Python math.acosh() Method
-
Syntax of Python
math.acosh()
Method -
Example Codes: Use Python
math.acosh()
to Compute Inverse Hyperbolic Cosine of a Value -
Example Codes: Use Python
math.acosh()
to Compute Arccosine and Cosine
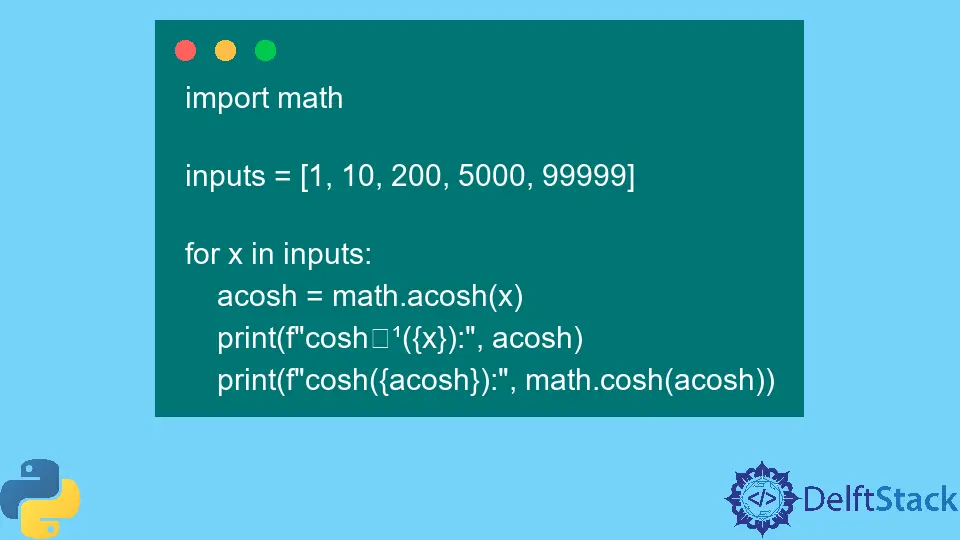
Python has a built-in library named math
for performing many mathematical operations. Fortunately, this library also supports trigonometric and inverse trigonometric operations.
The inverse of a hyperbolic cosine is known as inverse hyperbolic cosine or cosh⁻¹θ
. Its domain is the closed interval [1, +∞)
.
arcosh(θ) = ln(θ + √(θ² - 1))
This article will discuss the acosh()
method from the math module that helps compute inverse hyperbolic cosine for angles.
Syntax of Python math.acosh()
Method
math.acosh(x)
Parameters
Type | Explanation | |
---|---|---|
x |
Integer | A value between 1 and ∞, 1 inclusive. |
Returns
The acosh()
method returns the inverse hyperbolic cosine or cosh⁻¹
of x
in radians. The result is between the range 0
and ∞
, including 0
.
Example Codes: Use Python math.acosh()
to Compute Inverse Hyperbolic Cosine of a Value
import math
print(f"cosh⁻¹({1}) : {math.acosh(1)} radians")
print(f"cosh⁻¹({10}) : {math.acosh(10)} radians")
print(f"cosh⁻¹({200}) : {math.acosh(200)} radians")
print(f"cosh⁻¹({5000}) : {math.acosh(5000)} radians")
print(f"cosh⁻¹({99999}) : {math.acosh(99999)} radians")
Output:
cosh⁻¹(1) : 0.0 radians
cosh⁻¹(10) : 2.993222846126381 radians
cosh⁻¹(200) : 5.9914582970493875 radians
cosh⁻¹(5000) : 9.210340361976183 radians
cosh⁻¹(99999) : 12.206062645455173 radians
The Python code above computes the inverse of cosine or arccosine for 1
, 10
, 200
, 5000
, and 99999
. Note that all the results are in the range [0, ∞)
.
Example Codes: Use Python math.acosh()
to Compute Arccosine and Cosine
import math
inputs = [1, 10, 200, 5000, 99999]
for x in inputs:
acosh = math.acosh(x)
print(f"cosh⁻¹({x}):", acosh)
print(f"cosh({acosh}):", math.cosh(acosh))
Output:
cosh⁻¹(1): 0.0
cosh(0.0): 1.0
cosh⁻¹(10): 2.993222846126381
cosh(2.993222846126381): 9.999999999999998
cosh⁻¹(200): 5.9914582970493875
cosh(5.9914582970493875): 200.0
cosh⁻¹(5000): 9.210340361976183
cosh(9.210340361976183): 5000.000000000001
cosh⁻¹(99999): 12.206062645455173
cosh(12.206062645455173): 99999.00000000001
The Python code above calculates inverse hyperbolic cosine of some value x
and uses its result to re-compute x
using hyperbolic cosine. It performs the above operation for 1
, 10
, 200
, 5000
, and 99999
.