Python os.read() Method
Imran Alam
Jan 30, 2023
-
Syntax of
os.read()
: -
Example Codes: Use the
os.read()
Method to Read a File -
Example Codes: Use the
os.read()
Method to Read the Entire File -
Example Codes: Use the
os.read()
Method to Read Input -
Example Codes: Possible Errors While Using the
os.read()
Method
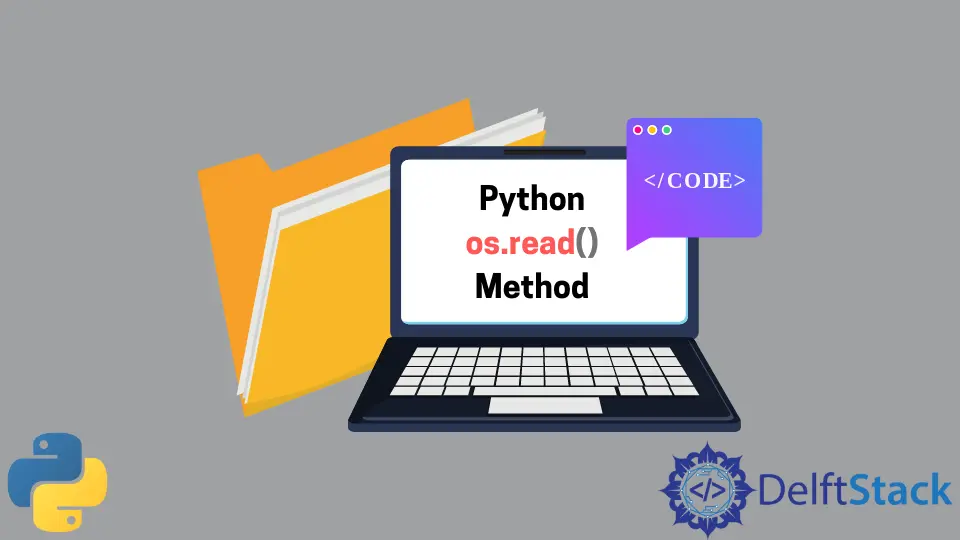
The os.read()
method is a built-in Python method used to read data from a file descriptor.
Syntax of os.read()
:
os.read(fd, n)
Parameters
fd |
This is the file descriptor to read from. |
n |
This is the number of bytes to read. |
Return
The os.read()
method returns the data read from the file descriptor as a string.
Example Codes: Use the os.read()
Method to Read a File
In the following example, the os.read()
method is used to read 10 bytes from the file descriptor:
import os
fd = os.open("test.txt", os.O_RDONLY)
data = os.read(fd, 10)
print(data)
Output:
This is
Example Codes: Use the os.read()
Method to Read the Entire File
In the following example, the os.read()
method is used to read the entire contents of the file:
import os
fd = os.open("test.txt", os.O_RDONLY)
data = os.read(fd, os.path.getsize("test.txt"))
print(data)
Output:
This is demo data
Example Codes: Use the os.read()
Method to Read Input
In the following example, the os.read()
method is used to read data from the standard input:
import os
data = os.read(0, 10)
print(data)
Input:
data
Output:
b'data\r\n'
Example Codes: Possible Errors While Using the os.read()
Method
Error | Description |
---|---|
ValueError |
This occurs if the fd is not a valid file descriptor. |
OSError |
This occurs if the fd is not open for reading. |
IOError |
This error occurs if the fd is not open. |
ValueError
import os
os.read(5, 10)
Output:
ValueError: 5 is not a valid file descriptor
OSError
import os
fd = os.open("test.txt", os.O_WRONLY)
os.read(fd, 10)
Output:
OSError: fd is not open for reading
IOError
import os
os.read(10, 10)
Output:
IOError: fd is not open