Python datetime.date.date.replace() Method
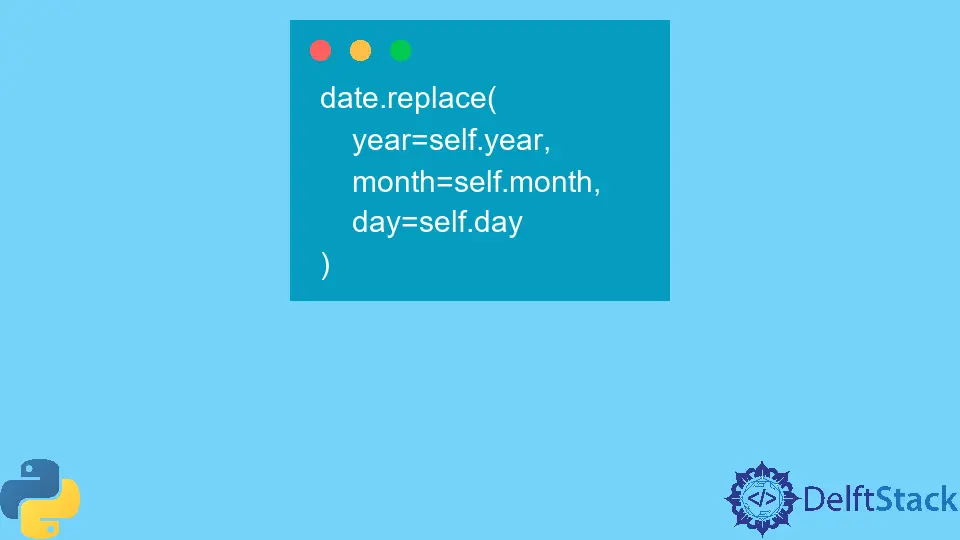
Python programming language is a dynamically-typed general-purpose language that offers a fine development experience. This language has huge community support, and one can find numerous third-party modules to work with.
This language provides a built-in module, datetime
, to work with date and time. The datetime
module is a robust module with many features.
It offers various methods and classes to perform dates and times-related computations and represent associated quantities.
The datetime
module contains a class date
that can represent a date. This class has several methods and attributes to create date
objects, manipulate existing objects, etc.
One such method is the replace()
method, which lets us edit an existing date
object and create a new object with those values. In this article, we will talk about this method in detail with some relevant examples.
Syntax
date.replace(year=self.year, month=self.month, day=self.day)
Parameters
Parameters | Type | Description |
---|---|---|
year |
Integer | A year value. |
month |
Integer | A month value between 1 and 12, inclusive. |
day |
Integer | A day value between 1 and 31, inclusive. |
Returns
The replace()
method returns a new date
object with all the same attributes as the original object, except for those attributes for which new values were provided.
Example Code: Use the datetime.date.date.replace()
Method in Python
import datetime
d = datetime.date(2022, 8, 25)
print("Date:", d)
print("Changed Date:", d.replace(year=2001, day=20))
Output:
Date: 2022-08-25
Changed Date: 2001-08-20
The Python code above first creates a date
object using the datetime.date
constructor.
Next, it modifies the date values of the date
object using the instance method replace()
. The print()
method prints the new date
object generated.