Pandas concat Function
-
pandas.concat()
Syntax -
Example: Concatenate 2 Pandas Series Along Row-Axis Using the
pandas.concat()
Method -
Example: Concatenate 2 Pandas Series Objects Along Column-Axis Using the
pandas.concat()
Method -
Example: Concatenate 2 Pandas DataFrame Objects Using the
pandas.concat()
Method -
Example: Concatenate DataFrame With a Series Object Using the
pandas.concat()
Method
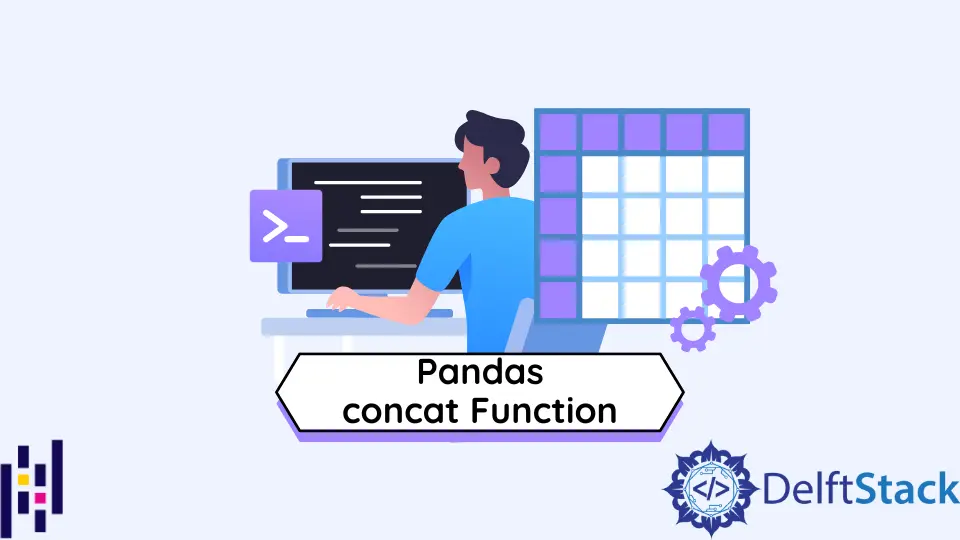
pandas.concat()
method concatenates Pandas DataFrame or Series objects.
pandas.concat()
Syntax
pandas.concat(
objs,
axis=0,
join="outer",
ignore_index=False,
keys=None,
levels=None,
names=None,
verify_integrity=False,
sort=False,
copy=True,
)
Parameters
objs |
sequence or mapping of Pandas Series or DataFrame objects to be concatenated. |
join |
Method of join(inner or outer ) |
axis |
concat along the row (axis=0 ) or column (axis=1 ) |
ignore_index |
Boolean. If True , the indexes from the original DataFrames is ignored. |
keys |
sequence to add an identifier to the result indexes |
levels |
levels to use for creating a MultiIndex |
names |
names for levels in MultiIndex |
verify_integrity |
Boolean. If True , check for duplicates |
sort |
Boolean. Sort non-concatenation axis if it is not already aligned when join is outer . |
copy |
Boolean. If False , avoid unnecessary copying of data |
Return
It returns a Series
object if all the Series
objects are concatenated along the axis=0
. It returns a DataFrame
object if any object to be concatenated is a DataFrame
, or the Series
objects are concatenated along the axis=1
.
Example: Concatenate 2 Pandas Series Along Row-Axis Using the pandas.concat()
Method
import pandas as pd
ser_1 = pd.Series([20, 45, 36, 45])
print("Series-1:")
print(ser_1, "\n")
ser_2 = pd.Series([48, 46, 34, 38])
print("Series-2:")
print(ser_2, "\n")
concatenated_ser = pd.concat([ser_1, ser_2])
print("Result after Concatenation of ser_1 and ser_2:")
print(concatenated_ser)
Output:
Series-1:
0 20
1 45
2 36
3 45
dtype: int64
Series-2:
0 48
1 46
2 34
3 38
dtype: int64
Result after Concatenation of ser_1 and ser_2:
0 20
1 45
2 36
3 45
0 48
1 46
2 34
3 38
dtype: int64
It concatenates the Series
objects ser_1
and ser_2
along axis=0
or row-wise. The rows of one of the Series
objects are stacked on top of the other. The concatenated object will take index
values from the parent objects by default. We can set ignore_index=True
to assign new index values to the concatenated object.
import pandas as pd
ser_1 = pd.Series([20, 45, 36, 45])
print("Series-1:")
print(ser_1, "\n")
ser_2 = pd.Series([48, 46, 34, 38])
print("Series-2:")
print(ser_2, "\n")
concatenated_ser = pd.concat([ser_1, ser_2], ignore_index=True)
print("Result after Concatenation of ser_1 and ser_2:")
print(concatenated_ser)
Output:
Series-1:
0 20
1 45
2 36
3 45
dtype: int64
Series-2:
0 48
1 46
2 34
3 38
dtype: int64
Result after Concatenation of ser_1 and ser_2:
0 20
1 45
2 36
3 45
4 48
5 46
6 34
7 38
dtype: int64
It concatenates the Series
objects and assigns new index values to the concatenated Series
object.
Example: Concatenate 2 Pandas Series Objects Along Column-Axis Using the pandas.concat()
Method
We set axis=1
in the pandas.concat()
method to concatenate Series
objects horizontally or along column-axis.
import pandas as pd
ser_1 = pd.Series([20, 45, 36, 45])
print("Series-1:")
print(ser_1, "\n")
ser_2 = pd.Series([48, 46, 34, 38])
print("Series-2:")
print(ser_2, "\n")
concatenated_ser = pd.concat([ser_1, ser_2], axis=1)
print("Result after Horizontal Concatenation of ser_1 and ser_2:")
print(concatenated_ser)
Output:
Series-1:
0 20
1 45
2 36
3 45
dtype: int64
Series-2:
0 48
1 46
2 34
3 38
dtype: int64
Result after Horizontal Concatenation of ser_1 and ser_2:
0 1
0 20 48
1 45 46
2 36 34
3 45 38
It horizontally stacks the Series
objects ser_1
and ser_2
.
Example: Concatenate 2 Pandas DataFrame Objects Using the pandas.concat()
Method
import pandas as pd
df_1 = pd.DataFrame({"Col-1": [1, 2, 3, 4], "Col-2": [5, 6, 7, 8]})
print("DataFrame-1:")
print(df_1, "\n")
df_2 = pd.DataFrame({"Col-1": [10, 20, 30, 40], "Col-2": [50, 60, 70, 80]})
print("DataFrame-2:")
print(df_2, "\n")
concatenated_df = pd.concat([df_1, df_2], ignore_index=True)
print("Result after Horizontal Concatenation of df_1 and df_2:")
print(concatenated_df)
Output:
DataFrame-1:
Col-1 Col-2
0 1 5
1 2 6
2 3 7
3 4 8
DataFrame-2:
Col-1 Col-2
0 10 50
1 20 60
2 30 70
3 40 80
Result after Horizontal Concatenation of df_1 and df_2:
Col-1 Col-2
0 1 5
1 2 6
2 3 7
3 4 8
4 10 50
5 20 60
6 30 70
7 40 80
It concatenates the DataFrame
objects df_1
and df_2
. By setting ignore_index=True
, we assign new indices to the concatenated DataFrame.
Example: Concatenate DataFrame With a Series Object Using the pandas.concat()
Method
import pandas as pd
df = pd.DataFrame({"Col-1": [1, 2, 3, 4], "Col-2": [5, 6, 7, 8]})
print("DataFrame Object:")
print(df, "\n")
ser = pd.Series([48, 46, 34, 38])
print("Series Object:")
print(ser, "\n")
ser_df = pd.concat([df, ser], axis=1)
print("Concatenation of ser and df:")
print(ser_df)
Output:
DataFrame Object:
Col-1 Col-2
0 1 5
1 2 6
2 3 7
3 4 8
Series Object:
0 48
1 46
2 34
3 38
dtype: int64
Concatenation of ser and df:
Col-1 Col-2 0
0 1 5 48
1 2 6 46
2 3 7 34
3 4 8 38
It concatenates the DataFrame object df
and the Series
object ser
together. The concatenation is done column-wise as we have set axis=1
in the pandas.concat()
method.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn