Konvertieren Sie Enum in Int in Java
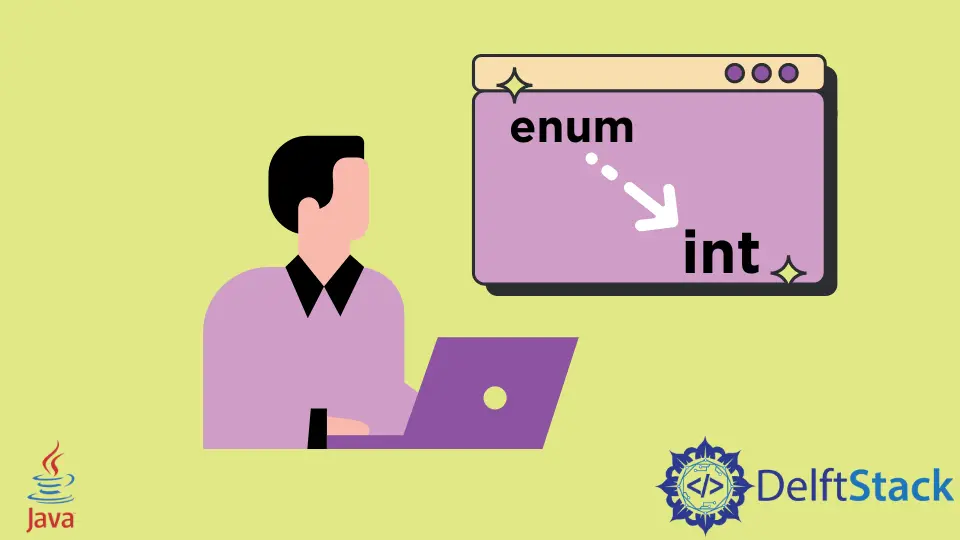
Dieses Tutorial zeigt, wie Sie Enum in Java in Int konvertieren.
Konvertieren Sie Enum in Int in Java
Java bietet keine integrierte Funktionalität zum Konvertieren von enum in int oder umgekehrt, aber wir können Methoden erstellen, um diese Operationen auszuführen. Wir können enum mit int-Werten mit der Methode value()
in int umwandeln.
Sehen wir uns den Schritt-für-Schritt-Prozess an:
-
Generieren Sie zunächst Ihr Enum mit int-Werten. In unserem Fall:
enum Cars { Toyota(10), Mercedes(20), Tesla(30), BMW(40), Audi(50); }
-
Erstellen Sie eine Methode, um die Enum-Werte
Cars
zu erhalten.public int getCarAsInt() { return cars; }
-
Erstellen Sie eine Methode, um das Aufzählungsmitglied
Cars
in int umzuwandeln. Diese Methode enthält einefor
-Schleife und eineif
-Bedingungsanweisung, die den ganzzahligen Wert zurückgibt.
```java
public static int convertCarToInt(Cars inputCar) {
for (Cars cars : Cars.values()) {
if (cars.getCarAsInt() == inputCar.getCarAsInt()) {
return cars.getCarAsInt();
}
}
return -1;
}
```
-
Wir können auch eine ähnliche Methode erstellen, um die int in die Aufzählung
Cars
umzuwandeln.public static Cars convertIntToCar(int intCars) { for (Cars cars : Cars.values()) { if (cars.getCarAsInt() == intCars) { return cars; } } return null; }
-
Wenden Sie schließlich die obigen Methoden auf jedes Enumerationsmitglied an. Lassen Sie uns ein Beispiel ausprobieren und jede Methode auf jedes Mitglied der Aufzählung
Cars
anwenden, wodurch die Aufzählungsmitglieder in int und int zurück in das Aufzählungsmitglied konvertiert werden:
```java
package delftstack;
enum Cars {
Toyota(10),
Mercedes(20),
Tesla(30),
BMW(40),
Audi(50);
private final int cars;
Cars(int cars) {
this.cars = cars;
}
public int getCarAsInt() {
return cars;
}
public static int convertCarToInt(Cars inputCar) {
for (Cars cars : Cars.values()) {
if (cars.getCarAsInt() == inputCar.getCarAsInt()) {
return cars.getCarAsInt();
}
}
return -1;
}
public static Cars convertIntToCar(int intCars) {
for (Cars cars : Cars.values()) {
if (cars.getCarAsInt() == intCars) {
return cars;
}
}
return null;
}
}
public class Example {
public static void main(String[] args) {
// For enum member Toyota
Cars Toyota_Car = Cars.Toyota;
int intCars = Toyota_Car.getCarAsInt();
// Get car as integer value
System.out.println("Get Toyota car as int value :" + intCars);
Toyota_Car = Cars.convertIntToCar(10);
// Convert integer value to corresponding car value
System.out.println("Convert integer 10 to car enum :" + Toyota_Car);
intCars = Cars.convertCarToInt(Cars.Toyota);
// Convert Cars value to corresponding integer value
System.out.println("Convert Toyota car to integer :" + intCars + "\n");
// For enum member Mercedes
Cars Mercedes_Car = Cars.Mercedes;
intCars = Mercedes_Car.getCarAsInt();
// Get car as integer value
System.out.println("Get Mercedes car as int value :" + intCars);
Mercedes_Car = Cars.convertIntToCar(20);
// Convert integer value to corresponding car value
System.out.println("Convert integer 10 to car enum :" + Mercedes_Car);
intCars = Cars.convertCarToInt(Cars.Mercedes);
// Convert Cars value to corresponding integer value
System.out.println("Convert Mercedes car to integer :" + intCars + "\n");
// For enum member Tesla
Cars Tesla_Car = Cars.Tesla;
intCars = Tesla_Car.getCarAsInt();
// Get car as integer value
System.out.println("Get Tesla car as int value :" + intCars);
Tesla_Car = Cars.convertIntToCar(30);
// Convert integer value to corresponding car value
System.out.println("Convert integer 10 to car enum :" + Tesla_Car);
intCars = Cars.convertCarToInt(Cars.Tesla);
// Convert Cars value to corresponding integer value
System.out.println("Convert Tesla car to integer :" + intCars + "\n");
// For enum member BMW
Cars BMW_Car = Cars.BMW;
intCars = BMW_Car.getCarAsInt();
// Get car as integer value
System.out.println("Get BMW car as int value :" + intCars);
BMW_Car = Cars.convertIntToCar(40);
// Convert integer value to corresponding car value
System.out.println("Convert integer 10 to car enum :" + BMW_Car);
intCars = Cars.convertCarToInt(Cars.BMW);
// Convert Cars value to corresponding integer value
System.out.println("Convert BMW car to integer :" + intCars + "\n");
// For enum member Audi
Cars Audi_Car = Cars.Audi;
intCars = Audi_Car.getCarAsInt();
// Get car as integer value
System.out.println("Get Audi car as int value :" + intCars);
Audi_Car = Cars.convertIntToCar(50);
// Convert integer value to corresponding car value
System.out.println("Convert integer 10 to car enum :" + Audi_Car);
intCars = Cars.convertCarToInt(Cars.Audi);
// Convert Cars value to corresponding integer value
System.out.println("Convert Audi car to integer :" + intCars + "\n");
}
}
```
<!--adsense-->
Der obige Code konvertiert jedes Enum-Element `Cars` in int und umgekehrt. Siehe die Ausgabe:
```text
Get Toyota car as int value :10
Convert integer 10 to car enum :Toyota
Convert Toyota car to integer :10
Get Mercedes car as int value :20
Convert integer 10 to car enum :Mercedes
Convert Mercedes car to integer :20
Get Tesla car as int value :30
Convert integer 10 to car enum :Tesla
Convert Tesla car to integer :30
Get BMW car as int value :40
Convert integer 10 to car enum :BMW
Convert BMW car to integer :40
Get Audi car as int value :50
Convert integer 10 to car enum :Audi
Convert Audi car to integer :50
```
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook