Usar el temporizador en C++
-
Usar la función
clock()
para implementar un temporizador en C++ -
Usa la función
gettimeofday
para implementar un temporizador en C++
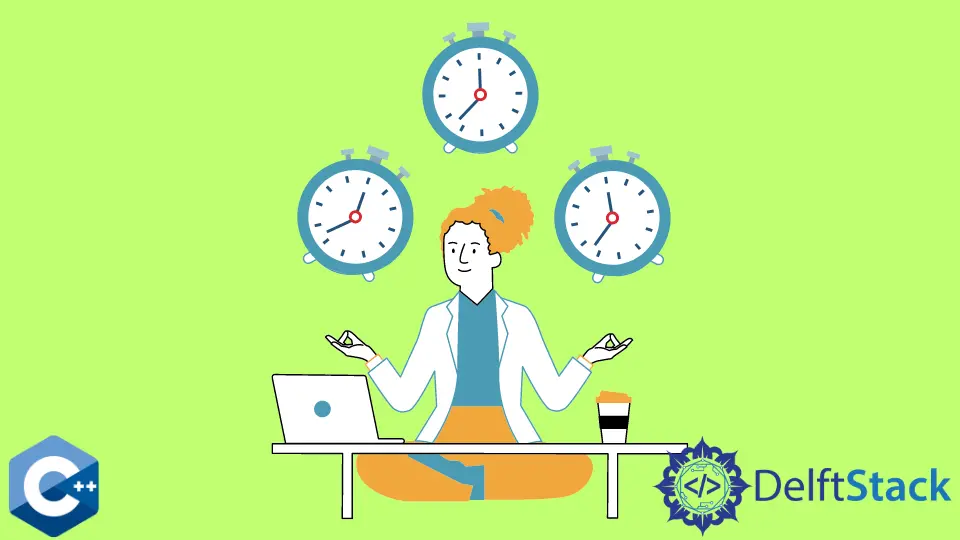
Este artículo demostrará múltiples métodos de cómo usar un temporizador en C++.
Usar la función clock()
para implementar un temporizador en C++
La función clock()
es un método compatible con POSIX para recuperar el tiempo del procesador del programa. La función devuelve el valor entero que necesita ser dividido por una constante definida por macro llamada CLOCKS_PER_SEC
para convertirlo en varios segundos.
El siguiente código de ejemplo implementa dos funciones para encontrar el valor máximo en un array int
. La función max_index
hace la búsqueda basada en el índice de los elementos, mientras que el max_value
se basa en el valor. El objetivo es calcular cuánto tiempo pasan para encontrar el máximo valor int
en la array de elementos 1,000,000
llena de números enteros aleatorios.
Fíjate que llamamos a la función clock()
dos veces - antes de la llamada max_index
y luego después de la llamada. Este esquema de medición del tiempo puede ser empleado generalmente sin tener en cuenta la función específica que recupera el tiempo. En este caso, podemos ver (dependiendo del sistema de hardware) que max_value
hace el trabajo ligeramente más rápido que la búsqueda basada en índices. Sin embargo, hay que tener en cuenta que estos algoritmos de búsqueda máxima tienen una complejidad O(N)
y no deben ser empleados en ningún código profesional excepto para la experimentación.
#include <chrono>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
constexpr int WIDTH = 1000000;
int main() {
clock_t start, end;
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
start = clock();
max = max_index(arr, WIDTH);
end = clock();
printf("max_index: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
start = clock();
max = max_value(arr, WIDTH);
end = clock();
printf("max_value: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
exit(EXIT_SUCCESS);
}
Producción :
max_value: 0.00131400 sec, max = 2147480499
max_value: 0.00089800 sec, max = 2147480499
Usa la función gettimeofday
para implementar un temporizador en C++
gettimeofday
es una función de recuperación de tiempo muy precisa en sistemas basados en Linux, que también puede ser llamada desde el código fuente de C++. La función fue diseñada para obtener los datos de la hora y la zona horaria, pero esta última ha sido depreciada durante algún tiempo, y el segundo argumento debería ser nullptr
en lugar de la struct
de la zona horaria válida. gettimeofday
almacena los datos de tiempo en una estructura especial llamada timeval
, que contiene dos miembros de datos tv_sec
que representan los segundos y tv_usec
para los microsegundos.
Como regla general, declaramos e inicializamos las dos estructuras timeval
antes de llamar a la función gettimeofday
. Una vez que la función es llamada, los datos deben ser almacenados con éxito en la correspondiente struct
si el valor de retorno de gettimeofday
es 0
. De lo contrario, el fallo se indica devolviendo el valor -1
. Observe que, después de que las structs se llenen con datos, necesita la conversión al valor de la unidad común del tiempo. Este código de muestra implementa la función time_diff
que devuelve el tiempo en segundos, el cual puede ser emitido a la consola según sea necesario.
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
constexpr int WIDTH = 1000000;
int main() {
struct timeval start {};
struct timeval end {};
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
gettimeofday(&start, nullptr);
max = max_index(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_index: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
gettimeofday(&start, nullptr);
max = max_value(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_value: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
exit(EXIT_SUCCESS);
}
Producción :
max_value: 0.00126000 sec, max = 2147474877
max_value: 0.00093900 sec, max = 2147474877
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook