Python os.chmod() Method
-
Syntax of the
os.chmod()
Method -
Example Codes: Working With the
os.chmod()
Method in Python -
Example Codes: Set Permission to an Octal Number Using the
os.chmod()
Method in Python
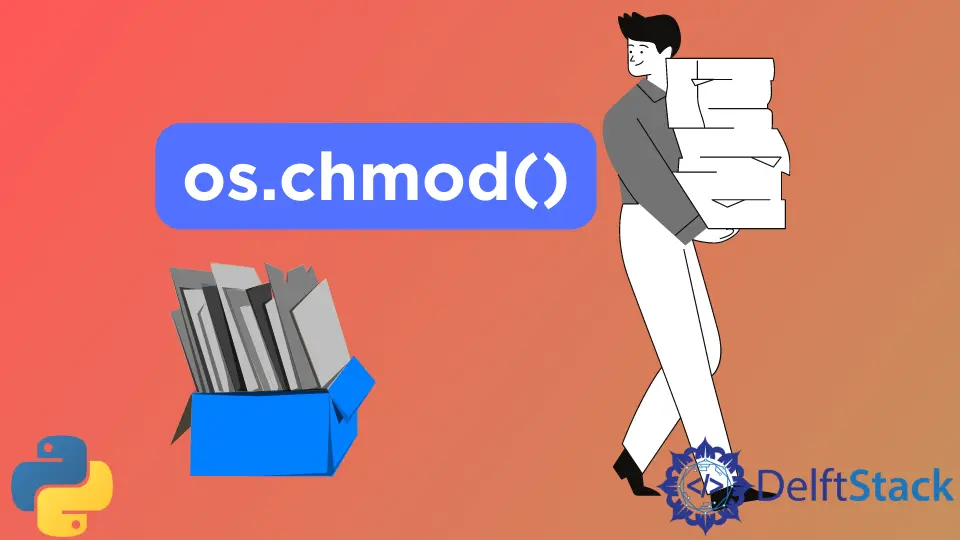
Python os.chmod()
method is an efficient way of changing the permission of a file/directory to the specified mode entered as a parameter.
Syntax of the os.chmod()
Method
os.chmod(path address, mode)
Parameters
path address |
It is a file system path address object or a symlink. The object can either be str or bytes. |
mode |
The mode can take one of the following values, or if you want a combination of some modes, you can use bitwise OR . |
1. stat.S_ISUID : This mode, when executed, sets the path’s mode to the user ID. |
|
2. stat.S_ISGID : This mode, when executed, sets the path’s mode to the group ID. |
|
3. stat.S_ISVTX : When set on a directory, this mode only allows the file owner to rename or delete it. |
|
4. stat.S_IRWXU : This mode allows the file owner to read, write and execute the file. |
|
5. stat.S_IREAD : This mode only allows the file owner to read it. It is synonymous with stat.s_IRUSR . |
|
6. stat.S_IWRITE : This mode only allows the file owner to write it. It is synonymous with stat.s_IWUSR . |
|
7. stat.S_IEXEC : This mode only allows the file owner to execute it. It is synonymous with stat.s_IXUSR . |
|
8. stat.S_IRUSR : This mode only allows the file owner to read it. It is synonymous with stat.s_IREAD. |
|
9. stat.S_IWUSR : This mode only allows the file owner to write it. It is synonymous with stat.s_IWRITE . |
|
10. stat.S_IXUSR : This mode only allows the file owner to execute it. It is synonymous with stat.s_IEXEC . |
|
11. stat.S_IRWXG : This mode allows a group of users to read, write and execute the file. |
|
12. stat.S_IRGRP : This mode only allows a group of users to read it. |
|
13. stat.S_IWGRP : This mode only allows a group of users to write it. |
|
14. stat.S_IXGRP : This mode only allows a group of users to execute it. |
|
15. stat.S_IRWXO : This mode allows special access to others, exclusive to the group, to read, write and execute the file. |
|
16. stat.S_IROTH : This mode allows special access to others, exclusive to the group, to read it only. |
|
17. stat.S_IWOTH : This mode allows special access to others, exclusive to the group, to write it only. |
|
18. stat.S_IXOTH : This mode allows special access to others, exclusive to the group, to execute it. |
|
19. stat.S_ENFMT : This mode acts as file locking enforcement. |
Return
In the execution process, this method does not return any value.
Example Codes: Working With the os.chmod()
Method in Python
import os
import sys
import stat
os.chmod("/home/file.txt", stat.S_IREAD)
print("Now, this file can be read only by the owner.")
os.chmod("/home/file.txt", stat.S_IRGRP)
print("Now, this file can be read only by a group of users.")
os.chmod("/home/file.txt", stat.S_IROTH)
print("The file access has changed; now it can be read by others.")
Output:
Now, this file can be read only by the owner.
Now, this file can be read only by a group of users.
The file access has changed; now it can be read by others.
This method can work on a specified file descriptor, an absolute or a relative path, and it might work on a symlink on some systems.
Example Codes: Set Permission to an Octal Number Using the os.chmod()
Method in Python
import os
file_name = "file.txt"
os.chmod(file_name, 0o777)
stat = os.stat(file_name)
mode = oct(stat.st_mode)[-3:]
print(mode)
Output:
777
When using the os.chmod()
method, write 0o
before the permissions to set because they represent an octal integer. In the above code, the file’s permission is set to 777
, meaning anyone can do anything they want with the file.
Find more numeric notations here
.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn