Python os.pipe() Method
-
Syntax of the
os.pipe()
Method -
Example Codes: Working With the
os.pipe()
Method in Python -
Example Codes: Understanding the
os.pipe()
Method in Python
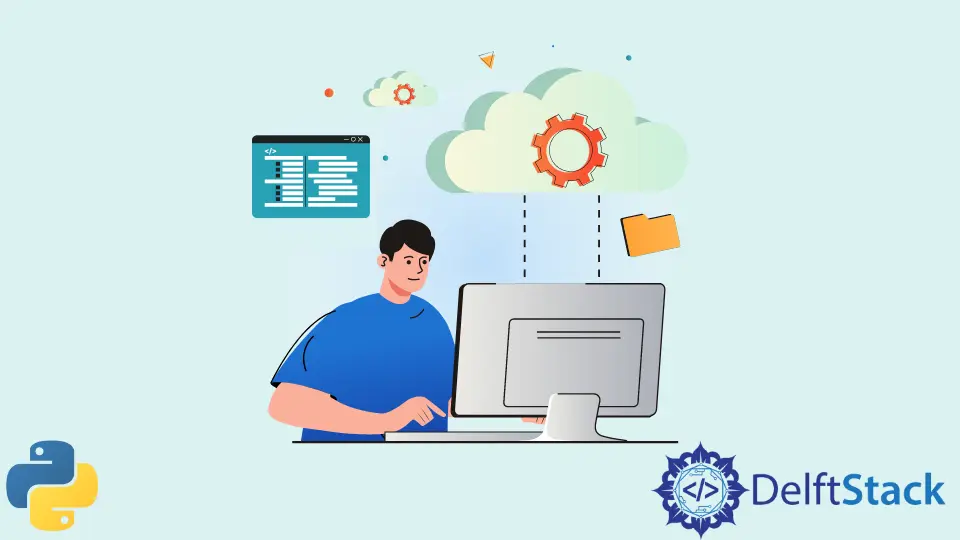
The os.pipe()
method is an efficient way of transferring information from one process to another through a pipe. The pipe is uni-directional, and the information passed is held by the OS until the intended process receives it.
Syntax of the os.pipe()
Method
os.pipe()
Parameters
This method does not take any parameters.
Return
This method returns a set of file descriptors (r, w) that can be used for reading and writing.
Example Codes: Working With the os.pipe()
Method in Python
import os
r, w = os.pipe()
pid = os.fork()
if pid > 0:
os.close(r)
print("Sending the following information: ")
text = b"Hello, World!"
os.write(w, text)
print("Written text:", text.decode())
else:
os.close(w)
print("\nThe intended process has received the information.")
Output:
Sending the following information:
Written text: Hello, World!
The intended process has received the information.
An OSError
might occur while using the os.pipe()
method due to invalid or inaccessible processes and paths.
Example Codes: Understanding the os.pipe()
Method in Python
import os
reading, writing = os.pipe()
pipe = os.fdopen(writing, "w")
pipe.write("Hello, world!")
pipe.close()
print("Received: ", os.fdopen(reading).read())
Output:
Received: Hello, world!
Note that the new file descriptors returned by the method os.pipe()
are non-inheritable.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn