Python math.cos() Method
Musfirah Waseem
Sep 16, 2022
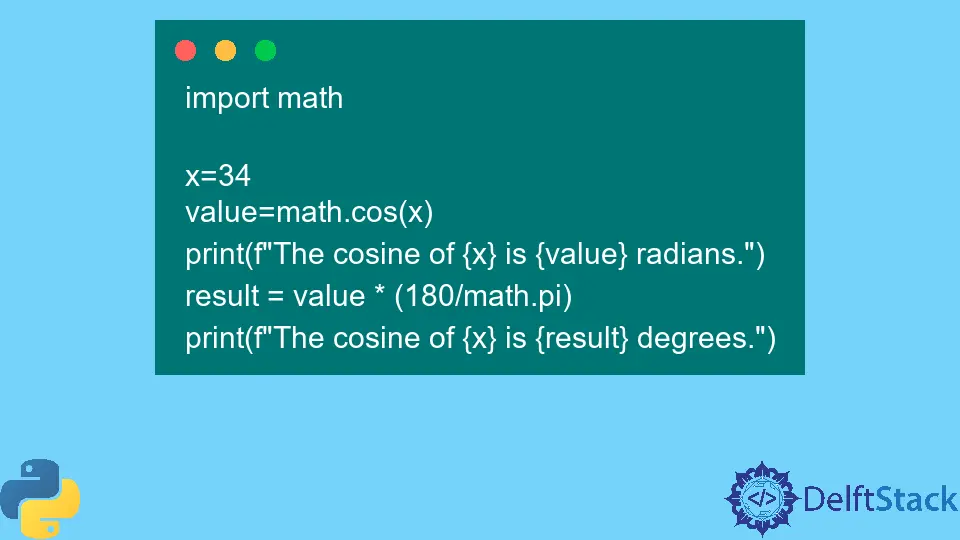
Python math.cos()
method is an efficient way of calculating the cosine of a number, x
, in radians.
Syntax
math.cos(x)
Parameters
x |
Any positive or negative value. |
Returns
This method’s return type is a float
value representing the cosine of x
in radians.
Examples
Use Python math.cos()
Method to Get Cosine of x
in Radians
Code Snippet:
import math
x = math.pi / 6
value = math.cos(x)
print(f"The cosine of {x} is {value}.")
x = 0
value = math.cos(x)
print(f"The cosine of {x} is {value}.")
x = -2.349593486
value = math.cos(x)
print(f"The cosine of {x} is {value}.")
Output:
The cosine of 0.5235987755982988 is 0.8660254037844387.
The cosine of 0 is 1.0.
The cosine of -2.349593486 is -0.7024237948400399.
Note that the parameter can be an integer value (int
) or a floating point number (float
). In addition, the values may be either positive or negative.
Use Python math.cos()
Method to Get Cosine of x
in Radians & Degrees
Code Snippet:
import math
x = 34
value = math.cos(x)
print(f"The cosine of {x} is {value} radians.")
result = value * (180 / math.pi)
print(f"The cosine of {x} is {result} degrees.")
Output:
The cosine of 34 is -0.8485702747846052 radians.
The cosine of 34 is -48.61949536541442 degrees.
Note that the output values of this method will always be in the range of -1
and 1
.
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn