Pandas DataFrame DataFrame.transpose() Function
-
Syntax of
pandas.DataFrame.transpose()
-
Example Codes:
DataFrame.transpose()
-
Example Codes:
DataFrame.transpose()
to TransposeDataFrame
With Homogeneous Data Types -
Example Codes:
DataFrame.transpose()
to TransposeDataFrame
With Mixed Data Types
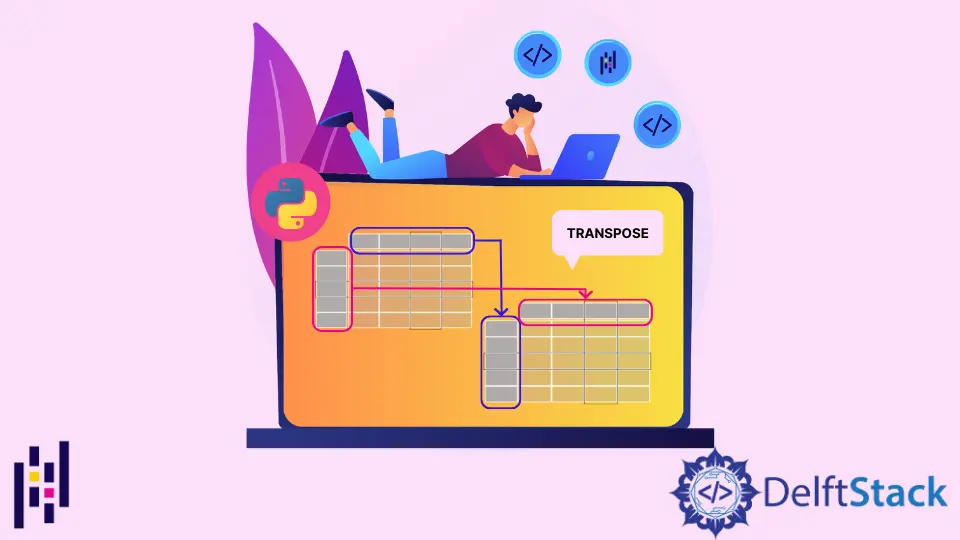
Python Pandas DataFrame.transpose()
function changes the rows of the DataFrame
to columns, and columns to rows. In other words, it generates a new DataFrame
which is the transpose of the original DataFrame
.
Syntax of pandas.DataFrame.transpose()
DataFrame.transpose(*args, copy=False)
Parameters
*args |
These are the additional keyword arguments for compatibility with NumPy . |
copy |
It is a boolean value. It decides whether the values of the DataFrame will be copied or not after taking the transpose. By default, its value is False . |
Return
It returns a transposed DataFrame
. The rows of the original DataFrame
are columns in the returned DataFrame
and vice versa.
Example Codes: DataFrame.transpose()
We will implement this function in the next few codes.
import pandas as pd
dataframe=pd.DataFrame({
'Attendance':
{0: 60,
1: 100,
2: 80,
3: 78,
4: 95},
'Name':
{0: 'Olivia',
1: 'John',
2: 'Laura',
3: 'Ben',
4: 'Kevin'},
'Obtained Marks':
{0: 90,
1: 75,
2: 82,
3: 64,
4: 45}
})
print(dataframe)
The example DataFrame
is,
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
All the parameters of this function are optional. If we execute this function without passing any parameter then it produces the following output.
import pandas as pd
dataframe=pd.DataFrame({
'Attendance':
{0: 60,
1: 100,
2: 80,
3: 78,
4: 95},
'Name':
{0: 'Olivia',
1: 'John',
2: 'Laura',
3: 'Ben',
4: 'Kevin'},
'Obtained Marks':
{0: 90,
1: 75,
2: 82,
3: 64,
4: 45}
})
dataframe1 = dataframe.transpose()
print(dataframe1)
Output:
0 1 2 3 4
Attendance 60 100 80 78 95
Name Olivia John Laura Ben Kevin
Obtained Marks 90 75 82 64 45
Example Codes: DataFrame.transpose()
to Transpose DataFrame
With Homogeneous Data Types
The behavior of this function is different for homogeneous and mixed data types. We will analyze it one by one. If we have a homogeneous type DataFrame
then the data types of the original and the transposed Dataframes
are the same.
The DataFrame
with the homogeneous data types is as follows
import pandas as pd
dataframe = pd.DataFrame(
{"A": {0: 6, 1: 20, 2: 80, 3: 78, 4: 95}, "B": {0: 60, 1: 50, 2: 7, 3: 67, 4: 54}}
)
print(dataframe)
Our DataFrame
is,
A B
0 6 60
1 20 50
2 80 7
3 78 67
4 95 54
5 98 34
To get the transpose of this DataFrame
,
import pandas as pd
dataframe = pd.DataFrame(
{"A": {0: 6, 1: 20, 2: 80, 3: 78, 4: 95}, "B": {0: 60, 1: 50, 2: 7, 3: 67, 4: 54}}
)
dataframe1 = dataframe.transpose()
print(dataframe1)
Output:
0 1 2 3 4
A 6 20 80 78 95
B 60 50 7 67 54
Now, let’s analyze the data types of the original DataFrame
and the returned DataFrame
.
import pandas as pd
dataframe = pd.DataFrame(
{"A": {0: 6, 1: 20, 2: 80, 3: 78, 4: 95}, "B": {0: 60, 1: 50, 2: 7, 3: 67, 4: 54}}
)
dataframe1 = dataframe.transpose()
print(dataframe.dtypes)
print(dataframe1.dtypes)
Output:
A int64
B int64
dtype: object
0 int64
1 int64
2 int64
3 int64
4 int64
dtype: object
Note that the data types of the original and the transposed DataFrames
are the same.
Example Codes: DataFrame.transpose()
to Transpose DataFrame
With Mixed Data Types
If we have a mixed type DataFrame
then the data types of the original and the transposed Dataframes
are different. The transposed DataFrame
has object data types. The DataFrame
with the mixed data types is as follows
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45},
}
)
print(dataframe)
Our DataFrame
is,
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
To get the transpose of this DataFrame
,
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45},
}
)
dataframe1 = dataframe.transpose()
print(dataframe1)
Output:
0 1 2 3 4
Attendance 60 100 80 78 95
Name Olivia John Laura Ben Kevin
Obtained Marks 90 75 82 64 45
Now, let’s analyze the data types of the original DataFrame
and the returned DataFrame
.
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 78, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 90, 1: 75, 2: 82, 3: 64, 4: 45},
}
)
dataframe1 = dataframe.transpose()
print(dataframe.dtypes)
print(dataframe1.dtypes)
Output:
Attendance int64
Name object
Obtained Marks int64
dtype: object
0 object
1 object
2 object
3 object
4 object
dtype: object
Note that the data types of the transposed DataFrame
are of the object
data type.