Python datetime.astimezone() Method
-
Syntax of Python
datetime.astimezone()
Method -
Example 1: Use
astimezone
Withtz
Module -
Example 2: Use
astimezone
Withdatetime.timezone
-
Example 3: Use
astimezone
Withpytz
Module -
TypeError: can't compare offset-naive and offset-aware datetimes
Encounter in Usingdatetime.astimezone()
Method - Conclusion
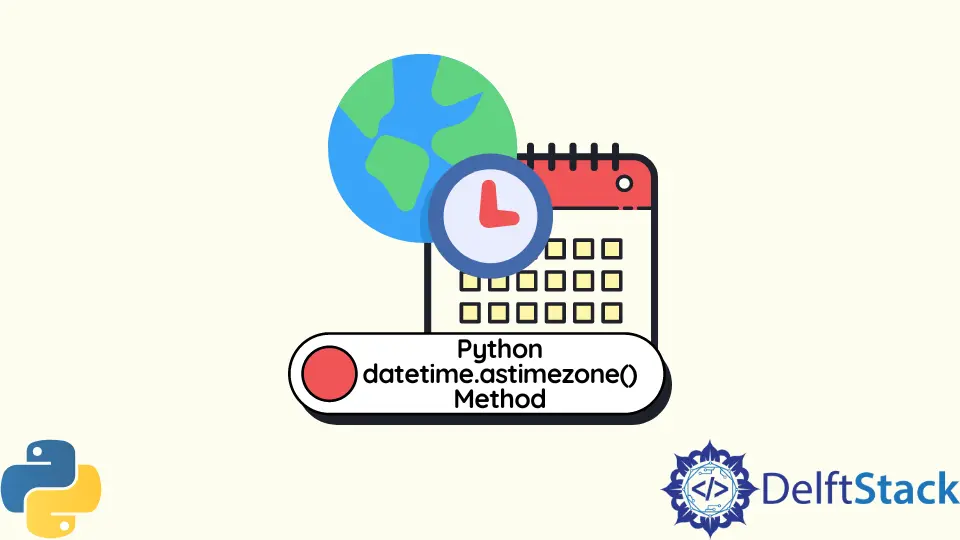
In programming, dealing with time zones is a common necessity, especially when working with datetime
objects.
The datetime
module is a built-in module available in Python programming language. This library offers reliable and efficient functions to work with dates, times, time differences, and time zones and perform various calculations over them.
This module contains a class datetime
that stores date along with time and timezone. This class’s objects have access to different instance methods, such as astimezone()
, that return a new datetime
object with timezone information.
The datetime.astimezone
method converts a datetime
object from one time zone to another, providing flexibility and accuracy when handling time-related data.
This method is particularly useful when working with applications that involve scheduling, logging, or any time-sensitive operations across different time zones.
Syntax of Python datetime.astimezone()
Method
<datetime object>.astimezone()
Parameters
Type | Description | |
---|---|---|
tz |
tzinfo |
A tzinfo object representing a valid timezone. The default value is None . |
The tzinfo
is an abstract base class that stores details about a timezone. Since it is an abstract class, it cannot be instantiated, and some concrete class has to instantiate the tzinfo
class and implement its four important methods: utcoffset(dt)
, dst(dt)
, tzname(dt)
, and fromutc(dt)
.
In this article, we will not perform the actual implementation of the concrete class. Instead, we will use the tz
module that has already subclassed the abstract tzinfo
class and the timezone
class of the datetime
module.
To learn more about the tzinfo
, its methods, and how it should be inherited and implemented, refer to the official Python documentation here. To learn more about the tz
module in-depth, refer to the official documentation here.
Returns
This method returns a new datetime
object with a new tz
attribute of type tzinfo
. The date and time values are adjusted to the local time related to the timezone.
By default, the datetime
object stores the date and time according to UTC or Coordinated Universal Time. In real-world applications, date and time are stored in UTC and not hard-coded in the server’s or user’s timezone.
Depending upon the user’s location and timezone, date and time values are adjusted and presented to the user. And it’s very straightforward to convert date and time from one timezone to another.
Example 1: Use astimezone
With tz
Module
The code snippet below demonstrates the usage of datetime.astimezone
by converting the current datetime
to multiple time zones.
Example code:
from datetime import datetime
from dateutil import tz
# Get the current datetime
dt = datetime.now()
# Define time zone objects for different regions
t1 = tz.gettz("America/Los_Angeles")
t2 = tz.gettz("Asia/Kolkata")
t3 = tz.gettz("Asia/Singapore")
t4 = tz.gettz("Europe/Paris")
t5 = tz.gettz("Canada/Pacific")
# Format datetime objects before printing
format_str = "%Y-%m-%d %H:%M:%S%z"
# Display datetime in various time zones
print("UTC:", dt.strftime(format_str))
print("America/Los_Angeles:", dt.astimezone(t1).strftime(format_str))
print("Asia/Kolkata:", dt.astimezone(t2).strftime(format_str))
print("Asia/Singapore:", dt.astimezone(t3).strftime(format_str))
print("Europe/Paris:", dt.astimezone(t4).strftime(format_str))
print("Canada/Pacific:", dt.astimezone(t5).strftime(format_str))
The Python code above first creates a datetime
object for the current time. Next, it creates 5 objects representing the timezones, namely, America/Los_Angeles
, Asia/Kolkata
, Asia/Singapore
, Europe/Paris
, and Canada/Pacific
.
All these objects are of type <class 'dateutil.zoneinfo.tzfile'>
. One can verify that using Python’s built-in type()
method.
The tzfile
is the concrete class that inherits the abstract class tzinfo
and implements all the crucial methods. Next, using the astimezone()
method, 5 timezone-aware datetime
objects are created.
Let’s compare all the new 5 datetime
objects with the basic datetime
object (UTC timezone). We will find that for every timezone-aware object, the date and time are adjusted to the timezone’s local time.
Depending on the timezone sign, we can easily verify that by adding or subtracting time from the UTC time.
Output:
This output demonstrates how the original datetime
is converted to the specified time zones, accurately reflecting the respective local times.
By utilizing datetime.astimezone
, developers can ensure consistency and precision when handling datetime
objects across different regions with distinct time zones.
Example 2: Use astimezone
With datetime.timezone
In this example, we’ll delve into how to utilize datetime.astimezone
to convert a datetime
object to various time zones, providing insights into different regions’ local times.
Example code:
from datetime import datetime, timezone, timedelta
# Get the current datetime
dt = datetime.now()
# Define time zone objects for different regions
t1 = timezone(timedelta(hours=-7, minutes=0), "America/Los_Angeles")
t2 = timezone(timedelta(hours=5, minutes=30), "Asia/Kolkata")
t3 = timezone(timedelta(hours=8, minutes=0), "Asia/Singapore")
t4 = timezone(timedelta(hours=2, minutes=0), "Europe/Paris")
t5 = timezone(timedelta(hours=7, minutes=0), "Canada/Pacific")
# Display datetime in various time zones
print("UTC:", dt)
print("America/Los_Angeles:", dt.astimezone(t1))
print("Asia/Kolkata:", dt.astimezone(t2))
print("Asia/Singapore:", dt.astimezone(t3))
print("Europe/Paris:", dt.astimezone(t4))
print("Canada/Pacific:", dt.astimezone(t5))
In the Python code snippet above, we import necessary modules, including datetime
for handling datetime
objects, timezone
for representing time zones, and timedelta
for specifying time differences.
The current datetime
is retrieved using datetime.now()
and stored in the variable dt
. Time zone objects for various regions are created using the timezone constructor, wherein we specify the time zone offsets from UTC along with the time zone names as strings, such as "America/Los_Angeles"
and "Asia/Kolkata"
.
Subsequently, the astimezone()
method is employed to convert the original datetime
object dt
to the time zones represented by the predefined time zone objects t1
, t2
, t3
, t4
, and t5
.
Finally, each converted datetime
object is printed along with its corresponding time zone label, showcasing the effective handling of time zone conversions within the Python script.
Output:
The datetime.timezone
class represents a timezone differently. It uses datetime.timedelta
to represent the offset.
The output demonstrates how the original datetime
is converted to different time zones accurately, reflecting the respective local times with their corresponding offsets from UTC.
This showcases the effectiveness of datetime.astimezone
in handling time zone conversions within Python applications.
Apart from that, everything remains the same as in the previous example.
Example 3: Use astimezone
With pytz
Module
In this example, we’ll explore how to use astimezone
to convert a datetime
object from one time zone to another using the pytz
library.
Example code:
from datetime import datetime, timezone
from pytz import timezone as pytz_timezone
# Create a datetime object representing a specific time (in UTC)
dt_utc = datetime(2024, 5, 15, 10, 30, tzinfo=timezone.utc)
# Convert to a different time zone using pytz
tz_target = pytz_timezone("America/New_York") # Define target time zone
# Convert datetime to the target time zone
dt_target = dt_utc.astimezone(tz_target)
# Display the converted datetime object
print("Original datetime (UTC):", dt_utc)
print("Converted datetime (America/New_York):", dt_target)
The code starts by creating a datetime
object dt_utc
representing a specific time in Coordinated Universal Time (UTC).
Then, a target time zone is defined using pytz.timezone
from the pytz
library, with 'America/New_York'
specified as the desired time zone.
Subsequently, the astimezone
method is employed to convert dt_utc
to the target time zone, resulting in the datetime
object dt_target
.
Lastly, both the original datetime
object in UTC and the converted datetime
object in the 'America/New_York'
time zone are printed to observe the difference in time zones.
Output:
TypeError: can't compare offset-naive and offset-aware datetimes
Encounter in Using datetime.astimezone()
Method
This example explores a scenario where an error occurs due to comparing a naive datetime
object with a timezone-aware datetime
object using the astimezone
method.
Example code:
from datetime import datetime, timezone, timedelta
# Create a naive datetime object
dt_naive = datetime(2024, 5, 15, 10, 30)
# Define the time zone for America/Los_Angeles
t1 = timezone(timedelta(hours=-7, minutes=0), "America/Los_Angeles")
# Convert the naive datetime to a timezone-aware datetime
dt_aware = dt_naive.astimezone(t1)
# Attempt to compare the naive datetime with the timezone-aware datetime
result = dt_naive > dt_aware
# Display the result
print("Result of comparison:", result)
The code showcases a scenario where an error arises from comparing a naive datetime
object with a timezone-aware datetime
object using the astimezone
method.
Initially, a naive datetime
object dt_naive
representing a specific date and time is created.
Subsequently, a timezone object t1
for 'America/Los_Angeles'
with an offset of -7 hours from UTC is defined. The naive datetime
object dt_naive
is then converted to a timezone-aware datetime
object dt_aware
using the astimezone
method with the specified time zone t1
.
An attempt is made to compare dt_naive
(a naive datetime
object) with dt_aware
(a timezone-aware datetime
object) using the >
operator.
However, this comparison results in a TypeError: can't compare offset-naive and offset-aware datetimes
, indicating that directly comparing naive and timezone-aware datetime
objects is not supported in Python.
Output:
Traceback (most recent call last):
File "C:/Users/Admin/Desktop/main.py", line 13, in <module>
result = dt_naive > dt_aware
TypeError: can't compare offset-naive and offset-aware datetimes
This example underscores the significance of ensuring that datetime
objects are of the same type (either naive or timezone-aware) before performing comparisons to prevent such errors.
Conclusion
In programming, handling time zones is essential, especially when dealing with datetime
objects.
Python’s datetime
module offers robust functionality for managing dates, times, time differences, and time zones efficiently. By utilizing the astimezone()
method, developers can seamlessly convert datetime
objects between different time zones, ensuring accuracy and consistency in time-related operations.
However, it’s crucial to handle datetime
objects consistently; attempting to compare naive datetime
objects with timezone-aware datetime
objects directly can lead to errors.
This demonstration highlights the importance of maintaining datetime
object types and handling time zone conversions appropriately to ensure error-free execution in Python programs.