Python os.path.islink() Method
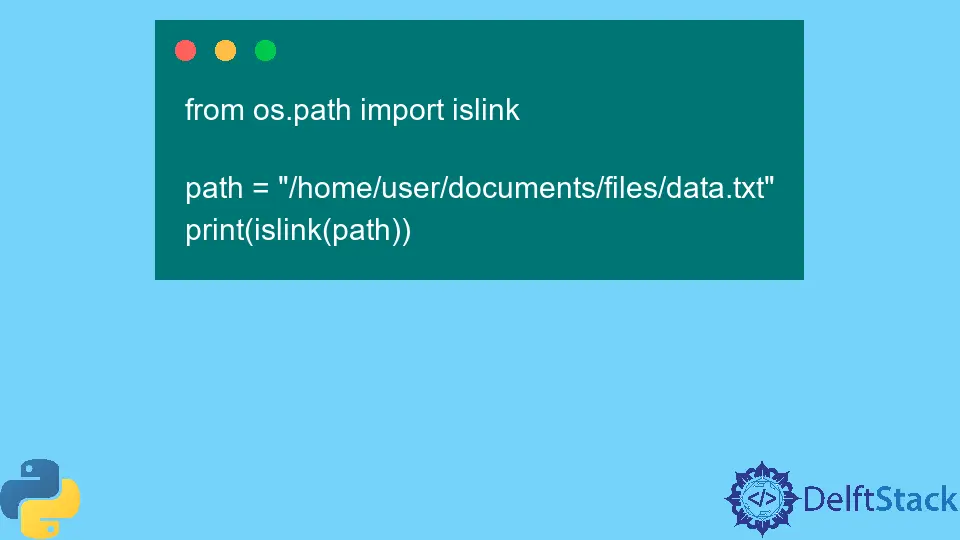
The islink()
method belongs to the os.path
module. The os
module is an in-built module available with Python that offers various procedures to interact with the operating system.
However, the path
module is a part of the os
module, which contains utilities to work with file system paths. The islink()
method checks if a path
is a symbolic link and leads to an existing resource.
Syntax
os.path.islink(path)
Parameters
Parameters | Type | Explanation |
---|---|---|
path |
string/bytes | A file system path. |
It also accepts an object (of type string or bytes) implementing the os.PathLike
protocol if we are using Python 3.6 or higher.
Returns
The islink()
method returns a Boolean
value. If the path
is a valid symbolic link, it returns True
. On the contrary, it returns False
for invalid paths.
Additionally, if the Python runtime environment does not support symbolic links, this method always returns False
.
Examples
Symbolic Link to a Resource
In Unix systems, we can create symbolic links or soft links using the ln
command. This command has the following syntax. To learn more about this command, refer to the official manual pages here.
ln [-sf] [source] [destination]
Create a text file, data.txt
, and add random data to it. Next, fire up the command line and run the following command to create a symbolic link for the file in the current directory.
ln -s 'data.txt' 'data-symlink.txt'
Now let’s understand how to use the islink()
method. First, refer to the following Python code.
from os.path import islink
path = "/home/user/documents/files/data-symlink.txt"
print(islink(path))
Output:
True
Since the path
is a symbolic link or the new file we just generated moments ago, the method returns True
.
Original Path to a Resource
from os.path import islink
path = "/home/user/documents/files/data.txt"
print(islink(path))
Output:
False
Since the path
stores address to the original resource, the islink()
method returns False
.