Python math.exp() Method
-
Syntax of Python
math.exp()
Method -
Example Codes: Use the
math.exp()
Method in Python -
Example Codes: Code Demonstrates an Error of the
math.exp()
Method -
Example Codes: The
math.exp()
Method and Its Inverse -
Example Codes: The
math.exp()
Method and Its Alternative Methods
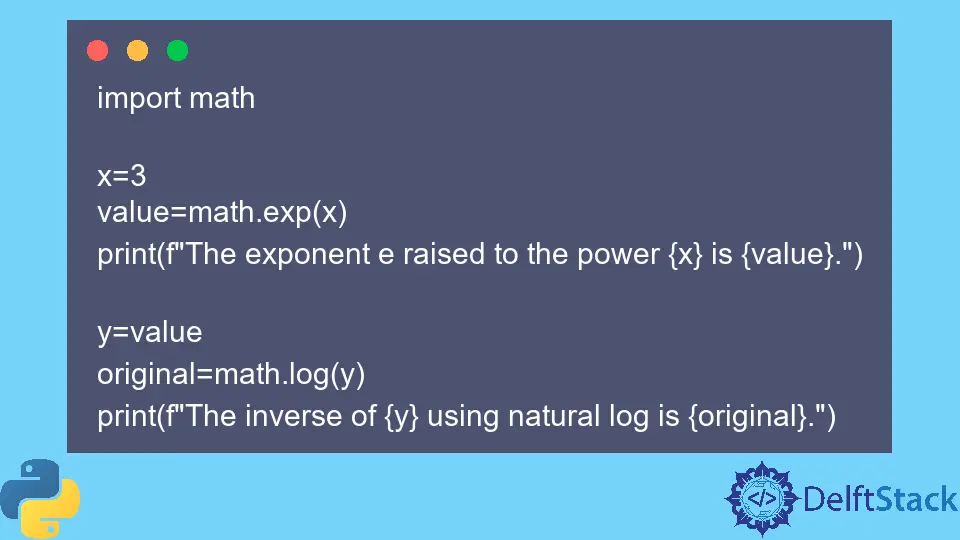
Python math.exp()
method is an efficient way of calculating the exponential value with the base set to e
.
Syntax of Python math.exp()
Method
math.exp(x)
Parameters
x |
Any positive or negative number specifying the exponent. |
Return
The return type of this method is a float value representing an exponential of x: e^x
.
Example Codes: Use the math.exp()
Method in Python
import math
x = -30.9
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
x = 0
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
x = 6
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
x = math.inf
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
Output:
The exponent e raised to the power -30.9 is 3.80452558642217e-14.
The exponent e raised to the power 0 is 1.0.
The exponent e raised to the power 6 is 403.4287934927351.
The exponent e raised to the power inf is inf.
Note that the values may be either positive or negative. These methods are used in mathematical computations related to geometry and have a certain application in astronomical computations.
Example Codes: Code Demonstrates an Error of the math.exp()
Method
import math
# enter a string
x = "Hi"
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
# enter a list
x = [1, 2, 3]
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
# enter complex numbers
x = 1 + 5j
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.exp(x)
TypeError: must be real number, not str
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.exp(x)
TypeError: must be real number, not list
Traceback (most recent call last):
File "main.py", line 6, in <module>
value=math.exp(x)
TypeError: can't convert complex to float
Note that e
is a math’s constant having a value approximately equal to 2.71828
.
Example Codes: The math.exp()
Method and Its Inverse
import math
x = 3
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
y = value
original = math.log(y)
print(f"The inverse of {y} using natural log is {original}.")
Output:
The exponent e raised to the power 3 is 20.085536923187668.
The inverse of 20.085536923187668 using natural log is 3.0.
Note that when one argument is entered in the math.log()
method, the natural logarithm of x (to base e) is automatically returned.
Example Codes: The math.exp()
Method and Its Alternative Methods
import math
x = 1
value = math.exp(x)
print(f"The exponent e raised to the power {x} is {value}.")
x = 1
value = math.e ** x
print(f"The exponent e raised to the power {x} is {value}.")
x = 1
value = pow(math.e, x)
print(f"The exponent e raised to the power {x} is {value}.")
Output:
The exponent e raised to the power 1 is 2.718281828459045.
The exponent e raised to the power 1 is 2.718281828459045.
The exponent e raised to the power 1 is 2.718281828459045.
Any of the above methods can be used to find the exponent of a number.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn