Python os.path.expanduser() Method
-
Syntax of
os.path.expanduser()
Method -
Example 1: Use the
os.path.expanduser()
Method in Python -
Example 2: Change the Environment on a Unix System in the
os.path.expanduser()
Method -
Example 3: Use the
os.path.expanduser()
Method on Windows OS -
Example 4: Use an Unspecified Environment Variable in the
os.path.expanduser()
Method
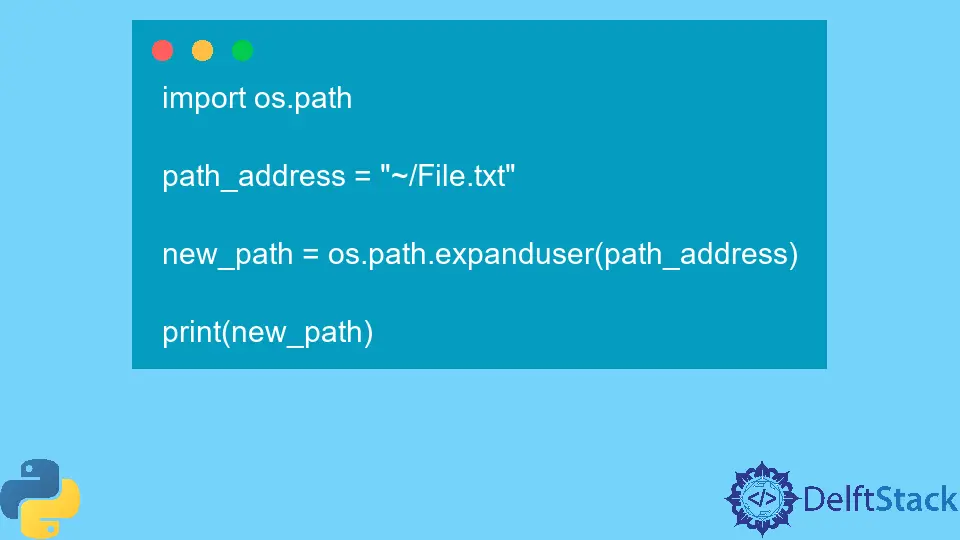
Python os.path.expanduser()
method is an efficient way of searching the home directory and expanding a defined path relative to it. The ~
(tilde symbol) extends the desired path, and the default is ~user
.
Syntax of os.path.expanduser()
Method
os.path.expanduser(path)
Parameters
path
- an address object of a file system path or a symlink. The object can either be an str
or bytes.
Return
The return type of this method is a string value representing the new path after expanding it, based on the input parameter.
Example 1: Use the os.path.expanduser()
Method in Python
import os.path
path_address = "~/File.txt"
new_path = os.path.expanduser(path_address)
print(new_path)
Output:
/File.txt
The os.path.expanduser()
works for any platform where a user has a home directory, such as Linux, Mac OS, and Windows.
Example 2: Change the Environment on a Unix System in the os.path.expanduser()
Method
import os.path
path_address = "~/File.txt"
os.environ["HOME"] = "/home / Documents"
new_path = os.path.expanduser(path_address)
print(new_path)
path_address = "~myfolder / File.txt"
new_path = os.path.expanduser(path_address)
print(new_path)
Output:
/home / Documents/File.txt
~myfolder / File.txt
On most Unix platforms, an initial ~
is replaced by the fixed value of the HOME
environment variable if it is set already.
Example 3: Use the os.path.expanduser()
Method on Windows OS
import os.path
path_address_1 = R"% HOME %\Documents\File.txt"
path_address_2 = R"C:\User\$USERNAME\HOME\File.txt"
path_address_3 = R"${temp}\File.txt"
new_path1 = os.path.expandvars(path_address_1)
new_path2 = os.path.expandvars(path_address_2)
new_path3 = os.path.expandvars(path_address_3)
print(new_path1)
print(new_path2)
print(new_path3)
Output:
% HOME %\Documents\File.txt
C:\User\$USERNAME\HOME\File.txt
${temp}\File.txt
On most Windows platforms, an initial ~
is replaced by the fixed value of HOME
and USERPROFILE
environment variable if it is set in advance.
Example 4: Use an Unspecified Environment Variable in the os.path.expanduser()
Method
import os.path
path_address = R"${MYHOME}/Documents / File.txt"
new_path = os.path.expandvars(path_address)
print(new_path)
Output:
${MYHOME}/Documents / File.txt
No environment variable is defined in the above code, so the path is unchanged and displayed as-is.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn