Formatear fecha y hora en C#
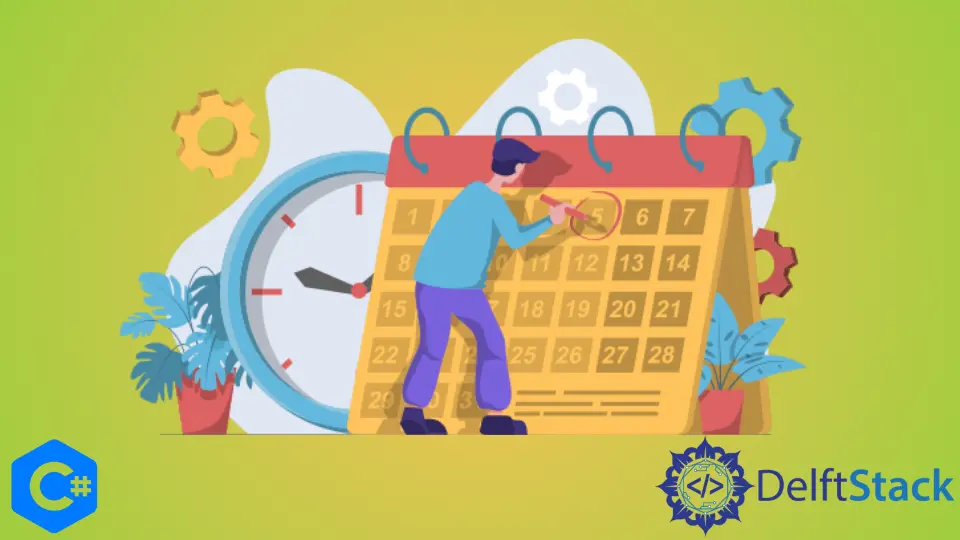
Este tutorial demostrará cómo crear una cadena formateada a partir de una variable DateTime
utilizando la función ToString()
o String.Format
.
Los especificadores de formato de fecha son la representación de cadena de los diferentes componentes en una variable DateTime
. Puede usarlos en las funciones ToString()
y String.Format
para especificar su propio formato DateTime
personalizado.
Estos son algunos especificadores de formato de fecha de uso común.
Fecha de muestra: 08/09/2021 3:25:10 PM
Especificadores de formato de fecha y hora personalizados:
Especificador de formato | Producción | Parte de fecha y hora |
---|---|---|
d |
8 |
Día |
dd |
08 |
Día |
M |
9 |
Mes |
MM |
09 |
Mes |
MMM |
Sep |
Mes |
MMMM |
September |
Mes |
yy |
21 |
Año |
yyyy |
2021 |
Año |
hh |
03 |
Hora |
HH |
15 |
Hora |
mm |
25 |
Minuto |
ss |
10 |
Segundo |
tt |
PM |
Tim |
Especificadores de formato de fecha y hora estándar:
especificador | Propiedad DateTimeFormatInfo | Valor del patrón |
---|---|---|
t |
ShortTimePattern |
h:mm tt |
d |
ShortDatePattern |
M/d/yyyy |
F |
FullDateTimePattern |
dddd, MMMM dd, yyyy h:mm:ss tt |
M |
MonthDayPattern |
MMMM dd |
Para obtener una lista más detallada y más ejemplos de especificadores de formato DateTime
, puede consultar la documentación oficial de Microsoft a continuación:
Formatear DateTime en C# usando ToString()
Formatear un DateTime
a una cadena es tan simple como aplicar ToString()
a DateTime
y proporcionar el formato que le gustaría usar.
DateTime.Now.ToString("ddMMyyyy") DateTime.Now.ToString("d")
Ejemplo:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = date.ToString("d");
// Format the datetime value to a custom format
string custom_fmt_date = date.ToString("ddMMyyyy");
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
Producción :
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021
Formatear DateTime en C# usando String.Format()
El formateo de un DateTime
a una cadena usando el método String.Format
se realiza proporcionando tanto el formato como el valor de DateTime
en los parámetros. El formato debe estar entre corchetes y con el prefijo "0:"
al imprimir el formato.
String.Format("{0:ddMMyyyy}", DateTime.Now) String.Format("{0:d}", DateTime.Now)
Ejemplo:
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
// Initialize the datetime value
DateTime date = new DateTime(2021, 12, 18, 16, 5, 7, 123);
// Format the datetime value to a standard format (ShortDatePattern)
string stanadard_fmt_date = String.Format("{0:d}", date);
// Format the datetime value to a custom format
string custom_fmt_date = String.Format("{0:ddMMyyyy}", date);
// Print the datetime formatted in the different ways
Console.WriteLine("Plain DateTime: " + date.ToString());
Console.WriteLine("Standard DateTime Format: " + stanadard_fmt_date);
Console.WriteLine("Custom DateTime Format: " + custom_fmt_date);
}
}
}
Producción :
Plain DateTime: 18/12/2021 4:05:07 pm
Standard DateTime Format: 18/12/2021
Custom DateTime Format: 18122021