Python os.dup2() Method
-
Syntax of Python
os.dup2()
: -
Example Code: Use the
os.dup2()
Method to Copy a File Descriptor to Another
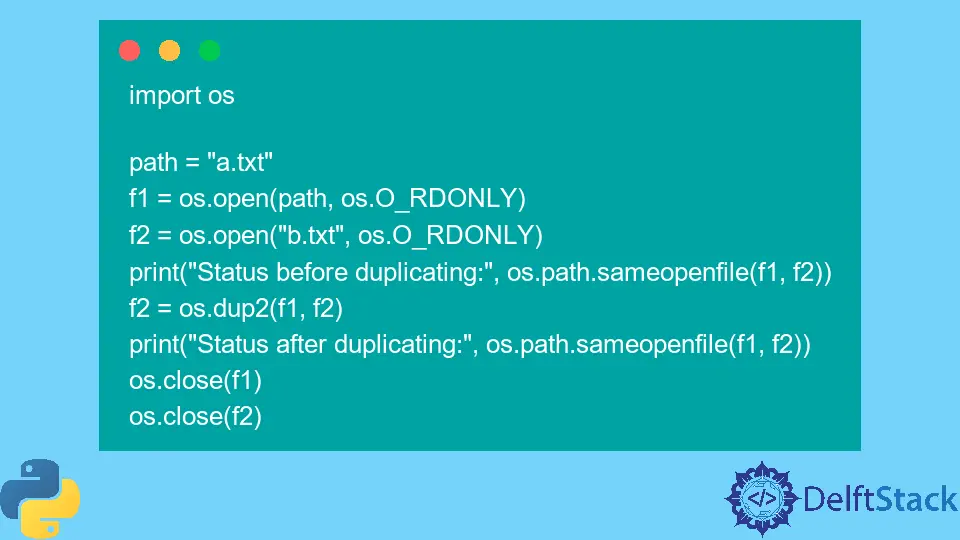
The dup2()
method belongs to the os
module. The os
module contains implementations for various functions to communicate and work with the operating system.
The dup2()
method copies a file descriptor to another and returns the new file descriptor.
Syntax of Python os.dup2()
:
os.dup2(fd, fd2, inheritable=True)
Parameters
Parameters | Type | Description |
---|---|---|
fd1 |
integer | A file descriptor associated with a valid resource. |
fd2 |
integer | A file descriptor associated with a valid resource. |
inheritable |
boolean | A flag to set the returned file descriptor as inheritable |
Return
The dup2()
method returns a file descriptor.
The fd2
file descriptor is closed, and the fd1
file descriptor is duplicated to the fd2
file descriptor, and based on the flag, fd2
is set to inheritable or not. The fd2
file descriptor is the returned object.
Example Code: Use the os.dup2()
Method to Copy a File Descriptor to Another
import os
path = "a.txt"
f1 = os.open(path, os.O_RDONLY)
f2 = os.open("b.txt", os.O_RDONLY)
print("Status before duplicating:", os.path.sameopenfile(f1, f2))
f2 = os.dup2(f1, f2)
print("Status after duplicating:", os.path.sameopenfile(f1, f2))
os.close(f1)
os.close(f2)
Output:
Status before duplicating: False
Status after duplicating: True
The Python code above first opened two different files. To prove that the two file descriptors are different, we used the sameopenfile()
method that returns a Boolean result and checks if the two file descriptors are the same or not.
Then, we copied the f1
file descriptor to f2
with the help of the dup2()
method. This method closes the b.txt
file and duplicates f1
to f2
.
Next, the sameopenfile()
method proves that both the file descriptors point to the same resource. Lastly, both file descriptors are closed.