Python numpy.unique() Function
-
Syntax of
numpy.unique()
: -
Example Codes:
numpy.unique()
Method -
Example Codes: Set
return_index=True
innumpy.unique()
Method -
Example Codes: Set
return_counts=True
innumpy.unique()
Method -
Example Codes: Set
return_inverse=True
innumpy.unique()
Method -
Example Codes: Set
axis
Parameter in thenumpy.unique()
Method
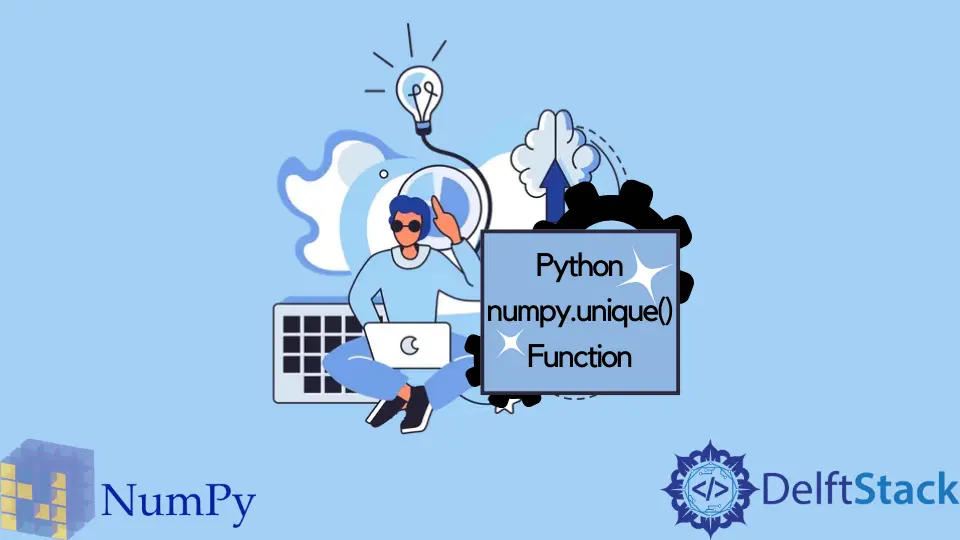
Python Numpy numpy.unique()
function retrieves all the unique values in the given NumPy
array and sorts these unique values.
Syntax of numpy.unique()
:
numpy.unique(
ar, return_index=False, return_inverse=False, return_counts=False, axis=None
)
Parameters
ar |
Array or Object which could be converted into an array |
return_index |
Boolean. If True, return an array of indices of the first occurrence of each unique value. |
return_inverse |
Boolean. If True, return the indices of a unique array, which can be used to reconstruct the input array. |
return_counts |
Boolean. If True, return an array of the count of each unique value. |
axis |
find unique rows (axis=0) or columns (axis=1). By default, unique elements are retrieved from the flattened array. |
Return
It returns sorted unique values of the array.
If return_index=True
, it returns an array of indices of the first occurrence of each unique value.
If return_counts=True
, it returns an array of the count of each unique value in the input array.
If return_inverse=True
, it returns the indices of a unique array, which can be used to reconstruct the input array.
Example Codes: numpy.unique()
Method
import numpy as np
a=np.array([[2,3,4],
[5,4,7],
[4,2,3]])
unique_array=np.unique(a)
print(unique_array)
Output:
[2 3 4 5 7]
It returns sorted unique values of the flattened input array.
By flattening the array, we mean placing all the rows one after another to convert the given array to a 1-D array.
Example Codes: Set return_index=True
in numpy.unique()
Method
import numpy as np
a=np.array([[2,3,4],
[5,4,7],
[4,2,3]])
unique_array=np.unique(a,return_index=True)
print(unique_array)
Output:
(array([2, 3, 4, 5, 7]), array([0, 1, 2, 3, 5]))
It gives a tuple of an array of sorted unique values in the given flattened input array and an array of indices of the first occurrence of each unique value.
Example Codes: Set return_counts=True
in numpy.unique()
Method
import numpy as np
a=np.array([[2,3,4],
[5,4,7],
[4,2,3]])
unique_array=np.unique(a,return_counts=True)
print(unique_array)
Output:
(array([2, 3, 4, 5, 7]), array([2, 2, 3, 1, 1]))
It gives a tuple of an array of sorted unique values in the given flattened input array and an array of the count of each unique value the input array.
Example Codes: Set return_inverse=True
in numpy.unique()
Method
import numpy as np
a=np.array([[2,3,4],
[5,4,7],
[4,2,3]])
unique_array=np.unique(a,return_inverse=True)
print(unique_array)
Output:
(array([2, 3, 4, 5, 7]), array([0, 1, 2, 3, 2, 4, 2, 0, 1]))
It gives a tuple of an array of sorted unique values in the given flattened input array and an array of the indices of a unique array.
Here, 2
occurs at the first position and the second last position of the flattened array. Similarly, we can find which value occurs at which position.
Example Codes: Set axis
Parameter in the numpy.unique()
Method
Find Unique Rows
import numpy as np
a=np.array([[2,3,2],
[2,3,2],
[4,2,3]])
unique_array=np.unique(a,axis=0)
print(unique_array)
Output:
[[2 3 2]
[4 2 3]]
It gives all the unique rows in the input array.
Find Unique Columns
import numpy as np
a=np.array([[2,3,2],
[2,3,2],
[3,2,3]])
unique_array=np.unique(a,axis=1)
print(unique_array)
Output:
[[2 3]
[2 3]
[3 2]]
It gives all the unique columns in the input array.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn