Python math.atan2() Method
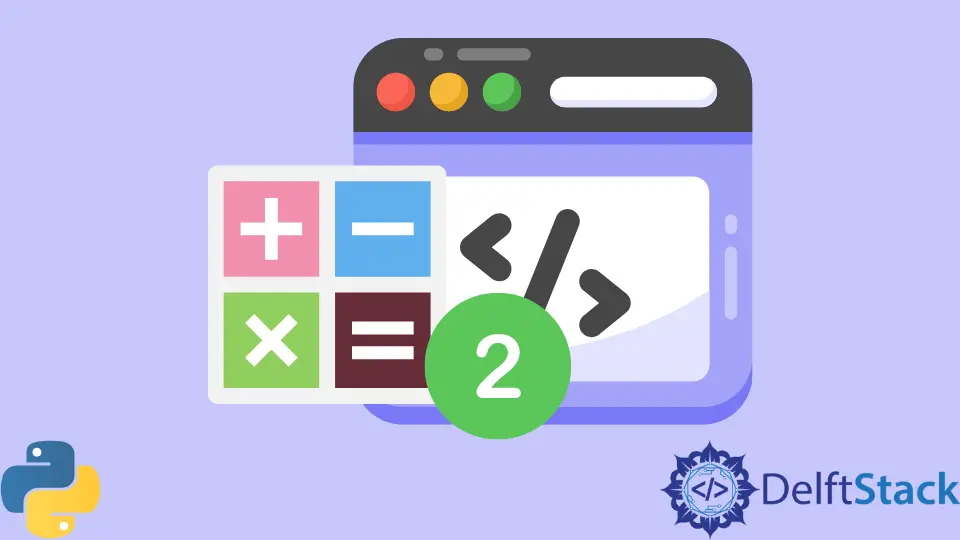
Python math.atan2()
method is an efficient way of calculating the arctangent of a number, x
, in radians. The value returned represents the angle theta
of a point (x
, y
) and the positive x-axis
.
Syntax
math.atan2(y, x)
Parameters
y |
Any positive or negative value. |
x |
Any positive or negative value. |
Return
The return type for this method is a float
value representing the arctangent of a theta
in radians. The value returned is in the range -PI
and PI.
Examples
Demonstrate the Correct Use of Python math.atan2()
Method
Code Snippet One:
import math
y = 0.334
x = -0.9
value = math.atan2(y, x)
print(f"The arctangent of {y},{x} is {value}.")
y = 100.0
x = 1
value = math.atan2(y, x)
print(f"The arctangent of {y},{x} is {value}.")
y = -0.03
x = 1087
value = math.atan2(y, x)
print(f"The arctangent of {y},{x} is {value}.")
Output:
The arctangent of 0.334,-0.9 is 2.7862357715696127.
The arctangent of 100.0,1 is 1.5607966601082315.
The arctangent of -0.03,1087 is -2.7598896037150882e-05.
Note that the parameters should be float
values. The values may be either positive or negative.
Code Snippet Two:
import math
y = [2, 4, 6]
x = [1, -4, 100]
for i in range(len(x)):
value = math.atan2(y[i], x[i])
print(f"The arctangent of {y},{x} is {value}.")
Output:
The arctangent of [2, 4, 6],[1, -4, 100] is 1.1071487177940904.
The arctangent of [2, 4, 6],[1, -4, 100] is 2.356194490192345.
The arctangent of [2, 4, 6],[1, -4, 100] is 0.05992815512120789.
Note that we can use this method to find the slope in radians when y
and x
coordinates are given.
Demonstrate an Error While Using the math.atan2()
Method
Code Snippet:
import math
y = 0
x = 1
value = math.atan2([y], [x])
print(f"The arctangent of {y},{x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.atan2([y],[x])
TypeError: must be the real number, not a list
Note that a TypeError
exception may occur if the argument value is not a float
.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn