while (tr.nextPtr != null) { if (String.valueOf(tr.value).equals( String.valueOf(key))) { // if the node is present, we break the loop prev.nextPtr = next; // we assign previous node's nextPtr to next node isNodepresent = true; break; } prev = tr; // updating the previous and next pointers tr = tr.nextPtr; next = tr.nextPtr; } if (isNodepresent == false && String.valueOf(tr.value).equals(String.valueOf(key))) { prev.nextPtr = null; isNodepresent = true; } if (isNodepresent) { length--; // if the node is present, we reduce the length } else { System.out.println("The value is not present inside the LinkedList"); } }
-
Función
añadir(posición,valor)
:void add(int position, T value) { if (position > length + 1) // if the position entered is more than the list length { System.out.println("Position out of bound"); return; } if (position == 1) { // if the position is one we'll just Node<T> temp = head; head = new Node<T>(value); head.nextPtr = temp; return; } Node<T> tr = head; Node<T> prev = new Node<T>(null); // creating a new node prev while (position - 1 > 0) // we find the position in the list { prev = tr; tr = tr.nextPtr; position--; } prev.nextPtr = new Node<T>(value); // update the next pointer of previous node prev.nextPtr.nextPtr = tr; }
-
Función
obtenerLongitud()
:int getLength() { return this.length; // returns the length of the list }
-
Función
estáVacío()
:boolean isEmpty() { if (head == null) // if the list is empty we return true return true; else return false; }
-
Función
clear()
:void clear() { head = null; // make head as null and length as zero length = 0; }
-
Función
toString()
:En el siguiente código, hemos agregado y anulado el método
toString
para imprimir el contenido deLinkedList
.@Override public String toString() { Node<T> temp = head; String str = "{ "; if (temp == null) // if the list is empty { System.out.println("list is empty"); } while (temp.nextPtr != null) // we keep appending data to string till the list is empty { str += String.valueOf(temp.value) + "->"; temp = temp.nextPtr; } str += String.valueOf(temp.value); return str + "}"; // we finally return the string }
Código de trabajo completo con la clase principal
:
class Node<T> {
T value;
Node<T> nextPtr;
Node(T value) {
this.value = value;
this.nextPtr = null;
}
}
class LinkedList<T>
{
Node<T> head;
private int length = 0;
LinkedList()
{
this.head = null;
}
void add(T value)
{
Node<T> temp = new Node<>(value);
if(this.head == null)
head = temp;
else
{
Node<T> tr = head;
while(tr.nextPtr!=null){
tr = tr.nextPtr;
}
tr.nextPtr = temp;
}
length = length + 1;
}
void remove(T key)
{
Node<T> prev = new Node<>(null);
prev.nextPtr = head;
Node<T> next = head.nextPtr;
Node<T> tr = head;
boolean isNodepresent = false;
if(head.value == key ){
head = head.nextPtr;
isNodepresent =true;
}
while(tr.nextPtr!=null)
{
if(String.valueOf(tr.value).equals(String.valueOf(key))){
prev.nextPtr = next;
isNodepresent = true;
break;
}
prev = tr;
tr = tr.nextPtr;
next = tr.nextPtr;
}
if(isNodepresent==false && String.valueOf(tr.value).equals(String.valueOf(key))){
prev.nextPtr = null;
isNodepresent = true;
}
if(isNodepresent)
{
length--;
}
else
{
System.out.println("The value is not present inside the LinkedList");
}
}
void add(int position,T value)
{
if(position>length+1)
{
System.out.println("Position out of bound");
return;
}
if(position==1){
Node<T> temp = head;
head = new Node<T>(value);
head.nextPtr = temp;
return;
}
Node<T> tr = head;
Node<T> prev = new Node<T>(null);
while(position-1>0)
{
prev = tr;
tr = tr.nextPtr;
position--;
}
prev.nextPtr = new Node<T>(value);
prev.nextPtr.nextPtr = tr;
}
int getLength()
{
return this.length;
}
boolean isEmpty()
{
if(head == null)
return true;
else
return false;
}
void clear()
{
head = null;
length = 0;
}
@Override
public String toString()
{
Node<T> temp = head;
String str = "{ ";
if(temp == null)
{
System.out.println( "list is empty");
}
while(temp.nextPtr!=null)
{
str += String.valueOf(temp.value) +"->";
temp = temp.nextPtr;
}
str += String.valueOf(temp.value);
return str+"}";
}
}
public class Example
{
public static void main(String[] args) {
LinkedList<Integer> ll = new LinkedList<>();
ll.add(1);
ll.add(2);
ll.add(3);
System.out.println(ll);
ll.remove(3);
System.out.println(ll);
ll.add(2,800);
System.out.println(ll);
}
}
Producción :
{ 1->2->3}
{ 1->2}
{ 1->800->2}