Python os.walk() Method
Imran Alam
Jan 30, 2023
Python
Python OS
-
Syntax of
os.walk()
: -
Example Codes: Use the
os.walk()
Method to Scan Directory -
Example Codes: Use the
os.walk()
Method With thetopdown
Parameter -
Example Codes: Use the
os.walk()
Method With theonerror
Parameter -
Example Codes: Use the
os.walk()
Method With thefollowlinks
Parameter
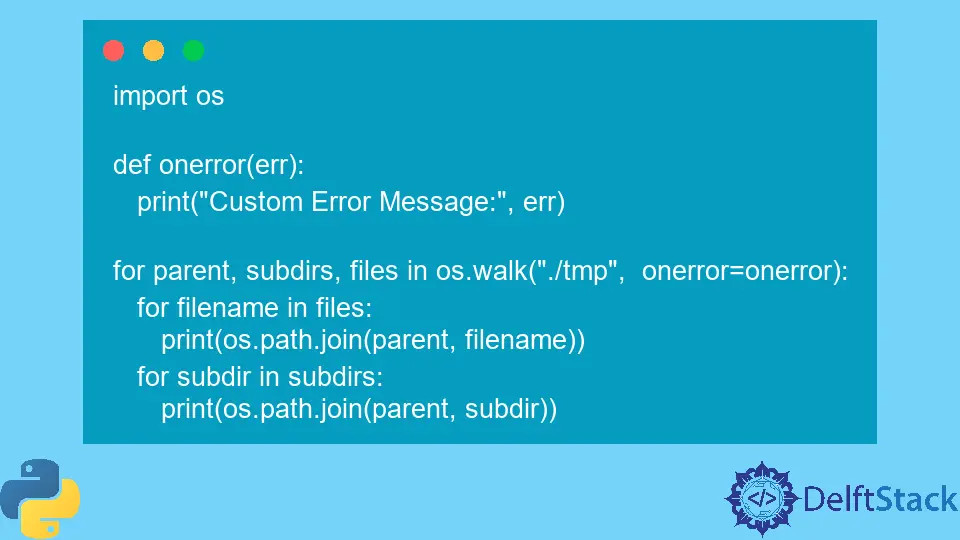
The os.walk()
method is a powerful tool that allows you to scan a directory for files and folders recursively.
Syntax of os.walk()
:
os.walk(path, topdown=True, onerror=None, followlinks=False)
Parameters
path |
This is the path to the directory that you want to scan. |
topdown |
This is a Boolean value that determines the order in which the directories are scanned. If set to True, the directories are scanned in top-down order, and if set to False, the directories are scanned in bottom-up order. |
onerror |
This function is called if an error occurs while scanning the directory. |
followlinks |
This is a Boolean value that determines whether symbolic links should be followed. |
Return
It returns a tuple containing the directory path, the directory names, and the file names.
Example Codes: Use the os.walk()
Method to Scan Directory
This example will scan the current directory for files and folders.
import os
for parent, subdirs, files in os.walk("."):
for filename in files:
print(os.path.join(parent, filename))
for subdir in subdirs:
print(os.path.join(parent, subdir))
Output:
.\[data.py](http://data.py/)
.\temp
.\temp\file1.txt
.\temp\file2.txt
.\temp\dir1
.\temp\dir2
Example Codes: Use the os.walk()
Method With the topdown
Parameter
This example will scan the current directory for files and folders in bottom-up order.
import os
for parent, subdirs, files in os.walk(".", topdown=False):
for filename in files:
print(os.path.join(parent, filename))
for subdir in subdirs:
print(os.path.join(parent, subdir))
Output:
.\temp\file1.txt
.\temp\file2.txt
.\temp\dir1
.\temp\dir2
.\[data.py](http://data.py/)
.\temp
Example Codes: Use the os.walk()
Method With the onerror
Parameter
In this example, we will scan the ./tmp
directory for files and folders. We will also print an error message if the directory does not exist.
import os
def onerror(err):
print("Custom Error Message:", err)
for parent, subdirs, files in os.walk("./tmp", onerror=onerror):
for filename in files:
print(os.path.join(parent, filename))
for subdir in subdirs:
print(os.path.join(parent, subdir))
Output:
Custom Error Message: [WinError 3] The system cannot find the path specified: './tmp'
To fix this error, you can create the /tmp
directory:
mkdir /tmp
Example Codes: Use the os.walk()
Method With the followlinks
Parameter
In this example, we will scan the current directory for files and folders and follow any symbolic links.
import os
for parent, subdirs, files in os.walk(".", followlinks=True):
for filename in files:
print(os.path.join(parent, filename))
for subdir in subdirs:
print(os.path.join(parent, subdir))
Output:
.\[data.py](http://data.py/)
.\temp
.\temp\file1.txt
.\temp\file2.txt
.\temp\dir1
.\temp\dir2
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe