Python sys.exit() Method
Vaibhav Vaibhav
Jan 30, 2023
Python
Python Sys
-
Syntax of Python
sys.exit()
Method -
Example Codes: Use
sys.exit(0)
Method for Successful Termination -
Example Codes: Use
sys.exit(None)
Method for Successful Termination - Example Codes: Failed Termination With a Non-zero Value
- Example Codes: Failed Termination With an Error Message
- Example Codes: Failed Termination With a Dictionary
- Example Codes: Failed Termination With a Class
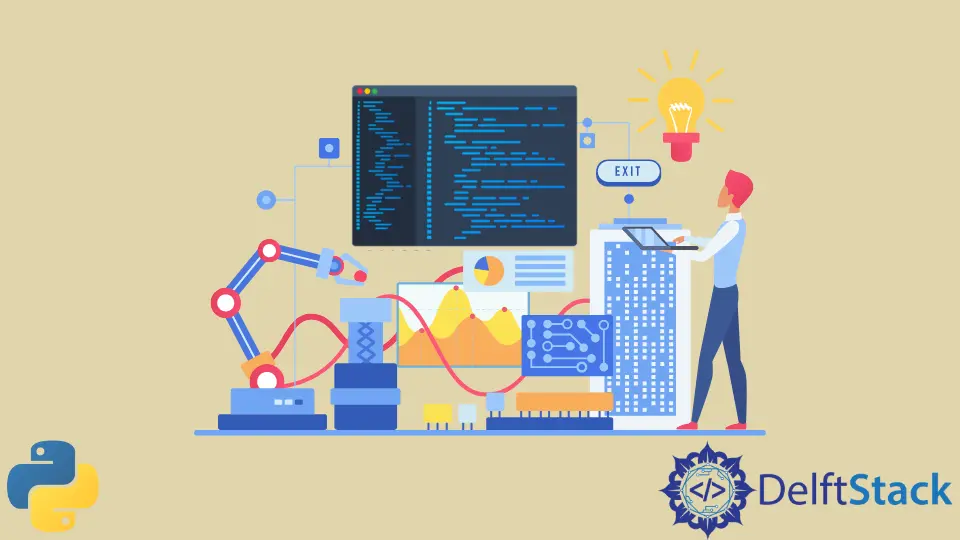
The sys
module deals with the Python interpreter, it is a built-in module available in Python. The sys.exit()
method allows us to stop the execution of a Python program, and it does so by raising a SystemExit
exception.
Syntax of Python sys.exit()
Method
sys.exit(arg)
Parameters
arg |
A parameter that defaults to 0 . 0 refers to a successful termination of the process, and any non-zero value relates to an abnormal or unsuccessful termination.Note that None is equivalent to giving a 0 , which relates to a successful termination. |
Returns
The exit()
method does not return anything.
Example Codes: Use sys.exit(0)
Method for Successful Termination
import sys
sys.exit(0)
Note that the default value is 0
, so passing a 0
is optional.
Example Codes: Use sys.exit(None)
Method for Successful Termination
import sys
sys.exit(None)
Example Codes: Failed Termination With a Non-zero Value
import sys
sys.exit(34)
This code throws an exception and will fail to terminate the program.
Example Codes: Failed Termination With an Error Message
import sys
sys.exit("ErrorName: This is an error message")
Output:
ErrorName: This is an error message
The exit()
function will print the exact string as an output.
Example Codes: Failed Termination With a Dictionary
import sys
sys.exit({"text": "Hello", "number": 25, "array": [1, 2, 3, 4, 5]})
Output:
{'text': 'Hello', 'number': 25, 'array': [1, 2, 3, 4, 5]}
process died unexpectedly: exit status 1
The exit()
function will print the dictionary.
Example Codes: Failed Termination With a Class
import sys
class Text:
def __init__(self, a, b, c):
self.a = a
self.b = b
self.c = c
def __repr__(self):
return f"Text({self.a}, {self.b}, {self.c})"
sys.exit(Text(1, 2, 3))
Output:
Text(1, 2, 3)
The exit()
function will print the string representation of the class object. If the class does not implement the __repr__()
method, the object’s memory address is printed.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Vaibhav Vaibhav