Wie man in C++ für Millisekunden schlafen kann
-
Verwendung von die Methode
std::this_thread::sleep_for
zum Einschlafen in C++ -
Verwendung von die Funktion
usleep
zum Einschlafen in C++ -
Verwendung von die Funktion
nanosleep
zum Einschlafen in C++
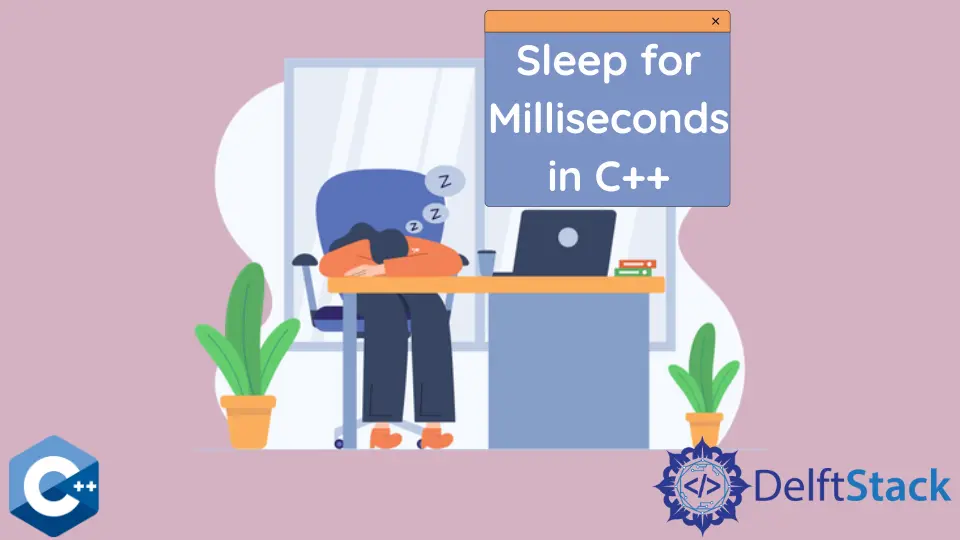
Dieser Artikel stellt Methoden zum Schlafen für Millisekunden in C++ vor.
Verwendung von die Methode std::this_thread::sleep_for
zum Einschlafen in C++
Diese Methode ist eine reine C++-Version der sleep
-Funktion aus der <thread>
-Bibliothek, und es ist die portable Version sowohl für Windows- als auch für Unix-Plattformen. Um ein besseres Beispiel zu demonstrieren, unterbrechen wir den Prozess für 3000 Millisekunden.
#include <chrono>
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(std::chrono::milliseconds(TIME_TO_SLEEP));
}
}
return 0;
}
Ausgabe:
Started loop...
Iteration - 0
Iteration - 1
Iteration - 2
Iteration - 3
Iteration - 4
Sleeping ....
Iteration - 5
Iteration - 6
Iteration - 7
Iteration - 8
Iteration - 9
Wir können den obigen Code mit einer eloquenteren Version umschreiben, indem wir den Namensraum std::chrono_literals
verwenden:
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
using namespace std::chrono_literals;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(3000ms);
}
}
return 0;
}
Verwendung von die Funktion usleep
zum Einschlafen in C++
usleep
ist eine POSIX-spezifische Funktion, die im Header <unistd.h>
definiert ist, die die Anzahl von Mikrosekunden als Argument akzeptiert, die vom Typ vorzeichenlose Ganzzahl sein sollte und in der Lage ist, Ganzzahlen im Bereich [0,1000000]
zu halten.
#include <unistd.h>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
usleep(TIME_TO_SLEEP * 1000);
;
}
}
return 0;
}
Alternativ können wir ein benutzerdefiniertes Makro mit der Funktion usleep
definieren und einen wiederverwendbaren Codeschnipsel erstellen.
#include <unistd.h>
#include <iostream>
#define SLEEP_MS(milsec) usleep(milsec * 1000)
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
SLEEP_MS(TIME_TO_SLEEP);
}
}
return 0;
}
Verwendung von die Funktion nanosleep
zum Einschlafen in C++
Die nanosleep
-Funktion ist eine weitere POSIX-spezifische Version, die eine bessere Behandlung von Interrupts bietet und eine feinere Auflösung des Schlafintervalls hat. Nämlich erzeugt ein Programmierer eine Timespec
-Struktur, um die Anzahl der Sekunden und Nanosekunden getrennt anzugeben. Nanosleep
nimmt auch den zweiten Strukturparameter timespec
, um das verbleibende Zeitintervall zurückzugeben, falls das Programm durch ein Signal unterbrochen wird. Beachten Sie, dass der Programmierer für die Behandlung von Unterbrechungen verantwortlich ist.
#include <ctime>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int SECS_TO_SLEEP = 3;
constexpr int NSEC_TO_SLEEP = 3;
int main() {
struct timespec request {
SECS_TO_SLEEP, NSEC_TO_SLEEP
}, remaining{SECS_TO_SLEEP, NSEC_TO_SLEEP};
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
nanosleep(&request, &remaining);
}
}
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook