Python math.ceil() Method
Musfirah Waseem
Sep 16, 2022
Python
Python Math
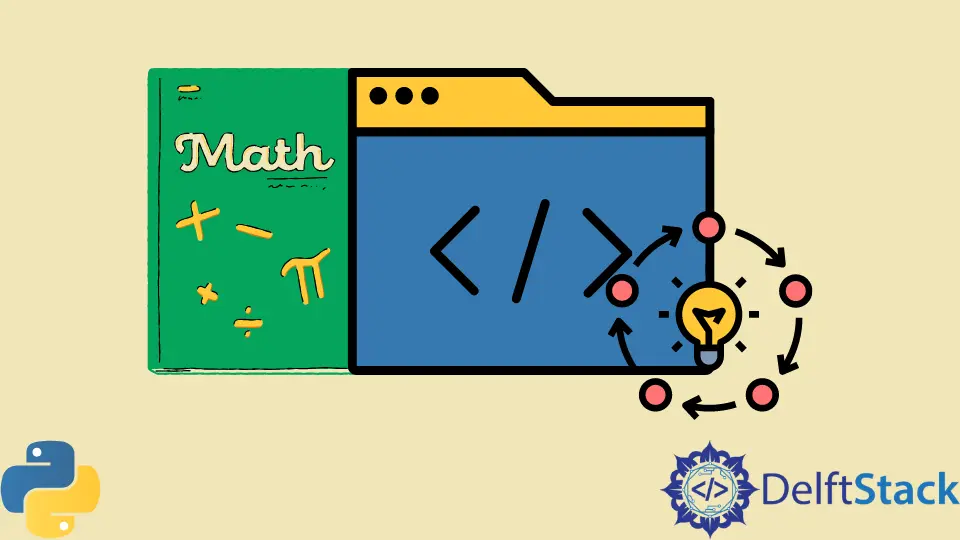
Python math.ceil()
method is an efficient way of rounding off a number to its smallest integral value greater than the given number. The exact number is returned if the given number is already an integer.
Syntax
math.ceil(x)
Parameters
x |
Any positive or negative number. |
Returns
The return type of this method is an integer representing the ceiling of x
.
Examples
Example Demonstrating Correct Use of math.ceil()
Code Snippet:
import math
x = 0.898762343
value = math.ceil(x)
print(f"The ceiling of {x} is {value}.")
x = -9.5555555
value = math.ceil(x)
print(f"The ceiling of {x} is {value}.")
x = 10
value = math.ceil(x)
print(f"The ceiling of {x} is {value}.")
Output:
The ceiling of 0.898762343 is 1.
The ceiling of -9.5555555 is -9.
The ceiling of 10 is 10.
Note that the parameter should be a valid number. The values may be either positive or negative.
Example Demonstrating an Error While Using math.ceil()
Code Snippet:
import math
# entering a string
x = "Hi"
value = math.ceil(x)
print(f"The ceiling of {x} is {value}.")
# entering a list
x = [1, 2, 3]
value = math.ceil(x)
print(f"The ceiling of {x} is {value}.")
# entering complex numbers
x = 1 + 5j
value = math.ceil(x)
print(f"The ceiling of {x} is {value}.")
# entering an infinite number
x = math.inf
value = math.ceil(x)
print(f"The ceiling of {x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.ceil(x)
TypeError: must be a real number, not str
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.ceil(x)
TypeError: must be a real number, not a list
^ATraceback (most recent call last):
File "main.py", line 7, in <module>
value=math.ceil(x)
TypeError: can't convert complex to float
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.ceil(x)
OverflowError: cannot convert float infinity to integer
The above code snippets show all possible syntactic errors when using the math.ceil()
method.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn