Python os.makedirs() Method
Imran Alam
Jan 30, 2023
Python
Python OS
-
Syntax of
os.makedirs()
: -
Example Codes: Use the
os.makedirs()
Method to Create a Directory -
Example Codes: Use the
os.makedirs()
Method to Create a Directory With Parents -
Example Codes: Use the
os.makedirs()
Method to Set the Mode of the Directory -
Example Codes: Possible Errors While Using the
os.makedirs()
Method
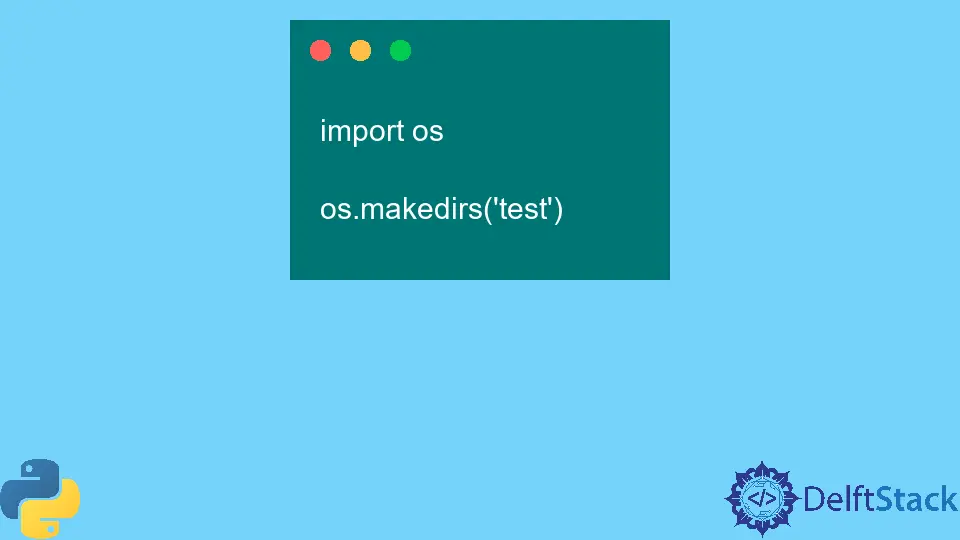
The os.makedirs()
method makes directories recursively. It creates all the intermediate directories needed to create the given directory.
Syntax of os.makedirs()
:
os.makedirs(path, mode=0o777, exist_ok=False)
Parameters
path |
This is the directory’s path to be created. |
mode |
This is the directory mode to be created. The default value is 0o777 (octal). |
exist_ok |
If set to True , it will not raise an error if the directory to be created already exists. The default value is False . |
Return
This method does not return any value.
Example Codes: Use the os.makedirs()
Method to Create a Directory
This example creates a directory named test
in the current directory.
import os
os.makedirs("test")
Example Codes: Use the os.makedirs()
Method to Create a Directory With Parents
This example creates a directory named test
in the current directory with its parents.
import os
os.makedirs("test/sub1/sub2")
Example Codes: Use the os.makedirs()
Method to Set the Mode of the Directory
This example creates a directory named test
in the current directory with the mode 0o755
.
import os
os.makedirs("test", mode=0o755)
Example Codes: Possible Errors While Using the os.makedirs()
Method
Error | Description |
---|---|
OSError |
This is raised when the given path is invalid. |
FileExistsError |
This is raised when the directory to be created already exists, and exist_ok is set to False . |
OSError
In this example, an invalid path is given, which results in an OSError
.
import os
os.makedirs("/test/sub1/sub2")
Output:
Traceback (most recent call last):
File "test.py", line 5, in <module>
os.makedirs('/test/sub1/sub2')
FileNotFoundError: [Errno 2] No such file or directory: '/test/sub1/sub2'
FileExistsError
In this example, the directory to be created already exists. Also, exist_ok
is set to False
, which results in a FileExistsError
.
import os
os.makedirs("test", exist_ok=False)
Output:
Traceback (most recent call last):
File "test.py", line 5, in <module>
os.makedirs('test', exist_ok=False)
FileExistsError: [Errno 17] File exists: 'test'
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe