MySQL ForEach-Schleife
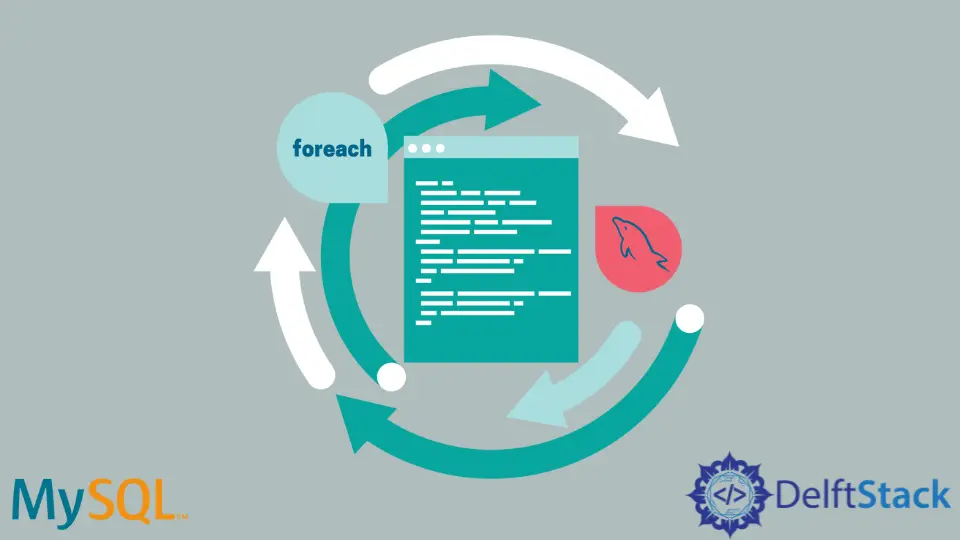
Dieses Tutorial zeigt, wie Sie die foreach
-Schleife in MySQL mit INSERT
, SELECT
, WHERE
und JOIN
in einer Anweisung simulieren.
MySQL-foreach
-Schleife
Um die Schleifensimulation foreach
zu verstehen, erstellen wir drei Tabellen, deren Namen und Attributnamen unten angegeben sind.
Tabellen und entsprechende Attribute:
users -> id, user_name, person_id, email
person -> id, person_name, address_id
address -> id, email
Wir verwenden die folgenden Abfragen, um die Tabellen zu erstellen.
# create `address`, `person`, and `users` tables
CREATE TABLE address(
id INT NOT NULL PRIMARY KEY AUTO_INCREMENT,
email VARCHAR(45) NULL
);
CREATE TABLE person (
id INT NOT NULL PRIMARY KEY AUTO_INCREMENT,
person_name VARCHAR(45) NOT NULL,
address_id INT NULL,
FOREIGN KEY (address_id) REFERENCES address(id)
);
CREATE TABLE users (
id INT NOT NULL PRIMARY KEY AUTO_INCREMENT,
user_name VARCHAR(45) NOT NULL,
person_id INT NOT NULL,
email VARCHAR(45) NOT NULL,
FOREIGN KEY (person_id) REFERENCES person(id)
);
Verwenden Sie außerdem die folgenden MySQL-Abfragen, um Daten in die gerade erstellten Tabellen einzufügen.
# insert data into the `address` table. Here
# we have parenthesis only because one field
# is `auto_incremented`, and the other can accept
# `null`.
INSERT INTO address () VALUES (),(),(),();
# insert data into the `person` table
INSERT INTO person (person_name)
VALUES
('Thomas Christopher'),
('Jim James'),
('Mehvish Ashiq'),
('Saira Daniel');
# insert data into the `users` table
INSERT INTO users(user_name, person_id, email)
VALUES
('chthomas', 1, 'chthomas@yahoo.com'),
('jjames', 2, 'jimjames@gmail.com'),
('mehvishashiq', 3, 'mehvish@yahoo.com'),
('danielsaira', 4, 'sairad@gmail.com');
Als nächstes verwenden wir die SELECT
-Anweisungen, um die jüngsten Daten zu betrachten.
# display data for all the specified tables
SELECT * FROM users;
SELECT * FROM person;
SELECT * FROM address;
Ausgabe (für die Tabelle users
):
+----+--------------+-----------+--------------------+
| id | user_name | person_id | email |
+----+--------------+-----------+--------------------+
| 1 | chthomas | 1 | chthomas@yahoo.com |
| 2 | jjames | 2 | jimjames@gmail.com |
| 3 | mehvishashiq | 3 | mehvish@yahoo.com |
| 4 | danielsaira | 4 | sairad@gmail.com |
+----+--------------+-----------+--------------------+
4 rows in set (0.00 sec)
Ausgabe (für die Tabelle person
):
+----+--------------------+------------+
| id | person_name | address_id |
+----+--------------------+------------+
| 1 | Thomas Christopher | NULL |
| 2 | Jim James | NULL |
| 3 | Mehvish Ashiq | NULL |
| 4 | Saira Daniel | NULL |
+----+--------------------+------------+
4 rows in set (0.00 sec)
Ausgabe (für die Tabelle Adresse
):
+----+-------+
| id | email |
+----+-------+
| 1 | NULL |
| 2 | NULL |
| 3 | NULL |
| 4 | NULL |
+----+-------+
4 rows in set (0.11 sec)
Wir sollen einen neuen Datensatz in der Tabelle address
erstellen, wenn users.person_id
NOT NULL
und person.address_id
NULL
ist. Die neu erstellte Zeile enthält die genaue E-Mail als users.email
.
Hier können wir die foreach
-Schleife auf folgende Weise simulieren.
# simulation of `foreach loop`
INSERT INTO address (email) SELECT users.email
FROM users JOIN person ON users.person_id = person.id
WHERE person.address_id IS NULL;
Verwenden Sie als Nächstes die Abfrage SELECT
, um die aktualisierten Daten anzuzeigen.
SELECT * FROM address;
Ausgang:
+----+--------------------+
| id | email |
+----+--------------------+
| 1 | NULL |
| 2 | NULL |
| 3 | NULL |
| 4 | NULL |
| 5 | chthomas@yahoo.com |
| 6 | jimjames@gmail.com |
| 7 | mehvish@yahoo.com |
| 8 | sairad@gmail.com |
+----+--------------------+
8 rows in set (0.00 sec)
Wir können die obige Abfrage aber auch mit cursor and procedure ausführen.