Pandas DataFrame.to_numeric() Function
Minahil Noor
Jan 30, 2023
Pandas
Pandas DataFrame
-
Syntax of
pandas.DataFrame.to_numeric()
: -
Example Codes:
DataFrame.to_numeric()
Method to Convert a Series to Numeric -
Example Codes:
DataFrame.to_numeric()
Method to Convert a Series to Integer
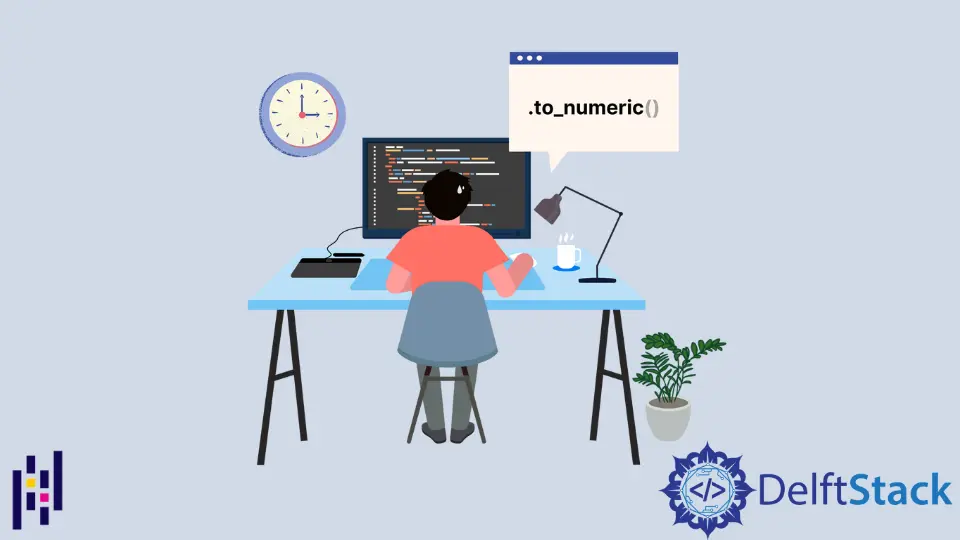
Python Pandas DataFrame.to_numeric()
function converts the passed argument to a numeric type.
Syntax of pandas.DataFrame.to_numeric()
:
DataFrame.to_numeric(arg, errors="raise", downcast=None)
Parameters
arg |
It is a scalar, list, tuple, 1-d array, or Series . It is the argument that we want to convert to numeric. |
errors |
It is a string parameter. It has three options: ignore , raise , or coerce . If it is set to raise , then an invalid argument will raise an exception. If it is set to coerce , then an invalid argument will be set as NaN. If it is set to ignore , then an invalid argument will return the input. |
downcast |
It is a string parameter. It has four options: integer , signed , unsigned , or float . |
Return
It returns a numeric if parsing is successful. If a series is passed, then it will return a series; otherwise, it will return ndarray
.
Example Codes: DataFrame.to_numeric()
Method to Convert a Series to Numeric
import pandas as pd
series = pd.Series(['1.0', '2', '-3', '4', '5.5', '6.7'])
print("The Original Series is: \n")
print(series)
series1 = pd.to_numeric(series)
print("The Numeric Series is: \n")
print(series1)
Output:
The Original Series is:
0 1.0
1 2
2 -3
3 4
4 5.5
5 6.7
dtype: object
The Numeric Series is:
0 1.0
1 2.0
2 -3.0
3 4.0
4 5.5
5 6.7
dtype: float64
The function has returned the numeric series.
Example Codes: DataFrame.to_numeric()
Method to Convert a Series to Integer
import pandas as pd
series = pd.Series(['1.0', '2', '-3', '4', '5', '6'])
print("The Original Series is: \n")
print(series)
series1 = pd.to_numeric(series, downcast='signed')
print("The Numeric Series is: \n")
print(series1)
Output:
The Original Series is:
0 1.0
1 2
2 -3
3 4
4 5
5 6
dtype: object
The Numeric Series is:
0 1
1 2
2 -3
3 4
4 5
5 6
dtype: int8
The function has returned the int8 type series.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe