Python math.cosh() Method
Musfirah Waseem
Sep 16, 2022
Python
Python Math
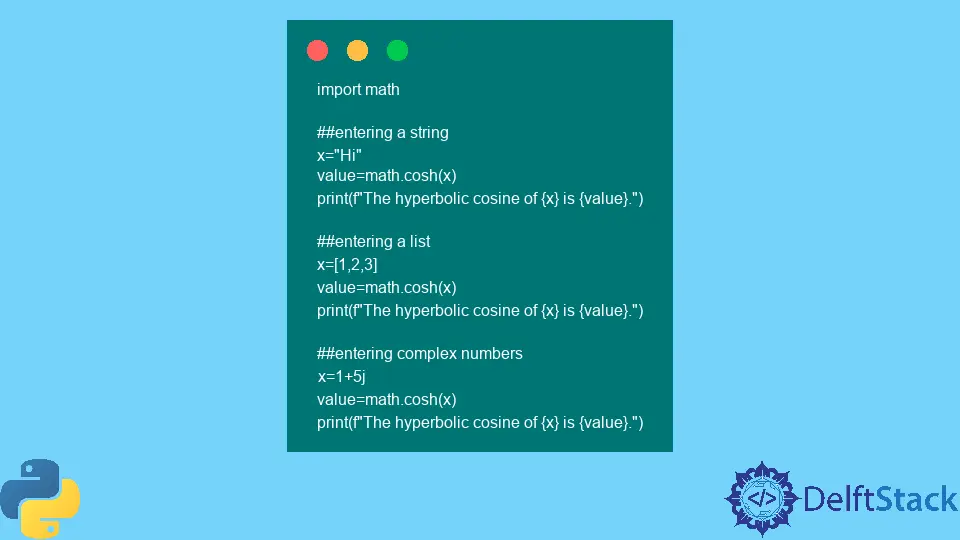
Python math.cosh()
method is an efficient way of calculating the hyperbolic cosine of a number, x
, in radians.
Syntax
math.cosh(x)
Parameters
x |
Any positive or negative value. |
Returns
This method’s return type is a float
value representing the hyperbolic cosine of x
.
Examples
Use Python math.cosh()
Method to Get Hyperbolic Cosine of x
in Radians
Code Snippet:
import math
x = -0.9
value = math.cosh(x)
print(f"The hyperbolic cosine of {x} is {value}.")
x = 0
value = math.cosh(x)
print(f"The hyperbolic cosine of {x} is {value}.")
x = 0.0086
value = math.cosh(x)
print(f"The hyperbolic cosine of {x} is {value}.")
x = math.inf
value = math.cosh(x)
print(f"The hyperbolic cosine of {x} is {value}.")
Output:
The hyperbolic cosine of -0.9 is 1.4330863854487745.
The hyperbolic cosine of 0 is 1.0.
The hyperbolic cosine of 0.0086 is 1.0000369802279205.
The hyperbolic cosine of inf is inf.
Note that the parameter should be a valid number. The values may be either positive or negative.
TypeError
Using Python math.cosh()
Method
Code Snippet:
import math
# entering a string
x = "Hi"
value = math.cosh(x)
print(f"The hyperbolic cosine of {x} is {value}.")
# entering a list
x = [1, 2, 3]
value = math.cosh(x)
print(f"The hyperbolic cosine of {x} is {value}.")
# entering complex numbers
x = 1 + 5j
value = math.cosh(x)
print(f"The hyperbolic cosine of {x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.cosh(x)
TypeError: must be a real number, not str
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.cosh(x)
TypeError: must be a real number, not a list
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.cosh(x)
TypeError: can't convert complex to float
The above code snippets show all possible syntactic errors when using the math.cosh
method.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn